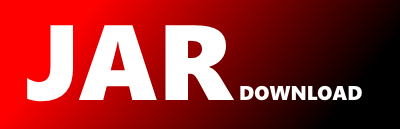
com.pulumi.azurenative.scom.outputs.MonitoringInstancePropertiesResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.scom.outputs;
import com.pulumi.azurenative.scom.outputs.AzureHybridBenefitPropertiesResponse;
import com.pulumi.azurenative.scom.outputs.DatabaseInstancePropertiesResponse;
import com.pulumi.azurenative.scom.outputs.DomainControllerPropertiesResponse;
import com.pulumi.azurenative.scom.outputs.DomainUserCredentialsResponse;
import com.pulumi.azurenative.scom.outputs.GmsaDetailsResponse;
import com.pulumi.azurenative.scom.outputs.LogAnalyticsConfigurationResponse;
import com.pulumi.azurenative.scom.outputs.ManagedInstanceOperationStatusResponse;
import com.pulumi.azurenative.scom.outputs.ManagementServerPropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class MonitoringInstancePropertiesResponse {
/**
* @return The properties to enable Azure Hybrid benefit for various SCOM infrastructure license.
*
*/
private @Nullable AzureHybridBenefitPropertiesResponse azureHybridBenefit;
/**
* @return The database instance where the SCOM Operational and Warehouse databases will be stored.
*
*/
private @Nullable DatabaseInstancePropertiesResponse databaseInstance;
/**
* @return Domain controller details
*
*/
private @Nullable DomainControllerPropertiesResponse domainController;
/**
* @return Domain user which will be used to join VMs to domain and login to VMs.
*
*/
private @Nullable DomainUserCredentialsResponse domainUserCredentials;
/**
* @return Gmsa Details for load balancer and vmss
*
*/
private @Nullable GmsaDetailsResponse gmsaDetails;
/**
* @return Details of Log Analytics workspace and data being ingested.
*
*/
private LogAnalyticsConfigurationResponse logAnalyticsProperties;
/**
* @return List of management server endpoints
*
*/
private List managementEndpoints;
/**
* @return Gets status of current and latest SCOM managed instance operations.
*
*/
private List operationsStatus;
/**
* @return SCOM product version to be installed on instance
*
*/
private String productVersion;
/**
* @return Gets or sets the provisioning state.
*
*/
private String provisioningState;
/**
* @return Virtual Network subnet id on which Aquila instance will be provisioned
*
*/
private @Nullable String vNetSubnetId;
private MonitoringInstancePropertiesResponse() {}
/**
* @return The properties to enable Azure Hybrid benefit for various SCOM infrastructure license.
*
*/
public Optional azureHybridBenefit() {
return Optional.ofNullable(this.azureHybridBenefit);
}
/**
* @return The database instance where the SCOM Operational and Warehouse databases will be stored.
*
*/
public Optional databaseInstance() {
return Optional.ofNullable(this.databaseInstance);
}
/**
* @return Domain controller details
*
*/
public Optional domainController() {
return Optional.ofNullable(this.domainController);
}
/**
* @return Domain user which will be used to join VMs to domain and login to VMs.
*
*/
public Optional domainUserCredentials() {
return Optional.ofNullable(this.domainUserCredentials);
}
/**
* @return Gmsa Details for load balancer and vmss
*
*/
public Optional gmsaDetails() {
return Optional.ofNullable(this.gmsaDetails);
}
/**
* @return Details of Log Analytics workspace and data being ingested.
*
*/
public LogAnalyticsConfigurationResponse logAnalyticsProperties() {
return this.logAnalyticsProperties;
}
/**
* @return List of management server endpoints
*
*/
public List managementEndpoints() {
return this.managementEndpoints;
}
/**
* @return Gets status of current and latest SCOM managed instance operations.
*
*/
public List operationsStatus() {
return this.operationsStatus;
}
/**
* @return SCOM product version to be installed on instance
*
*/
public String productVersion() {
return this.productVersion;
}
/**
* @return Gets or sets the provisioning state.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return Virtual Network subnet id on which Aquila instance will be provisioned
*
*/
public Optional vNetSubnetId() {
return Optional.ofNullable(this.vNetSubnetId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(MonitoringInstancePropertiesResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable AzureHybridBenefitPropertiesResponse azureHybridBenefit;
private @Nullable DatabaseInstancePropertiesResponse databaseInstance;
private @Nullable DomainControllerPropertiesResponse domainController;
private @Nullable DomainUserCredentialsResponse domainUserCredentials;
private @Nullable GmsaDetailsResponse gmsaDetails;
private LogAnalyticsConfigurationResponse logAnalyticsProperties;
private List managementEndpoints;
private List operationsStatus;
private String productVersion;
private String provisioningState;
private @Nullable String vNetSubnetId;
public Builder() {}
public Builder(MonitoringInstancePropertiesResponse defaults) {
Objects.requireNonNull(defaults);
this.azureHybridBenefit = defaults.azureHybridBenefit;
this.databaseInstance = defaults.databaseInstance;
this.domainController = defaults.domainController;
this.domainUserCredentials = defaults.domainUserCredentials;
this.gmsaDetails = defaults.gmsaDetails;
this.logAnalyticsProperties = defaults.logAnalyticsProperties;
this.managementEndpoints = defaults.managementEndpoints;
this.operationsStatus = defaults.operationsStatus;
this.productVersion = defaults.productVersion;
this.provisioningState = defaults.provisioningState;
this.vNetSubnetId = defaults.vNetSubnetId;
}
@CustomType.Setter
public Builder azureHybridBenefit(@Nullable AzureHybridBenefitPropertiesResponse azureHybridBenefit) {
this.azureHybridBenefit = azureHybridBenefit;
return this;
}
@CustomType.Setter
public Builder databaseInstance(@Nullable DatabaseInstancePropertiesResponse databaseInstance) {
this.databaseInstance = databaseInstance;
return this;
}
@CustomType.Setter
public Builder domainController(@Nullable DomainControllerPropertiesResponse domainController) {
this.domainController = domainController;
return this;
}
@CustomType.Setter
public Builder domainUserCredentials(@Nullable DomainUserCredentialsResponse domainUserCredentials) {
this.domainUserCredentials = domainUserCredentials;
return this;
}
@CustomType.Setter
public Builder gmsaDetails(@Nullable GmsaDetailsResponse gmsaDetails) {
this.gmsaDetails = gmsaDetails;
return this;
}
@CustomType.Setter
public Builder logAnalyticsProperties(LogAnalyticsConfigurationResponse logAnalyticsProperties) {
if (logAnalyticsProperties == null) {
throw new MissingRequiredPropertyException("MonitoringInstancePropertiesResponse", "logAnalyticsProperties");
}
this.logAnalyticsProperties = logAnalyticsProperties;
return this;
}
@CustomType.Setter
public Builder managementEndpoints(List managementEndpoints) {
if (managementEndpoints == null) {
throw new MissingRequiredPropertyException("MonitoringInstancePropertiesResponse", "managementEndpoints");
}
this.managementEndpoints = managementEndpoints;
return this;
}
public Builder managementEndpoints(ManagementServerPropertiesResponse... managementEndpoints) {
return managementEndpoints(List.of(managementEndpoints));
}
@CustomType.Setter
public Builder operationsStatus(List operationsStatus) {
if (operationsStatus == null) {
throw new MissingRequiredPropertyException("MonitoringInstancePropertiesResponse", "operationsStatus");
}
this.operationsStatus = operationsStatus;
return this;
}
public Builder operationsStatus(ManagedInstanceOperationStatusResponse... operationsStatus) {
return operationsStatus(List.of(operationsStatus));
}
@CustomType.Setter
public Builder productVersion(String productVersion) {
if (productVersion == null) {
throw new MissingRequiredPropertyException("MonitoringInstancePropertiesResponse", "productVersion");
}
this.productVersion = productVersion;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("MonitoringInstancePropertiesResponse", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder vNetSubnetId(@Nullable String vNetSubnetId) {
this.vNetSubnetId = vNetSubnetId;
return this;
}
public MonitoringInstancePropertiesResponse build() {
final var _resultValue = new MonitoringInstancePropertiesResponse();
_resultValue.azureHybridBenefit = azureHybridBenefit;
_resultValue.databaseInstance = databaseInstance;
_resultValue.domainController = domainController;
_resultValue.domainUserCredentials = domainUserCredentials;
_resultValue.gmsaDetails = gmsaDetails;
_resultValue.logAnalyticsProperties = logAnalyticsProperties;
_resultValue.managementEndpoints = managementEndpoints;
_resultValue.operationsStatus = operationsStatus;
_resultValue.productVersion = productVersion;
_resultValue.provisioningState = provisioningState;
_resultValue.vNetSubnetId = vNetSubnetId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy