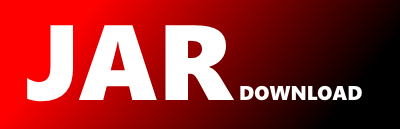
com.pulumi.azurenative.network.outputs.VirtualNetworkGatewayPolicyGroupResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.network.outputs;
import com.pulumi.azurenative.network.outputs.SubResourceResponse;
import com.pulumi.azurenative.network.outputs.VirtualNetworkGatewayPolicyGroupMemberResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class VirtualNetworkGatewayPolicyGroupResponse {
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
private String etag;
/**
* @return Resource ID.
*
*/
private @Nullable String id;
/**
* @return Shows if this is a Default VirtualNetworkGatewayPolicyGroup or not.
*
*/
private Boolean isDefault;
/**
* @return The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
private @Nullable String name;
/**
* @return Multiple PolicyMembers for VirtualNetworkGatewayPolicyGroup.
*
*/
private List policyMembers;
/**
* @return Priority for VirtualNetworkGatewayPolicyGroup.
*
*/
private Integer priority;
/**
* @return The provisioning state of the VirtualNetworkGatewayPolicyGroup resource.
*
*/
private String provisioningState;
/**
* @return List of references to vngClientConnectionConfigurations.
*
*/
private List vngClientConnectionConfigurations;
private VirtualNetworkGatewayPolicyGroupResponse() {}
/**
* @return A unique read-only string that changes whenever the resource is updated.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Resource ID.
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return Shows if this is a Default VirtualNetworkGatewayPolicyGroup or not.
*
*/
public Boolean isDefault() {
return this.isDefault;
}
/**
* @return The name of the resource that is unique within a resource group. This name can be used to access the resource.
*
*/
public Optional name() {
return Optional.ofNullable(this.name);
}
/**
* @return Multiple PolicyMembers for VirtualNetworkGatewayPolicyGroup.
*
*/
public List policyMembers() {
return this.policyMembers;
}
/**
* @return Priority for VirtualNetworkGatewayPolicyGroup.
*
*/
public Integer priority() {
return this.priority;
}
/**
* @return The provisioning state of the VirtualNetworkGatewayPolicyGroup resource.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return List of references to vngClientConnectionConfigurations.
*
*/
public List vngClientConnectionConfigurations() {
return this.vngClientConnectionConfigurations;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(VirtualNetworkGatewayPolicyGroupResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String etag;
private @Nullable String id;
private Boolean isDefault;
private @Nullable String name;
private List policyMembers;
private Integer priority;
private String provisioningState;
private List vngClientConnectionConfigurations;
public Builder() {}
public Builder(VirtualNetworkGatewayPolicyGroupResponse defaults) {
Objects.requireNonNull(defaults);
this.etag = defaults.etag;
this.id = defaults.id;
this.isDefault = defaults.isDefault;
this.name = defaults.name;
this.policyMembers = defaults.policyMembers;
this.priority = defaults.priority;
this.provisioningState = defaults.provisioningState;
this.vngClientConnectionConfigurations = defaults.vngClientConnectionConfigurations;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("VirtualNetworkGatewayPolicyGroupResponse", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder isDefault(Boolean isDefault) {
if (isDefault == null) {
throw new MissingRequiredPropertyException("VirtualNetworkGatewayPolicyGroupResponse", "isDefault");
}
this.isDefault = isDefault;
return this;
}
@CustomType.Setter
public Builder name(@Nullable String name) {
this.name = name;
return this;
}
@CustomType.Setter
public Builder policyMembers(List policyMembers) {
if (policyMembers == null) {
throw new MissingRequiredPropertyException("VirtualNetworkGatewayPolicyGroupResponse", "policyMembers");
}
this.policyMembers = policyMembers;
return this;
}
public Builder policyMembers(VirtualNetworkGatewayPolicyGroupMemberResponse... policyMembers) {
return policyMembers(List.of(policyMembers));
}
@CustomType.Setter
public Builder priority(Integer priority) {
if (priority == null) {
throw new MissingRequiredPropertyException("VirtualNetworkGatewayPolicyGroupResponse", "priority");
}
this.priority = priority;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("VirtualNetworkGatewayPolicyGroupResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder vngClientConnectionConfigurations(List vngClientConnectionConfigurations) {
if (vngClientConnectionConfigurations == null) {
throw new MissingRequiredPropertyException("VirtualNetworkGatewayPolicyGroupResponse", "vngClientConnectionConfigurations");
}
this.vngClientConnectionConfigurations = vngClientConnectionConfigurations;
return this;
}
public Builder vngClientConnectionConfigurations(SubResourceResponse... vngClientConnectionConfigurations) {
return vngClientConnectionConfigurations(List.of(vngClientConnectionConfigurations));
}
public VirtualNetworkGatewayPolicyGroupResponse build() {
final var _resultValue = new VirtualNetworkGatewayPolicyGroupResponse();
_resultValue.etag = etag;
_resultValue.id = id;
_resultValue.isDefault = isDefault;
_resultValue.name = name;
_resultValue.policyMembers = policyMembers;
_resultValue.priority = priority;
_resultValue.provisioningState = provisioningState;
_resultValue.vngClientConnectionConfigurations = vngClientConnectionConfigurations;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy