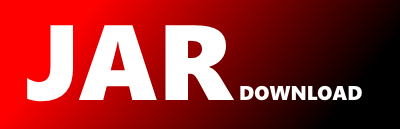
com.pulumi.azurenative.storage.outputs.GetBlobContainerImmutabilityPolicyResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storage.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetBlobContainerImmutabilityPolicyResult {
/**
* @return This property can only be changed for unlocked time-based retention policies. When enabled, new blocks can be written to an append blob while maintaining immutability protection and compliance. Only new blocks can be added and any existing blocks cannot be modified or deleted. This property cannot be changed with ExtendImmutabilityPolicy API.
*
*/
private @Nullable Boolean allowProtectedAppendWrites;
/**
* @return This property can only be changed for unlocked time-based retention policies. When enabled, new blocks can be written to both 'Append and Bock Blobs' while maintaining immutability protection and compliance. Only new blocks can be added and any existing blocks cannot be modified or deleted. This property cannot be changed with ExtendImmutabilityPolicy API. The 'allowProtectedAppendWrites' and 'allowProtectedAppendWritesAll' properties are mutually exclusive.
*
*/
private @Nullable Boolean allowProtectedAppendWritesAll;
/**
* @return Resource Etag.
*
*/
private String etag;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The immutability period for the blobs in the container since the policy creation, in days.
*
*/
private @Nullable Integer immutabilityPeriodSinceCreationInDays;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The ImmutabilityPolicy state of a blob container, possible values include: Locked and Unlocked.
*
*/
private String state;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetBlobContainerImmutabilityPolicyResult() {}
/**
* @return This property can only be changed for unlocked time-based retention policies. When enabled, new blocks can be written to an append blob while maintaining immutability protection and compliance. Only new blocks can be added and any existing blocks cannot be modified or deleted. This property cannot be changed with ExtendImmutabilityPolicy API.
*
*/
public Optional allowProtectedAppendWrites() {
return Optional.ofNullable(this.allowProtectedAppendWrites);
}
/**
* @return This property can only be changed for unlocked time-based retention policies. When enabled, new blocks can be written to both 'Append and Bock Blobs' while maintaining immutability protection and compliance. Only new blocks can be added and any existing blocks cannot be modified or deleted. This property cannot be changed with ExtendImmutabilityPolicy API. The 'allowProtectedAppendWrites' and 'allowProtectedAppendWritesAll' properties are mutually exclusive.
*
*/
public Optional allowProtectedAppendWritesAll() {
return Optional.ofNullable(this.allowProtectedAppendWritesAll);
}
/**
* @return Resource Etag.
*
*/
public String etag() {
return this.etag;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The immutability period for the blobs in the container since the policy creation, in days.
*
*/
public Optional immutabilityPeriodSinceCreationInDays() {
return Optional.ofNullable(this.immutabilityPeriodSinceCreationInDays);
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return The ImmutabilityPolicy state of a blob container, possible values include: Locked and Unlocked.
*
*/
public String state() {
return this.state;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetBlobContainerImmutabilityPolicyResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean allowProtectedAppendWrites;
private @Nullable Boolean allowProtectedAppendWritesAll;
private String etag;
private String id;
private @Nullable Integer immutabilityPeriodSinceCreationInDays;
private String name;
private String state;
private String type;
public Builder() {}
public Builder(GetBlobContainerImmutabilityPolicyResult defaults) {
Objects.requireNonNull(defaults);
this.allowProtectedAppendWrites = defaults.allowProtectedAppendWrites;
this.allowProtectedAppendWritesAll = defaults.allowProtectedAppendWritesAll;
this.etag = defaults.etag;
this.id = defaults.id;
this.immutabilityPeriodSinceCreationInDays = defaults.immutabilityPeriodSinceCreationInDays;
this.name = defaults.name;
this.state = defaults.state;
this.type = defaults.type;
}
@CustomType.Setter
public Builder allowProtectedAppendWrites(@Nullable Boolean allowProtectedAppendWrites) {
this.allowProtectedAppendWrites = allowProtectedAppendWrites;
return this;
}
@CustomType.Setter
public Builder allowProtectedAppendWritesAll(@Nullable Boolean allowProtectedAppendWritesAll) {
this.allowProtectedAppendWritesAll = allowProtectedAppendWritesAll;
return this;
}
@CustomType.Setter
public Builder etag(String etag) {
if (etag == null) {
throw new MissingRequiredPropertyException("GetBlobContainerImmutabilityPolicyResult", "etag");
}
this.etag = etag;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetBlobContainerImmutabilityPolicyResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder immutabilityPeriodSinceCreationInDays(@Nullable Integer immutabilityPeriodSinceCreationInDays) {
this.immutabilityPeriodSinceCreationInDays = immutabilityPeriodSinceCreationInDays;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetBlobContainerImmutabilityPolicyResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetBlobContainerImmutabilityPolicyResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetBlobContainerImmutabilityPolicyResult", "type");
}
this.type = type;
return this;
}
public GetBlobContainerImmutabilityPolicyResult build() {
final var _resultValue = new GetBlobContainerImmutabilityPolicyResult();
_resultValue.allowProtectedAppendWrites = allowProtectedAppendWrites;
_resultValue.allowProtectedAppendWritesAll = allowProtectedAppendWritesAll;
_resultValue.etag = etag;
_resultValue.id = id;
_resultValue.immutabilityPeriodSinceCreationInDays = immutabilityPeriodSinceCreationInDays;
_resultValue.name = name;
_resultValue.state = state;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy