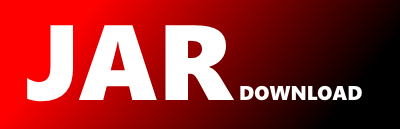
com.pulumi.azurenative.network.outputs.GetExpressRouteCircuitPeeringResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.ExpressRouteCircuitConnectionResponse;
import com.pulumi.azurenative.network.outputs.ExpressRouteCircuitPeeringConfigResponse;
import com.pulumi.azurenative.network.outputs.ExpressRouteCircuitStatsResponse;
import com.pulumi.azurenative.network.outputs.ExpressRouteConnectionIdResponse;
import com.pulumi.azurenative.network.outputs.Ipv6ExpressRouteCircuitPeeringConfigResponse;
import com.pulumi.azurenative.network.outputs.PeerExpressRouteCircuitConnectionResponse;
import com.pulumi.azurenative.network.outputs.SubResourceResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetExpressRouteCircuitPeeringResult {
/**
* @return The Azure ASN.
*
*/
private @Nullable Integer azureASN;
/**
* @return The list of circuit connections associated with Azure Private Peering for this circuit.
*
*/
private @Nullable List connections;
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return The ExpressRoute connection.
*
*/
private @Nullable ExpressRouteConnectionIdResponse expressRouteConnection;
/**
* @return The GatewayManager Etag.
*
*/
private @Nullable String gatewayManagerEtag;
/**
* @return Resource ID.
*
*/
private @Nullable String id;
/**
* @return The IPv6 peering configuration.
*
*/
private @Nullable Ipv6ExpressRouteCircuitPeeringConfigResponse ipv6PeeringConfig;
/**
* @return Who was the last to modify the peering.
*
*/
private String lastModifiedBy;
/**
* @return The Microsoft peering configuration.
*
*/
private @Nullable ExpressRouteCircuitPeeringConfigResponse microsoftPeeringConfig;
/**
* @return The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
private @Nullable String name;
/**
* @return The peer ASN.
*
*/
private @Nullable Double peerASN;
/**
* @return The list of peered circuit connections associated with Azure Private Peering for this circuit.
*
*/
private List peeredConnections;
/**
* @return The peering type.
*
*/
private @Nullable String peeringType;
/**
* @return The primary port.
*
*/
private @Nullable String primaryAzurePort;
/**
* @return The primary address prefix.
*
*/
private @Nullable String primaryPeerAddressPrefix;
/**
* @return The provisioning state of the express route circuit peering resource.
*
*/
private String provisioningState;
/**
* @return The reference to the RouteFilter resource.
*
*/
private @Nullable SubResourceResponse routeFilter;
/**
* @return The secondary port.
*
*/
private @Nullable String secondaryAzurePort;
/**
* @return The secondary address prefix.
*
*/
private @Nullable String secondaryPeerAddressPrefix;
/**
* @return The shared key.
*
*/
private @Nullable String sharedKey;
/**
* @return The peering state.
*
*/
private @Nullable String state;
/**
* @return The peering stats of express route circuit.
*
*/
private @Nullable ExpressRouteCircuitStatsResponse stats;
/**
* @return Type of the resource.
*
*/
private String type;
/**
* @return The VLAN ID.
*
*/
private @Nullable Integer vlanId;
private GetExpressRouteCircuitPeeringResult() {}
/**
* @return The Azure ASN.
*
*/
public Optional azureASN() {
return Optional.ofNullable(this.azureASN);
}
/**
* @return The list of circuit connections associated with Azure Private Peering for this circuit.
*
*/
public List connections() {
return this.connections == null ? List.of() : this.connections;
}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return The ExpressRoute connection.
*
*/
public Optional expressRouteConnection() {
return Optional.ofNullable(this.expressRouteConnection);
}
/**
* @return The GatewayManager Etag.
*
*/
public Optional gatewayManagerEtag() {
return Optional.ofNullable(this.gatewayManagerEtag);
}
/**
* @return Resource ID.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return The IPv6 peering configuration.
*
*/
public Optional ipv6PeeringConfig() {
return Optional.ofNullable(this.ipv6PeeringConfig);
}
/**
* @return Who was the last to modify the peering.
*
*/
public String lastModifiedBy() {
return this.lastModifiedBy;
}
/**
* @return The Microsoft peering configuration.
*
*/
public Optional microsoftPeeringConfig() {
return Optional.ofNullable(this.microsoftPeeringConfig);
}
/**
* @return The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return The peer ASN.
*
*/
public Optional peerASN() {
return Optional.ofNullable(this.peerASN);
}
/**
* @return The list of peered circuit connections associated with Azure Private Peering for this circuit.
*
*/
public List peeredConnections() {
return this.peeredConnections;
}
/**
* @return The peering type.
*
*/
public Optional peeringType() {
return Optional.ofNullable(this.peeringType);
}
/**
* @return The primary port.
*
*/
public Optional primaryAzurePort() {
return Optional.ofNullable(this.primaryAzurePort);
}
/**
* @return The primary address prefix.
*
*/
public Optional primaryPeerAddressPrefix() {
return Optional.ofNullable(this.primaryPeerAddressPrefix);
}
/**
* @return The provisioning state of the express route circuit peering resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The reference to the RouteFilter resource.
*
*/
public Optional routeFilter() {
return Optional.ofNullable(this.routeFilter);
}
/**
* @return The secondary port.
*
*/
public Optional secondaryAzurePort() {
return Optional.ofNullable(this.secondaryAzurePort);
}
/**
* @return The secondary address prefix.
*
*/
public Optional secondaryPeerAddressPrefix() {
return Optional.ofNullable(this.secondaryPeerAddressPrefix);
}
/**
* @return The shared key.
*
*/
public Optional sharedKey() {
return Optional.ofNullable(this.sharedKey);
}
/**
* @return The peering state.
*
*/
public Optional state() {
return Optional.ofNullable(this.state);
}
/**
* @return The peering stats of express route circuit.
*
*/
public Optional stats() {
return Optional.ofNullable(this.stats);
}
/**
* @return Type of the resource.
*
*/
public String type() {
return this.type;
}
/**
* @return The VLAN ID.
*
*/
public Optional vlanId() {
return Optional.ofNullable(this.vlanId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetExpressRouteCircuitPeeringResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer azureASN;
private @Nullable List connections;
private String etag;
private @Nullable ExpressRouteConnectionIdResponse expressRouteConnection;
private @Nullable String gatewayManagerEtag;
private @Nullable String id;
private @Nullable Ipv6ExpressRouteCircuitPeeringConfigResponse ipv6PeeringConfig;
private String lastModifiedBy;
private @Nullable ExpressRouteCircuitPeeringConfigResponse microsoftPeeringConfig;
private @Nullable String name;
private @Nullable Double peerASN;
private List peeredConnections;
private @Nullable String peeringType;
private @Nullable String primaryAzurePort;
private @Nullable String primaryPeerAddressPrefix;
private String provisioningState;
private @Nullable SubResourceResponse routeFilter;
private @Nullable String secondaryAzurePort;
private @Nullable String secondaryPeerAddressPrefix;
private @Nullable String sharedKey;
private @Nullable String state;
private @Nullable ExpressRouteCircuitStatsResponse stats;
private String type;
private @Nullable Integer vlanId;
public Builder() {}
public Builder(GetExpressRouteCircuitPeeringResult defaults) {
Objects.requireNonNull(defaults);
this.azureASN = defaults.azureASN;
this.connections = defaults.connections;
this.etag = defaults.etag;
this.expressRouteConnection = defaults.expressRouteConnection;
this.gatewayManagerEtag = defaults.gatewayManagerEtag;
this.id = defaults.id;
this.ipv6PeeringConfig = defaults.ipv6PeeringConfig;
this.lastModifiedBy = defaults.lastModifiedBy;
this.microsoftPeeringConfig = defaults.microsoftPeeringConfig;
this.name = defaults.name;
this.peerASN = defaults.peerASN;
this.peeredConnections = defaults.peeredConnections;
this.peeringType = defaults.peeringType;
this.primaryAzurePort = defaults.primaryAzurePort;
this.primaryPeerAddressPrefix = defaults.primaryPeerAddressPrefix;
this.provisioningState = defaults.provisioningState;
this.routeFilter = defaults.routeFilter;
this.secondaryAzurePort = defaults.secondaryAzurePort;
this.secondaryPeerAddressPrefix = defaults.secondaryPeerAddressPrefix;
this.sharedKey = defaults.sharedKey;
this.state = defaults.state;
this.stats = defaults.stats;
this.type = defaults.type;
this.vlanId = defaults.vlanId;
}
@CustomType.Setter
public Builder azureASN(@Nullable Integer azureASN) {
this.azureASN = azureASN;
return this;
}
@CustomType.Setter
public Builder connections(@Nullable List connections) {
this.connections = connections;
return this;
}
public Builder connections(ExpressRouteCircuitConnectionResponse... connections) {
return connections(List.of(connections));
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetExpressRouteCircuitPeeringResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder expressRouteConnection(@Nullable ExpressRouteConnectionIdResponse expressRouteConnection) {
this.expressRouteConnection = expressRouteConnection;
return this;
}
@CustomType.Setter
public Builder gatewayManagerEtag(@Nullable String gatewayManagerEtag) {
this.gatewayManagerEtag = gatewayManagerEtag;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder ipv6PeeringConfig(@Nullable Ipv6ExpressRouteCircuitPeeringConfigResponse ipv6PeeringConfig) {
this.ipv6PeeringConfig = ipv6PeeringConfig;
return this;
}
@CustomType.Setter
public Builder lastModifiedBy(String lastModifiedBy) {
if (lastModifiedBy == null) {
throw new MissingRequiredPropertyException("GetExpressRouteCircuitPeeringResult", "lastModifiedBy");
}
this.lastModifiedBy = lastModifiedBy;
return this;
}
@CustomType.Setter
public Builder microsoftPeeringConfig(@Nullable ExpressRouteCircuitPeeringConfigResponse microsoftPeeringConfig) {
this.microsoftPeeringConfig = microsoftPeeringConfig;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder peerASN(@Nullable Double peerASN) {
this.peerASN = peerASN;
return this;
}
@CustomType.Setter
public Builder peeredConnections(List peeredConnections) {
if (peeredConnections == null) {
throw new MissingRequiredPropertyException("GetExpressRouteCircuitPeeringResult", "peeredConnections");
}
this.peeredConnections = peeredConnections;
return this;
}
public Builder peeredConnections(PeerExpressRouteCircuitConnectionResponse... peeredConnections) {
return peeredConnections(List.of(peeredConnections));
}
@CustomType.Setter
public Builder peeringType(@Nullable String peeringType) {
this.peeringType = peeringType;
return this;
}
@CustomType.Setter
public Builder primaryAzurePort(@Nullable String primaryAzurePort) {
this.primaryAzurePort = primaryAzurePort;
return this;
}
@CustomType.Setter
public Builder primaryPeerAddressPrefix(@Nullable String primaryPeerAddressPrefix) {
this.primaryPeerAddressPrefix = primaryPeerAddressPrefix;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetExpressRouteCircuitPeeringResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder routeFilter(@Nullable SubResourceResponse routeFilter) {
this.routeFilter = routeFilter;
return this;
}
@CustomType.Setter
public Builder secondaryAzurePort(@Nullable String secondaryAzurePort) {
this.secondaryAzurePort = secondaryAzurePort;
return this;
}
@CustomType.Setter
public Builder secondaryPeerAddressPrefix(@Nullable String secondaryPeerAddressPrefix) {
this.secondaryPeerAddressPrefix = secondaryPeerAddressPrefix;
return this;
}
@CustomType.Setter
public Builder sharedKey(@Nullable String sharedKey) {
this.sharedKey = sharedKey;
return this;
}
@CustomType.Setter
public Builder state(@Nullable String state) {
this.state = state;
return this;
}
@CustomType.Setter
public Builder stats(@Nullable ExpressRouteCircuitStatsResponse stats) {
this.stats = stats;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetExpressRouteCircuitPeeringResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder vlanId(@Nullable Integer vlanId) {
this.vlanId = vlanId;
return this;
}
public GetExpressRouteCircuitPeeringResult build() {
final var _resultValue = new GetExpressRouteCircuitPeeringResult();
_resultValue.azureASN = azureASN;
_resultValue.connections = connections;
_resultValue.etag = etag;
_resultValue.expressRouteConnection = expressRouteConnection;
_resultValue.gatewayManagerEtag = gatewayManagerEtag;
_resultValue.id = id;
_resultValue.ipv6PeeringConfig = ipv6PeeringConfig;
_resultValue.lastModifiedBy = lastModifiedBy;
_resultValue.microsoftPeeringConfig = microsoftPeeringConfig;
_resultValue.name = name;
_resultValue.peerASN = peerASN;
_resultValue.peeredConnections = peeredConnections;
_resultValue.peeringType = peeringType;
_resultValue.primaryAzurePort = primaryAzurePort;
_resultValue.primaryPeerAddressPrefix = primaryPeerAddressPrefix;
_resultValue.provisioningState = provisioningState;
_resultValue.routeFilter = routeFilter;
_resultValue.secondaryAzurePort = secondaryAzurePort;
_resultValue.secondaryPeerAddressPrefix = secondaryPeerAddressPrefix;
_resultValue.sharedKey = sharedKey;
_resultValue.state = state;
_resultValue.stats = stats;
_resultValue.type = type;
_resultValue.vlanId = vlanId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy