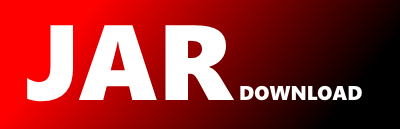
com.pulumi.azurenative.recoveryservices.outputs.InMageRcmFailbackMobilityAgentDetailsResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.recoveryservices.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
@CustomType
public final class InMageRcmFailbackMobilityAgentDetailsResponse {
/**
* @return The agent version expiry date.
*
*/
private String agentVersionExpiryDate;
/**
* @return The driver version.
*
*/
private String driverVersion;
/**
* @return The driver version expiry date.
*
*/
private String driverVersionExpiryDate;
/**
* @return A value indicating whether agent is upgradeable or not.
*
*/
private String isUpgradeable;
/**
* @return The time of the last heartbeat received from the agent.
*
*/
private String lastHeartbeatUtc;
/**
* @return The latest upgradeable version available without reboot.
*
*/
private String latestUpgradableVersionWithoutReboot;
/**
* @return The latest agent version available.
*
*/
private String latestVersion;
/**
* @return The whether update is possible or not.
*
*/
private List reasonsBlockingUpgrade;
/**
* @return The agent version.
*
*/
private String version;
private InMageRcmFailbackMobilityAgentDetailsResponse() {}
/**
* @return The agent version expiry date.
*
*/
public String agentVersionExpiryDate() {
return this.agentVersionExpiryDate;
}
/**
* @return The driver version.
*
*/
public String driverVersion() {
return this.driverVersion;
}
/**
* @return The driver version expiry date.
*
*/
public String driverVersionExpiryDate() {
return this.driverVersionExpiryDate;
}
/**
* @return A value indicating whether agent is upgradeable or not.
*
*/
public String isUpgradeable() {
return this.isUpgradeable;
}
/**
* @return The time of the last heartbeat received from the agent.
*
*/
public String lastHeartbeatUtc() {
return this.lastHeartbeatUtc;
}
/**
* @return The latest upgradeable version available without reboot.
*
*/
public String latestUpgradableVersionWithoutReboot() {
return this.latestUpgradableVersionWithoutReboot;
}
/**
* @return The latest agent version available.
*
*/
public String latestVersion() {
return this.latestVersion;
}
/**
* @return The whether update is possible or not.
*
*/
public List reasonsBlockingUpgrade() {
return this.reasonsBlockingUpgrade;
}
/**
* @return The agent version.
*
*/
public String version() {
return this.version;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(InMageRcmFailbackMobilityAgentDetailsResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String agentVersionExpiryDate;
private String driverVersion;
private String driverVersionExpiryDate;
private String isUpgradeable;
private String lastHeartbeatUtc;
private String latestUpgradableVersionWithoutReboot;
private String latestVersion;
private List reasonsBlockingUpgrade;
private String version;
public Builder() {}
public Builder(InMageRcmFailbackMobilityAgentDetailsResponse defaults) {
Objects.requireNonNull(defaults);
this.agentVersionExpiryDate = defaults.agentVersionExpiryDate;
this.driverVersion = defaults.driverVersion;
this.driverVersionExpiryDate = defaults.driverVersionExpiryDate;
this.isUpgradeable = defaults.isUpgradeable;
this.lastHeartbeatUtc = defaults.lastHeartbeatUtc;
this.latestUpgradableVersionWithoutReboot = defaults.latestUpgradableVersionWithoutReboot;
this.latestVersion = defaults.latestVersion;
this.reasonsBlockingUpgrade = defaults.reasonsBlockingUpgrade;
this.version = defaults.version;
}
@CustomType.Setter
public Builder agentVersionExpiryDate(String agentVersionExpiryDate) {
if (agentVersionExpiryDate == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackMobilityAgentDetailsResponse", "agentVersionExpiryDate");
}
this.agentVersionExpiryDate = agentVersionExpiryDate;
return this;
}
@CustomType.Setter
public Builder driverVersion(String driverVersion) {
if (driverVersion == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackMobilityAgentDetailsResponse", "driverVersion");
}
this.driverVersion = driverVersion;
return this;
}
@CustomType.Setter
public Builder driverVersionExpiryDate(String driverVersionExpiryDate) {
if (driverVersionExpiryDate == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackMobilityAgentDetailsResponse", "driverVersionExpiryDate");
}
this.driverVersionExpiryDate = driverVersionExpiryDate;
return this;
}
@CustomType.Setter
public Builder isUpgradeable(String isUpgradeable) {
if (isUpgradeable == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackMobilityAgentDetailsResponse", "isUpgradeable");
}
this.isUpgradeable = isUpgradeable;
return this;
}
@CustomType.Setter
public Builder lastHeartbeatUtc(String lastHeartbeatUtc) {
if (lastHeartbeatUtc == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackMobilityAgentDetailsResponse", "lastHeartbeatUtc");
}
this.lastHeartbeatUtc = lastHeartbeatUtc;
return this;
}
@CustomType.Setter
public Builder latestUpgradableVersionWithoutReboot(String latestUpgradableVersionWithoutReboot) {
if (latestUpgradableVersionWithoutReboot == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackMobilityAgentDetailsResponse", "latestUpgradableVersionWithoutReboot");
}
this.latestUpgradableVersionWithoutReboot = latestUpgradableVersionWithoutReboot;
return this;
}
@CustomType.Setter
public Builder latestVersion(String latestVersion) {
if (latestVersion == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackMobilityAgentDetailsResponse", "latestVersion");
}
this.latestVersion = latestVersion;
return this;
}
@CustomType.Setter
public Builder reasonsBlockingUpgrade(List reasonsBlockingUpgrade) {
if (reasonsBlockingUpgrade == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackMobilityAgentDetailsResponse", "reasonsBlockingUpgrade");
}
this.reasonsBlockingUpgrade = reasonsBlockingUpgrade;
return this;
}
public Builder reasonsBlockingUpgrade(String... reasonsBlockingUpgrade) {
return reasonsBlockingUpgrade(List.of(reasonsBlockingUpgrade));
}
@CustomType.Setter
public Builder version(String version) {
if (version == null) {
throw new MissingRequiredPropertyException("InMageRcmFailbackMobilityAgentDetailsResponse", "version");
}
this.version = version;
return this;
}
public InMageRcmFailbackMobilityAgentDetailsResponse build() {
final var _resultValue = new InMageRcmFailbackMobilityAgentDetailsResponse();
_resultValue.agentVersionExpiryDate = agentVersionExpiryDate;
_resultValue.driverVersion = driverVersion;
_resultValue.driverVersionExpiryDate = driverVersionExpiryDate;
_resultValue.isUpgradeable = isUpgradeable;
_resultValue.lastHeartbeatUtc = lastHeartbeatUtc;
_resultValue.latestUpgradableVersionWithoutReboot = latestUpgradableVersionWithoutReboot;
_resultValue.latestVersion = latestVersion;
_resultValue.reasonsBlockingUpgrade = reasonsBlockingUpgrade;
_resultValue.version = version;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy