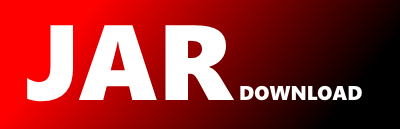
com.pulumi.azurenative.networkcloud.L3NetworkArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.networkcloud;
import com.pulumi.azurenative.networkcloud.enums.HybridAksIpamEnabled;
import com.pulumi.azurenative.networkcloud.enums.HybridAksPluginType;
import com.pulumi.azurenative.networkcloud.enums.IpAllocationType;
import com.pulumi.azurenative.networkcloud.inputs.ExtendedLocationArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class L3NetworkArgs extends com.pulumi.resources.ResourceArgs {
public static final L3NetworkArgs Empty = new L3NetworkArgs();
/**
* The extended location of the cluster associated with the resource.
*
*/
@Import(name="extendedLocation", required=true)
private Output extendedLocation;
/**
* @return The extended location of the cluster associated with the resource.
*
*/
public Output extendedLocation() {
return this.extendedLocation;
}
/**
* Field Deprecated. The field was previously optional, now it will have no defined behavior and will be ignored. The indicator of whether or not to disable IPAM allocation on the network attachment definition injected into the Hybrid AKS Cluster.
*
*/
@Import(name="hybridAksIpamEnabled")
private @Nullable Output> hybridAksIpamEnabled;
/**
* @return Field Deprecated. The field was previously optional, now it will have no defined behavior and will be ignored. The indicator of whether or not to disable IPAM allocation on the network attachment definition injected into the Hybrid AKS Cluster.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy