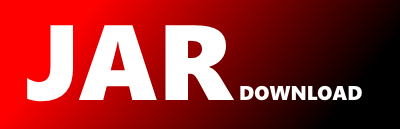
com.pulumi.azurenative.networkanalytics.DataProductArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkanalytics;
import com.pulumi.azurenative.networkanalytics.enums.ControlState;
import com.pulumi.azurenative.networkanalytics.inputs.DataProductNetworkAclsArgs;
import com.pulumi.azurenative.networkanalytics.inputs.EncryptionKeyDetailsArgs;
import com.pulumi.azurenative.networkanalytics.inputs.ManagedResourceGroupConfigurationArgs;
import com.pulumi.azurenative.networkanalytics.inputs.ManagedServiceIdentityArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DataProductArgs extends com.pulumi.resources.ResourceArgs {
public static final DataProductArgs Empty = new DataProductArgs();
/**
* Current configured minor version of the data product resource.
*
*/
@Import(name="currentMinorVersion")
private @Nullable Output currentMinorVersion;
/**
* @return Current configured minor version of the data product resource.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy