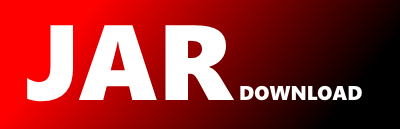
com.pulumi.azurenative.operationalinsights.outputs.GetMachineGroupResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.operationalinsights.outputs;
import com.pulumi.azurenative.operationalinsights.outputs.MachineReferenceWithHintsResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetMachineGroupResult {
/**
* @return Count of machines in this group. The value of count may be bigger than the number of machines in case of the group has been truncated due to exceeding the max number of machines a group can handle.
*
*/
private @Nullable Integer count;
/**
* @return User defined name for the group
*
*/
private String displayName;
/**
* @return Resource ETAG.
*
*/
private @Nullable String etag;
/**
* @return Type of the machine group
*
*/
private @Nullable String groupType;
/**
* @return Resource identifier.
*
*/
private String id;
/**
* @return Additional resource type qualifier.
* Expected value is 'machineGroup'.
*
*/
private String kind;
/**
* @return References of the machines in this group. The hints within each reference do not represent the current value of the corresponding fields. They are a snapshot created during the last time the machine group was updated.
*
*/
private @Nullable List machines;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return Resource type.
*
*/
private String type;
private GetMachineGroupResult() {}
/**
* @return Count of machines in this group. The value of count may be bigger than the number of machines in case of the group has been truncated due to exceeding the max number of machines a group can handle.
*
*/
public Optional count() {
return Optional.ofNullable(this.count);
}
/**
* @return User defined name for the group
*
*/
public String displayName() {
return this.displayName;
}
/**
* @return Resource ETAG.
*
*/
public Optional etag() {
return Optional.ofNullable(this.etag);
}
/**
* @return Type of the machine group
*
*/
public Optional groupType() {
return Optional.ofNullable(this.groupType);
}
/**
* @return Resource identifier.
*
*/
public String id() {
return this.id;
}
/**
* @return Additional resource type qualifier.
* Expected value is 'machineGroup'.
*
*/
public String kind() {
return this.kind;
}
/**
* @return References of the machines in this group. The hints within each reference do not represent the current value of the corresponding fields. They are a snapshot created during the last time the machine group was updated.
*
*/
public List machines() {
return this.machines == null ? List.of() : this.machines;
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetMachineGroupResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Integer count;
private String displayName;
private @Nullable String etag;
private @Nullable String groupType;
private String id;
private String kind;
private @Nullable List machines;
private String name;
private String type;
public Builder() {}
public Builder(GetMachineGroupResult defaults) {
Objects.requireNonNull(defaults);
this.count = defaults.count;
this.displayName = defaults.displayName;
this.etag = defaults.etag;
this.groupType = defaults.groupType;
this.id = defaults.id;
this.kind = defaults.kind;
this.machines = defaults.machines;
this.name = defaults.name;
this.type = defaults.type;
}
@CustomType.Setter
public Builder count(@Nullable Integer count) {
this.count = count;
return this;
}
@CustomType.Setter
public Builder displayName(String displayName) {
if (displayName == null) {
throw new MissingRequiredPropertyException("GetMachineGroupResult", "displayName");
}
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder etag(@Nullable String etag) {
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder groupType(@Nullable String groupType) {
this.groupType = groupType;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetMachineGroupResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kind(String kind) {
if (kind == null) {
throw new MissingRequiredPropertyException("GetMachineGroupResult", "kind");
}
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder machines(@Nullable List machines) {
this.machines = machines;
return this;
}
public Builder machines(MachineReferenceWithHintsResponse... machines) {
return machines(List.of(machines));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetMachineGroupResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetMachineGroupResult", "type");
}
this.type = type;
return this;
}
public GetMachineGroupResult build() {
final var _resultValue = new GetMachineGroupResult();
_resultValue.count = count;
_resultValue.displayName = displayName;
_resultValue.etag = etag;
_resultValue.groupType = groupType;
_resultValue.id = id;
_resultValue.kind = kind;
_resultValue.machines = machines;
_resultValue.name = name;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy