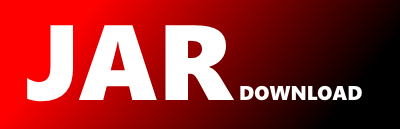
com.pulumi.azurenative.securityinsights.outputs.GetBookmarkResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.securityinsights.outputs;
import com.pulumi.azurenative.securityinsights.outputs.IncidentInfoResponse;
import com.pulumi.azurenative.securityinsights.outputs.SystemDataResponse;
import com.pulumi.azurenative.securityinsights.outputs.UserInfoResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetBookmarkResult {
/**
* @return The time the bookmark was created
*
*/
private @Nullable String created;
/**
* @return Describes a user that created the bookmark
*
*/
private @Nullable UserInfoResponse createdBy;
/**
* @return The display name of the bookmark
*
*/
private String displayName;
/**
* @return Etag of the azure resource
*
*/
private @Nullable String etag;
/**
* @return The bookmark event time
*
*/
private @Nullable String eventTime;
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
private String id;
/**
* @return Describes an incident that relates to bookmark
*
*/
private @Nullable IncidentInfoResponse incidentInfo;
/**
* @return List of labels relevant to this bookmark
*
*/
private @Nullable List labels;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The notes of the bookmark
*
*/
private @Nullable String notes;
/**
* @return The query of the bookmark.
*
*/
private String query;
/**
* @return The end time for the query
*
*/
private @Nullable String queryEndTime;
/**
* @return The query result of the bookmark.
*
*/
private @Nullable String queryResult;
/**
* @return The start time for the query
*
*/
private @Nullable String queryStartTime;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
/**
* @return The last time the bookmark was updated
*
*/
private @Nullable String updated;
/**
* @return Describes a user that updated the bookmark
*
*/
private @Nullable UserInfoResponse updatedBy;
private GetBookmarkResult() {}
/**
* @return The time the bookmark was created
*
*/
public Optional created() {
return Optional.ofNullable(this.created);
}
/**
* @return Describes a user that created the bookmark
*
*/
public Optional createdBy() {
return Optional.ofNullable(this.createdBy);
}
/**
* @return The display name of the bookmark
*
*/
public String displayName() {
return this.displayName;
}
/**
* @return Etag of the azure resource
*
*/
public Optional etag() {
return Optional.ofNullable(this.etag);
}
/**
* @return The bookmark event time
*
*/
public Optional eventTime() {
return Optional.ofNullable(this.eventTime);
}
/**
* @return Fully qualified resource ID for the resource. E.g. "/subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}"
*
*/
public String id() {
return this.id;
}
/**
* @return Describes an incident that relates to bookmark
*
*/
public Optional incidentInfo() {
return Optional.ofNullable(this.incidentInfo);
}
/**
* @return List of labels relevant to this bookmark
*
*/
public List labels() {
return this.labels == null ? List.of() : this.labels;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return The notes of the bookmark
*
*/
public Optional notes() {
return Optional.ofNullable(this.notes);
}
/**
* @return The query of the bookmark.
*
*/
public String query() {
return this.query;
}
/**
* @return The end time for the query
*
*/
public Optional queryEndTime() {
return Optional.ofNullable(this.queryEndTime);
}
/**
* @return The query result of the bookmark.
*
*/
public Optional queryResult() {
return Optional.ofNullable(this.queryResult);
}
/**
* @return The start time for the query
*
*/
public Optional queryStartTime() {
return Optional.ofNullable(this.queryStartTime);
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
/**
* @return The last time the bookmark was updated
*
*/
public Optional updated() {
return Optional.ofNullable(this.updated);
}
/**
* @return Describes a user that updated the bookmark
*
*/
public Optional updatedBy() {
return Optional.ofNullable(this.updatedBy);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetBookmarkResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String created;
private @Nullable UserInfoResponse createdBy;
private String displayName;
private @Nullable String etag;
private @Nullable String eventTime;
private String id;
private @Nullable IncidentInfoResponse incidentInfo;
private @Nullable List labels;
private String name;
private @Nullable String notes;
private String query;
private @Nullable String queryEndTime;
private @Nullable String queryResult;
private @Nullable String queryStartTime;
private SystemDataResponse systemData;
private String type;
private @Nullable String updated;
private @Nullable UserInfoResponse updatedBy;
public Builder() {}
public Builder(GetBookmarkResult defaults) {
Objects.requireNonNull(defaults);
this.created = defaults.created;
this.createdBy = defaults.createdBy;
this.displayName = defaults.displayName;
this.etag = defaults.etag;
this.eventTime = defaults.eventTime;
this.id = defaults.id;
this.incidentInfo = defaults.incidentInfo;
this.labels = defaults.labels;
this.name = defaults.name;
this.notes = defaults.notes;
this.query = defaults.query;
this.queryEndTime = defaults.queryEndTime;
this.queryResult = defaults.queryResult;
this.queryStartTime = defaults.queryStartTime;
this.systemData = defaults.systemData;
this.type = defaults.type;
this.updated = defaults.updated;
this.updatedBy = defaults.updatedBy;
}
@CustomType.Setter
public Builder created(@Nullable String created) {
this.created = created;
return this;
}
@CustomType.Setter
public Builder createdBy(@Nullable UserInfoResponse createdBy) {
this.createdBy = createdBy;
return this;
}
@CustomType.Setter
public Builder displayName(String displayName) {
if (displayName == null) {
throw new MissingRequiredPropertyException("GetBookmarkResult", "displayName");
}
this.displayName = displayName;
return this;
}
@CustomType.Setter
public Builder etag(@Nullable String etag) {
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder eventTime(@Nullable String eventTime) {
this.eventTime = eventTime;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetBookmarkResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder incidentInfo(@Nullable IncidentInfoResponse incidentInfo) {
this.incidentInfo = incidentInfo;
return this;
}
@CustomType.Setter
public Builder labels(@Nullable List labels) {
this.labels = labels;
return this;
}
public Builder labels(String... labels) {
return labels(List.of(labels));
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetBookmarkResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder notes(@Nullable String notes) {
this.notes = notes;
return this;
}
@CustomType.Setter
public Builder query(String query) {
if (query == null) {
throw new MissingRequiredPropertyException("GetBookmarkResult", "query");
}
this.query = query;
return this;
}
@CustomType.Setter
public Builder queryEndTime(@Nullable String queryEndTime) {
this.queryEndTime = queryEndTime;
return this;
}
@CustomType.Setter
public Builder queryResult(@Nullable String queryResult) {
this.queryResult = queryResult;
return this;
}
@CustomType.Setter
public Builder queryStartTime(@Nullable String queryStartTime) {
this.queryStartTime = queryStartTime;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetBookmarkResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetBookmarkResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder updated(@Nullable String updated) {
this.updated = updated;
return this;
}
@CustomType.Setter
public Builder updatedBy(@Nullable UserInfoResponse updatedBy) {
this.updatedBy = updatedBy;
return this;
}
public GetBookmarkResult build() {
final var _resultValue = new GetBookmarkResult();
_resultValue.created = created;
_resultValue.createdBy = createdBy;
_resultValue.displayName = displayName;
_resultValue.etag = etag;
_resultValue.eventTime = eventTime;
_resultValue.id = id;
_resultValue.incidentInfo = incidentInfo;
_resultValue.labels = labels;
_resultValue.name = name;
_resultValue.notes = notes;
_resultValue.query = query;
_resultValue.queryEndTime = queryEndTime;
_resultValue.queryResult = queryResult;
_resultValue.queryStartTime = queryStartTime;
_resultValue.systemData = systemData;
_resultValue.type = type;
_resultValue.updated = updated;
_resultValue.updatedBy = updatedBy;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy