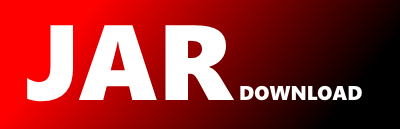
com.pulumi.azurenative.networkcloud.AgentPoolArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkcloud;
import com.pulumi.azurenative.networkcloud.enums.AgentPoolMode;
import com.pulumi.azurenative.networkcloud.inputs.AdministratorConfigurationArgs;
import com.pulumi.azurenative.networkcloud.inputs.AgentOptionsArgs;
import com.pulumi.azurenative.networkcloud.inputs.AgentPoolUpgradeSettingsArgs;
import com.pulumi.azurenative.networkcloud.inputs.AttachedNetworkConfigurationArgs;
import com.pulumi.azurenative.networkcloud.inputs.ExtendedLocationArgs;
import com.pulumi.azurenative.networkcloud.inputs.KubernetesLabelArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AgentPoolArgs extends com.pulumi.resources.ResourceArgs {
public static final AgentPoolArgs Empty = new AgentPoolArgs();
/**
* The administrator credentials to be used for the nodes in this agent pool.
*
*/
@Import(name="administratorConfiguration")
private @Nullable Output administratorConfiguration;
/**
* @return The administrator credentials to be used for the nodes in this agent pool.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy