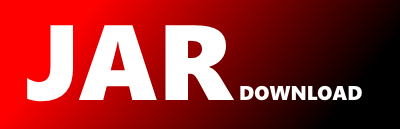
com.pulumi.azurenative.sql.outputs.GetDatabaseThreatDetectionPolicyResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.sql.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetDatabaseThreatDetectionPolicyResult {
/**
* @return Specifies the semicolon-separated list of alerts that are disabled, or empty string to disable no alerts. Possible values: Sql_Injection; Sql_Injection_Vulnerability; Access_Anomaly; Data_Exfiltration; Unsafe_Action.
*
*/
private @Nullable String disabledAlerts;
/**
* @return Specifies that the alert is sent to the account administrators.
*
*/
private @Nullable String emailAccountAdmins;
/**
* @return Specifies the semicolon-separated list of e-mail addresses to which the alert is sent.
*
*/
private @Nullable String emailAddresses;
/**
* @return Resource ID.
*
*/
private String id;
/**
* @return Resource kind.
*
*/
private String kind;
/**
* @return The geo-location where the resource lives
*
*/
private @Nullable String location;
/**
* @return Resource name.
*
*/
private String name;
/**
* @return Specifies the number of days to keep in the Threat Detection audit logs.
*
*/
private @Nullable Integer retentionDays;
/**
* @return Specifies the state of the policy. If state is Enabled, storageEndpoint and storageAccountAccessKey are required.
*
*/
private String state;
/**
* @return Specifies the blob storage endpoint (e.g. https://MyAccount.blob.core.windows.net). This blob storage will hold all Threat Detection audit logs. If state is Enabled, storageEndpoint is required.
*
*/
private @Nullable String storageEndpoint;
/**
* @return Resource type.
*
*/
private String type;
/**
* @return Specifies whether to use the default server policy.
*
*/
private @Nullable String useServerDefault;
private GetDatabaseThreatDetectionPolicyResult() {}
/**
* @return Specifies the semicolon-separated list of alerts that are disabled, or empty string to disable no alerts. Possible values: Sql_Injection; Sql_Injection_Vulnerability; Access_Anomaly; Data_Exfiltration; Unsafe_Action.
*
*/
public Optional disabledAlerts() {
return Optional.ofNullable(this.disabledAlerts);
}
/**
* @return Specifies that the alert is sent to the account administrators.
*
*/
public Optional emailAccountAdmins() {
return Optional.ofNullable(this.emailAccountAdmins);
}
/**
* @return Specifies the semicolon-separated list of e-mail addresses to which the alert is sent.
*
*/
public Optional emailAddresses() {
return Optional.ofNullable(this.emailAddresses);
}
/**
* @return Resource ID.
*
*/
public String id() {
return this.id;
}
/**
* @return Resource kind.
*
*/
public String kind() {
return this.kind;
}
/**
* @return The geo-location where the resource lives
*
*/
public Optional location() {
return Optional.ofNullable(this.location);
}
/**
* @return Resource name.
*
*/
public String name() {
return this.name;
}
/**
* @return Specifies the number of days to keep in the Threat Detection audit logs.
*
*/
public Optional retentionDays() {
return Optional.ofNullable(this.retentionDays);
}
/**
* @return Specifies the state of the policy. If state is Enabled, storageEndpoint and storageAccountAccessKey are required.
*
*/
public String state() {
return this.state;
}
/**
* @return Specifies the blob storage endpoint (e.g. https://MyAccount.blob.core.windows.net). This blob storage will hold all Threat Detection audit logs. If state is Enabled, storageEndpoint is required.
*
*/
public Optional storageEndpoint() {
return Optional.ofNullable(this.storageEndpoint);
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
/**
* @return Specifies whether to use the default server policy.
*
*/
public Optional useServerDefault() {
return Optional.ofNullable(this.useServerDefault);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDatabaseThreatDetectionPolicyResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String disabledAlerts;
private @Nullable String emailAccountAdmins;
private @Nullable String emailAddresses;
private String id;
private String kind;
private @Nullable String location;
private String name;
private @Nullable Integer retentionDays;
private String state;
private @Nullable String storageEndpoint;
private String type;
private @Nullable String useServerDefault;
public Builder() {}
public Builder(GetDatabaseThreatDetectionPolicyResult defaults) {
Objects.requireNonNull(defaults);
this.disabledAlerts = defaults.disabledAlerts;
this.emailAccountAdmins = defaults.emailAccountAdmins;
this.emailAddresses = defaults.emailAddresses;
this.id = defaults.id;
this.kind = defaults.kind;
this.location = defaults.location;
this.name = defaults.name;
this.retentionDays = defaults.retentionDays;
this.state = defaults.state;
this.storageEndpoint = defaults.storageEndpoint;
this.type = defaults.type;
this.useServerDefault = defaults.useServerDefault;
}
@CustomType.Setter
public Builder disabledAlerts(@Nullable String disabledAlerts) {
this.disabledAlerts = disabledAlerts;
return this;
}
@CustomType.Setter
public Builder emailAccountAdmins(@Nullable String emailAccountAdmins) {
this.emailAccountAdmins = emailAccountAdmins;
return this;
}
@CustomType.Setter
public Builder emailAddresses(@Nullable String emailAddresses) {
this.emailAddresses = emailAddresses;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetDatabaseThreatDetectionPolicyResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder kind(String kind) {
if (kind == null) {
throw new MissingRequiredPropertyException("GetDatabaseThreatDetectionPolicyResult", "kind");
}
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder location(@Nullable String location) {
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetDatabaseThreatDetectionPolicyResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder retentionDays(@Nullable Integer retentionDays) {
this.retentionDays = retentionDays;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetDatabaseThreatDetectionPolicyResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder storageEndpoint(@Nullable String storageEndpoint) {
this.storageEndpoint = storageEndpoint;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetDatabaseThreatDetectionPolicyResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder useServerDefault(@Nullable String useServerDefault) {
this.useServerDefault = useServerDefault;
return this;
}
public GetDatabaseThreatDetectionPolicyResult build() {
final var _resultValue = new GetDatabaseThreatDetectionPolicyResult();
_resultValue.disabledAlerts = disabledAlerts;
_resultValue.emailAccountAdmins = emailAccountAdmins;
_resultValue.emailAddresses = emailAddresses;
_resultValue.id = id;
_resultValue.kind = kind;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.retentionDays = retentionDays;
_resultValue.state = state;
_resultValue.storageEndpoint = storageEndpoint;
_resultValue.type = type;
_resultValue.useServerDefault = useServerDefault;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy