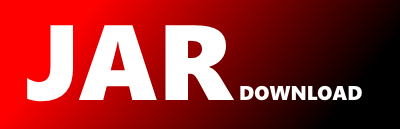
com.pulumi.azurenative.operationalinsights.DataExportArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.operationalinsights;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DataExportArgs extends com.pulumi.resources.ResourceArgs {
public static final DataExportArgs Empty = new DataExportArgs();
/**
* The latest data export rule modification time.
*
*/
@Import(name="createdDate")
private @Nullable Output createdDate;
/**
* @return The latest data export rule modification time.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy