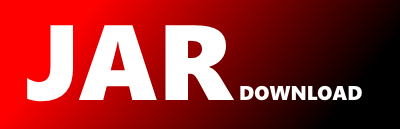
com.pulumi.azurenative.storage.FileShare Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storage;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.storage.FileShareArgs;
import com.pulumi.azurenative.storage.outputs.SignedIdentifierResponse;
import com.pulumi.core.Alias;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Properties of the file share, including Id, resource name, resource type, Etag.
* Azure REST API version: 2022-09-01. Prior API version in Azure Native 1.x: 2021-02-01.
*
* Other available API versions: 2023-01-01, 2023-04-01, 2023-05-01.
*
* ## Example Usage
* ### Create NFS Shares
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.FileShare;
* import com.pulumi.azurenative.storage.FileShareArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var fileShare = new FileShare("fileShare", FileShareArgs.builder()
* .accountName("sto666")
* .enabledProtocols("NFS")
* .resourceGroupName("res346")
* .shareName("share1235")
* .build());
*
* }
* }
*
* }
*
* ### PutShares
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.FileShare;
* import com.pulumi.azurenative.storage.FileShareArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var fileShare = new FileShare("fileShare", FileShareArgs.builder()
* .accountName("sto328")
* .resourceGroupName("res3376")
* .shareName("share6185")
* .build());
*
* }
* }
*
* }
*
* ### PutShares with Access Tier
*
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azurenative.storage.FileShare;
* import com.pulumi.azurenative.storage.FileShareArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var fileShare = new FileShare("fileShare", FileShareArgs.builder()
* .accessTier("Hot")
* .accountName("sto666")
* .resourceGroupName("res346")
* .shareName("share1235")
* .build());
*
* }
* }
*
* }
*
*
* ## Import
*
* An existing resource can be imported using its type token, name, and identifier, e.g.
*
* ```sh
* $ pulumi import azure-native:storage:FileShare share1235 /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/Microsoft.Storage/storageAccounts/{accountName}/fileServices/default/shares/{shareName}
* ```
*
*/
@ResourceType(type="azure-native:storage:FileShare")
public class FileShare extends com.pulumi.resources.CustomResource {
/**
* Access tier for specific share. GpV2 account can choose between TransactionOptimized (default), Hot, and Cool. FileStorage account can choose Premium.
*
*/
@Export(name="accessTier", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> accessTier;
/**
* @return Access tier for specific share. GpV2 account can choose between TransactionOptimized (default), Hot, and Cool. FileStorage account can choose Premium.
*
*/
public Output> accessTier() {
return Codegen.optional(this.accessTier);
}
/**
* Indicates the last modification time for share access tier.
*
*/
@Export(name="accessTierChangeTime", refs={String.class}, tree="[0]")
private Output accessTierChangeTime;
/**
* @return Indicates the last modification time for share access tier.
*
*/
public Output accessTierChangeTime() {
return this.accessTierChangeTime;
}
/**
* Indicates if there is a pending transition for access tier.
*
*/
@Export(name="accessTierStatus", refs={String.class}, tree="[0]")
private Output accessTierStatus;
/**
* @return Indicates if there is a pending transition for access tier.
*
*/
public Output accessTierStatus() {
return this.accessTierStatus;
}
/**
* Indicates whether the share was deleted.
*
*/
@Export(name="deleted", refs={Boolean.class}, tree="[0]")
private Output deleted;
/**
* @return Indicates whether the share was deleted.
*
*/
public Output deleted() {
return this.deleted;
}
/**
* The deleted time if the share was deleted.
*
*/
@Export(name="deletedTime", refs={String.class}, tree="[0]")
private Output deletedTime;
/**
* @return The deleted time if the share was deleted.
*
*/
public Output deletedTime() {
return this.deletedTime;
}
/**
* The authentication protocol that is used for the file share. Can only be specified when creating a share.
*
*/
@Export(name="enabledProtocols", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> enabledProtocols;
/**
* @return The authentication protocol that is used for the file share. Can only be specified when creating a share.
*
*/
public Output> enabledProtocols() {
return Codegen.optional(this.enabledProtocols);
}
/**
* Resource Etag.
*
*/
@Export(name="etag", refs={String.class}, tree="[0]")
private Output etag;
/**
* @return Resource Etag.
*
*/
public Output etag() {
return this.etag;
}
/**
* Returns the date and time the share was last modified.
*
*/
@Export(name="lastModifiedTime", refs={String.class}, tree="[0]")
private Output lastModifiedTime;
/**
* @return Returns the date and time the share was last modified.
*
*/
public Output lastModifiedTime() {
return this.lastModifiedTime;
}
/**
* Specifies whether the lease on a share is of infinite or fixed duration, only when the share is leased.
*
*/
@Export(name="leaseDuration", refs={String.class}, tree="[0]")
private Output leaseDuration;
/**
* @return Specifies whether the lease on a share is of infinite or fixed duration, only when the share is leased.
*
*/
public Output leaseDuration() {
return this.leaseDuration;
}
/**
* Lease state of the share.
*
*/
@Export(name="leaseState", refs={String.class}, tree="[0]")
private Output leaseState;
/**
* @return Lease state of the share.
*
*/
public Output leaseState() {
return this.leaseState;
}
/**
* The lease status of the share.
*
*/
@Export(name="leaseStatus", refs={String.class}, tree="[0]")
private Output leaseStatus;
/**
* @return The lease status of the share.
*
*/
public Output leaseStatus() {
return this.leaseStatus;
}
/**
* A name-value pair to associate with the share as metadata.
*
*/
@Export(name="metadata", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> metadata;
/**
* @return A name-value pair to associate with the share as metadata.
*
*/
public Output>> metadata() {
return Codegen.optional(this.metadata);
}
/**
* The name of the resource
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the resource
*
*/
public Output name() {
return this.name;
}
/**
* Remaining retention days for share that was soft deleted.
*
*/
@Export(name="remainingRetentionDays", refs={Integer.class}, tree="[0]")
private Output remainingRetentionDays;
/**
* @return Remaining retention days for share that was soft deleted.
*
*/
public Output remainingRetentionDays() {
return this.remainingRetentionDays;
}
/**
* The property is for NFS share only. The default is NoRootSquash.
*
*/
@Export(name="rootSquash", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> rootSquash;
/**
* @return The property is for NFS share only. The default is NoRootSquash.
*
*/
public Output> rootSquash() {
return Codegen.optional(this.rootSquash);
}
/**
* The maximum size of the share, in gigabytes. Must be greater than 0, and less than or equal to 5TB (5120). For Large File Shares, the maximum size is 102400.
*
*/
@Export(name="shareQuota", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> shareQuota;
/**
* @return The maximum size of the share, in gigabytes. Must be greater than 0, and less than or equal to 5TB (5120). For Large File Shares, the maximum size is 102400.
*
*/
public Output> shareQuota() {
return Codegen.optional(this.shareQuota);
}
/**
* The approximate size of the data stored on the share. Note that this value may not include all recently created or recently resized files.
*
*/
@Export(name="shareUsageBytes", refs={Double.class}, tree="[0]")
private Output shareUsageBytes;
/**
* @return The approximate size of the data stored on the share. Note that this value may not include all recently created or recently resized files.
*
*/
public Output shareUsageBytes() {
return this.shareUsageBytes;
}
/**
* List of stored access policies specified on the share.
*
*/
@Export(name="signedIdentifiers", refs={List.class,SignedIdentifierResponse.class}, tree="[0,1]")
private Output* @Nullable */ List> signedIdentifiers;
/**
* @return List of stored access policies specified on the share.
*
*/
public Output>> signedIdentifiers() {
return Codegen.optional(this.signedIdentifiers);
}
/**
* Creation time of share snapshot returned in the response of list shares with expand param "snapshots".
*
*/
@Export(name="snapshotTime", refs={String.class}, tree="[0]")
private Output snapshotTime;
/**
* @return Creation time of share snapshot returned in the response of list shares with expand param "snapshots".
*
*/
public Output snapshotTime() {
return this.snapshotTime;
}
/**
* The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
@Export(name="type", refs={String.class}, tree="[0]")
private Output type;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public Output type() {
return this.type;
}
/**
* The version of the share.
*
*/
@Export(name="version", refs={String.class}, tree="[0]")
private Output version;
/**
* @return The version of the share.
*
*/
public Output version() {
return this.version;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public FileShare(java.lang.String name) {
this(name, FileShareArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public FileShare(java.lang.String name, FileShareArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public FileShare(java.lang.String name, FileShareArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:storage:FileShare", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private FileShare(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure-native:storage:FileShare", name, null, makeResourceOptions(options, id), false);
}
private static FileShareArgs makeArgs(FileShareArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? FileShareArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.aliases(List.of(
Output.of(Alias.builder().type("azure-native:storage/v20190401:FileShare").build()),
Output.of(Alias.builder().type("azure-native:storage/v20190601:FileShare").build()),
Output.of(Alias.builder().type("azure-native:storage/v20200801preview:FileShare").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210101:FileShare").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210201:FileShare").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210401:FileShare").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210601:FileShare").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210801:FileShare").build()),
Output.of(Alias.builder().type("azure-native:storage/v20210901:FileShare").build()),
Output.of(Alias.builder().type("azure-native:storage/v20220501:FileShare").build()),
Output.of(Alias.builder().type("azure-native:storage/v20220901:FileShare").build()),
Output.of(Alias.builder().type("azure-native:storage/v20230101:FileShare").build()),
Output.of(Alias.builder().type("azure-native:storage/v20230401:FileShare").build()),
Output.of(Alias.builder().type("azure-native:storage/v20230501:FileShare").build())
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static FileShare get(java.lang.String name, Output id, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new FileShare(name, id, options);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy