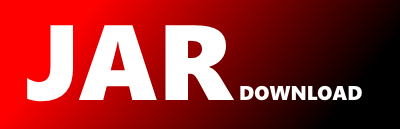
com.pulumi.azurenative.network.inputs.FrontDoorMatchConditionArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.inputs;
import com.pulumi.azurenative.network.enums.FrontDoorMatchVariable;
import com.pulumi.azurenative.network.enums.Operator;
import com.pulumi.azurenative.network.enums.TransformType;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Define a match condition.
*
*/
public final class FrontDoorMatchConditionArgs extends com.pulumi.resources.ResourceArgs {
public static final FrontDoorMatchConditionArgs Empty = new FrontDoorMatchConditionArgs();
/**
* List of possible match values.
*
*/
@Import(name="matchValue", required=true)
private Output> matchValue;
/**
* @return List of possible match values.
*
*/
public Output> matchValue() {
return this.matchValue;
}
/**
* Request variable to compare with.
*
*/
@Import(name="matchVariable", required=true)
private Output> matchVariable;
/**
* @return Request variable to compare with.
*
*/
public Output> matchVariable() {
return this.matchVariable;
}
/**
* Describes if the result of this condition should be negated.
*
*/
@Import(name="negateCondition")
private @Nullable Output negateCondition;
/**
* @return Describes if the result of this condition should be negated.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy