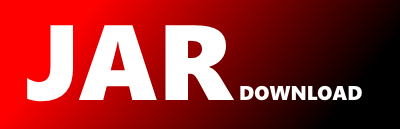
com.pulumi.azurenative.storage.outputs.GetEncryptionScopeResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storage.outputs;
import com.pulumi.azurenative.storage.outputs.EncryptionScopeKeyVaultPropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetEncryptionScopeResult {
/**
* @return Gets the creation date and time of the encryption scope in UTC.
*
*/
private String creationTime;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return The key vault properties for the encryption scope. This is a required field if encryption scope 'source' attribute is set to 'Microsoft.KeyVault'.
*
*/
private @Nullable EncryptionScopeKeyVaultPropertiesResponse keyVaultProperties;
/**
* @return Gets the last modification date and time of the encryption scope in UTC.
*
*/
private String lastModifiedTime;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return A boolean indicating whether or not the service applies a secondary layer of encryption with platform managed keys for data at rest.
*
*/
private @Nullable Boolean requireInfrastructureEncryption;
/**
* @return The provider for the encryption scope. Possible values (case-insensitive): Microsoft.Storage, Microsoft.KeyVault.
*
*/
private @Nullable String source;
/**
* @return The state of the encryption scope. Possible values (case-insensitive): Enabled, Disabled.
*
*/
private @Nullable String state;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetEncryptionScopeResult() {}
/**
* @return Gets the creation date and time of the encryption scope in UTC.
*
*/
public String creationTime() {
return this.creationTime;
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return The key vault properties for the encryption scope. This is a required field if encryption scope 'source' attribute is set to 'Microsoft.KeyVault'.
*
*/
public Optional keyVaultProperties() {
return Optional.ofNullable(this.keyVaultProperties);
}
/**
* @return Gets the last modification date and time of the encryption scope in UTC.
*
*/
public String lastModifiedTime() {
return this.lastModifiedTime;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return A boolean indicating whether or not the service applies a secondary layer of encryption with platform managed keys for data at rest.
*
*/
public Optional requireInfrastructureEncryption() {
return Optional.ofNullable(this.requireInfrastructureEncryption);
}
/**
* @return The provider for the encryption scope. Possible values (case-insensitive): Microsoft.Storage, Microsoft.KeyVault.
*
*/
public Optional source() {
return Optional.ofNullable(this.source);
}
/**
* @return The state of the encryption scope. Possible values (case-insensitive): Enabled, Disabled.
*
*/
public Optional state() {
return Optional.ofNullable(this.state);
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetEncryptionScopeResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String creationTime;
private String id;
private @Nullable EncryptionScopeKeyVaultPropertiesResponse keyVaultProperties;
private String lastModifiedTime;
private String name;
private @Nullable Boolean requireInfrastructureEncryption;
private @Nullable String source;
private @Nullable String state;
private String type;
public Builder() {}
public Builder(GetEncryptionScopeResult defaults) {
Objects.requireNonNull(defaults);
this.creationTime = defaults.creationTime;
this.id = defaults.id;
this.keyVaultProperties = defaults.keyVaultProperties;
this.lastModifiedTime = defaults.lastModifiedTime;
this.name = defaults.name;
this.requireInfrastructureEncryption = defaults.requireInfrastructureEncryption;
this.source = defaults.source;
this.state = defaults.state;
this.type = defaults.type;
}
@CustomType.Setter
public Builder creationTime(String creationTime) {
if (creationTime == null) {
throw new MissingRequiredPropertyException("GetEncryptionScopeResult", "creationTime");
}
this.creationTime = creationTime;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetEncryptionScopeResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder keyVaultProperties(@Nullable EncryptionScopeKeyVaultPropertiesResponse keyVaultProperties) {
this.keyVaultProperties = keyVaultProperties;
return this;
}
@CustomType.Setter
public Builder lastModifiedTime(String lastModifiedTime) {
if (lastModifiedTime == null) {
throw new MissingRequiredPropertyException("GetEncryptionScopeResult", "lastModifiedTime");
}
this.lastModifiedTime = lastModifiedTime;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetEncryptionScopeResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder requireInfrastructureEncryption(@Nullable Boolean requireInfrastructureEncryption) {
this.requireInfrastructureEncryption = requireInfrastructureEncryption;
return this;
}
@CustomType.Setter
public Builder source(@Nullable String source) {
this.source = source;
return this;
}
@CustomType.Setter
public Builder state(@Nullable String state) {
this.state = state;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetEncryptionScopeResult", "type");
}
this.type = type;
return this;
}
public GetEncryptionScopeResult build() {
final var _resultValue = new GetEncryptionScopeResult();
_resultValue.creationTime = creationTime;
_resultValue.id = id;
_resultValue.keyVaultProperties = keyVaultProperties;
_resultValue.lastModifiedTime = lastModifiedTime;
_resultValue.name = name;
_resultValue.requireInfrastructureEncryption = requireInfrastructureEncryption;
_resultValue.source = source;
_resultValue.state = state;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy