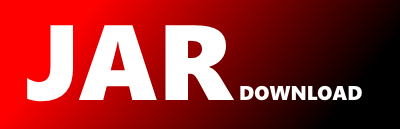
com.pulumi.azurenative.storagecache.outputs.GetImportJobResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.storagecache.outputs;
import com.pulumi.azurenative.storagecache.outputs.SystemDataResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetImportJobResult {
/**
* @return A recent and frequently updated rate of total files, directories, and symlinks imported per second.
*
*/
private Double blobsImportedPerSecond;
/**
* @return A recent and frequently updated rate of blobs walked per second.
*
*/
private Double blobsWalkedPerSecond;
/**
* @return How the import job will handle conflicts. For example, if the import job is trying to bring in a directory, but a file is at that path, how it handles it. Fail indicates that the import job should stop immediately and not do anything with the conflict. Skip indicates that it should pass over the conflict. OverwriteIfDirty causes the import job to delete and re-import the file or directory if it is a conflicting type, is dirty, or was not previously imported. OverwriteAlways extends OverwriteIfDirty to include releasing files that had been restored but were not dirty. Please reference https://learn.microsoft.com/en-us/azure/azure-managed-lustre/ for a thorough explanation of these resolution modes.
*
*/
private @Nullable String conflictResolutionMode;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return An array of blob paths/prefixes that get imported into the cluster namespace. It has '/' as the default value.
*
*/
private @Nullable List importPrefixes;
/**
* @return The time of the last completed archive operation
*
*/
private String lastCompletionTime;
/**
* @return The time the latest archive operation started
*
*/
private String lastStartedTime;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return Total non-conflict oriented errors the import job will tolerate before exiting with failure. -1 means infinite. 0 means exit immediately and is the default.
*
*/
private @Nullable Integer maximumErrors;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return ARM provisioning state.
*
*/
private String provisioningState;
/**
* @return The state of the import job. InProgress indicates the import is still running. Canceled indicates it has been canceled by the user. Completed indicates import finished, successfully importing all discovered blobs into the Lustre namespace. CompletedPartial indicates the import finished but some blobs either were found to be conflicting and could not be imported or other errors were encountered. Failed means the import was unable to complete due to a fatal error.
*
*/
private String state;
/**
* @return The status message of the import job.
*
*/
private String statusMessage;
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
private SystemDataResponse systemData;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The total blobs that have been imported since import began.
*
*/
private Double totalBlobsImported;
/**
* @return The total blob objects walked.
*
*/
private Double totalBlobsWalked;
/**
* @return Number of conflicts in the import job.
*
*/
private Integer totalConflicts;
/**
* @return Number of errors in the import job.
*
*/
private Integer totalErrors;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetImportJobResult() {}
/**
* @return A recent and frequently updated rate of total files, directories, and symlinks imported per second.
*
*/
public Double blobsImportedPerSecond() {
return this.blobsImportedPerSecond;
}
/**
* @return A recent and frequently updated rate of blobs walked per second.
*
*/
public Double blobsWalkedPerSecond() {
return this.blobsWalkedPerSecond;
}
/**
* @return How the import job will handle conflicts. For example, if the import job is trying to bring in a directory, but a file is at that path, how it handles it. Fail indicates that the import job should stop immediately and not do anything with the conflict. Skip indicates that it should pass over the conflict. OverwriteIfDirty causes the import job to delete and re-import the file or directory if it is a conflicting type, is dirty, or was not previously imported. OverwriteAlways extends OverwriteIfDirty to include releasing files that had been restored but were not dirty. Please reference https://learn.microsoft.com/en-us/azure/azure-managed-lustre/ for a thorough explanation of these resolution modes.
*
*/
public Optional conflictResolutionMode() {
return Optional.ofNullable(this.conflictResolutionMode);
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return An array of blob paths/prefixes that get imported into the cluster namespace. It has '/' as the default value.
*
*/
public List importPrefixes() {
return this.importPrefixes == null ? List.of() : this.importPrefixes;
}
/**
* @return The time of the last completed archive operation
*
*/
public String lastCompletionTime() {
return this.lastCompletionTime;
}
/**
* @return The time the latest archive operation started
*
*/
public String lastStartedTime() {
return this.lastStartedTime;
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return Total non-conflict oriented errors the import job will tolerate before exiting with failure. -1 means infinite. 0 means exit immediately and is the default.
*
*/
public Optional maximumErrors() {
return Optional.ofNullable(this.maximumErrors);
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return ARM provisioning state.
*
*/
public String provisioningState() {
return this.provisioningState;
}
/**
* @return The state of the import job. InProgress indicates the import is still running. Canceled indicates it has been canceled by the user. Completed indicates import finished, successfully importing all discovered blobs into the Lustre namespace. CompletedPartial indicates the import finished but some blobs either were found to be conflicting and could not be imported or other errors were encountered. Failed means the import was unable to complete due to a fatal error.
*
*/
public String state() {
return this.state;
}
/**
* @return The status message of the import job.
*
*/
public String statusMessage() {
return this.statusMessage;
}
/**
* @return Azure Resource Manager metadata containing createdBy and modifiedBy information.
*
*/
public SystemDataResponse systemData() {
return this.systemData;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The total blobs that have been imported since import began.
*
*/
public Double totalBlobsImported() {
return this.totalBlobsImported;
}
/**
* @return The total blob objects walked.
*
*/
public Double totalBlobsWalked() {
return this.totalBlobsWalked;
}
/**
* @return Number of conflicts in the import job.
*
*/
public Integer totalConflicts() {
return this.totalConflicts;
}
/**
* @return Number of errors in the import job.
*
*/
public Integer totalErrors() {
return this.totalErrors;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetImportJobResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Double blobsImportedPerSecond;
private Double blobsWalkedPerSecond;
private @Nullable String conflictResolutionMode;
private String id;
private @Nullable List importPrefixes;
private String lastCompletionTime;
private String lastStartedTime;
private String location;
private @Nullable Integer maximumErrors;
private String name;
private String provisioningState;
private String state;
private String statusMessage;
private SystemDataResponse systemData;
private @Nullable Map tags;
private Double totalBlobsImported;
private Double totalBlobsWalked;
private Integer totalConflicts;
private Integer totalErrors;
private String type;
public Builder() {}
public Builder(GetImportJobResult defaults) {
Objects.requireNonNull(defaults);
this.blobsImportedPerSecond = defaults.blobsImportedPerSecond;
this.blobsWalkedPerSecond = defaults.blobsWalkedPerSecond;
this.conflictResolutionMode = defaults.conflictResolutionMode;
this.id = defaults.id;
this.importPrefixes = defaults.importPrefixes;
this.lastCompletionTime = defaults.lastCompletionTime;
this.lastStartedTime = defaults.lastStartedTime;
this.location = defaults.location;
this.maximumErrors = defaults.maximumErrors;
this.name = defaults.name;
this.provisioningState = defaults.provisioningState;
this.state = defaults.state;
this.statusMessage = defaults.statusMessage;
this.systemData = defaults.systemData;
this.tags = defaults.tags;
this.totalBlobsImported = defaults.totalBlobsImported;
this.totalBlobsWalked = defaults.totalBlobsWalked;
this.totalConflicts = defaults.totalConflicts;
this.totalErrors = defaults.totalErrors;
this.type = defaults.type;
}
@CustomType.Setter
public Builder blobsImportedPerSecond(Double blobsImportedPerSecond) {
if (blobsImportedPerSecond == null) {
throw new MissingRequiredPropertyException("GetImportJobResult", "blobsImportedPerSecond");
}
this.blobsImportedPerSecond = blobsImportedPerSecond;
return this;
}
@CustomType.Setter
public Builder blobsWalkedPerSecond(Double blobsWalkedPerSecond) {
if (blobsWalkedPerSecond == null) {
throw new MissingRequiredPropertyException("GetImportJobResult", "blobsWalkedPerSecond");
}
this.blobsWalkedPerSecond = blobsWalkedPerSecond;
return this;
}
@CustomType.Setter
public Builder conflictResolutionMode(@Nullable String conflictResolutionMode) {
this.conflictResolutionMode = conflictResolutionMode;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetImportJobResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder importPrefixes(@Nullable List importPrefixes) {
this.importPrefixes = importPrefixes;
return this;
}
public Builder importPrefixes(String... importPrefixes) {
return importPrefixes(List.of(importPrefixes));
}
@CustomType.Setter
public Builder lastCompletionTime(String lastCompletionTime) {
if (lastCompletionTime == null) {
throw new MissingRequiredPropertyException("GetImportJobResult", "lastCompletionTime");
}
this.lastCompletionTime = lastCompletionTime;
return this;
}
@CustomType.Setter
public Builder lastStartedTime(String lastStartedTime) {
if (lastStartedTime == null) {
throw new MissingRequiredPropertyException("GetImportJobResult", "lastStartedTime");
}
this.lastStartedTime = lastStartedTime;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetImportJobResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder maximumErrors(@Nullable Integer maximumErrors) {
this.maximumErrors = maximumErrors;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetImportJobResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder provisioningState(String provisioningState) {
if (provisioningState == null) {
throw new MissingRequiredPropertyException("GetImportJobResult", "provisioningState");
}
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder state(String state) {
if (state == null) {
throw new MissingRequiredPropertyException("GetImportJobResult", "state");
}
this.state = state;
return this;
}
@CustomType.Setter
public Builder statusMessage(String statusMessage) {
if (statusMessage == null) {
throw new MissingRequiredPropertyException("GetImportJobResult", "statusMessage");
}
this.statusMessage = statusMessage;
return this;
}
@CustomType.Setter
public Builder systemData(SystemDataResponse systemData) {
if (systemData == null) {
throw new MissingRequiredPropertyException("GetImportJobResult", "systemData");
}
this.systemData = systemData;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder totalBlobsImported(Double totalBlobsImported) {
if (totalBlobsImported == null) {
throw new MissingRequiredPropertyException("GetImportJobResult", "totalBlobsImported");
}
this.totalBlobsImported = totalBlobsImported;
return this;
}
@CustomType.Setter
public Builder totalBlobsWalked(Double totalBlobsWalked) {
if (totalBlobsWalked == null) {
throw new MissingRequiredPropertyException("GetImportJobResult", "totalBlobsWalked");
}
this.totalBlobsWalked = totalBlobsWalked;
return this;
}
@CustomType.Setter
public Builder totalConflicts(Integer totalConflicts) {
if (totalConflicts == null) {
throw new MissingRequiredPropertyException("GetImportJobResult", "totalConflicts");
}
this.totalConflicts = totalConflicts;
return this;
}
@CustomType.Setter
public Builder totalErrors(Integer totalErrors) {
if (totalErrors == null) {
throw new MissingRequiredPropertyException("GetImportJobResult", "totalErrors");
}
this.totalErrors = totalErrors;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetImportJobResult", "type");
}
this.type = type;
return this;
}
public GetImportJobResult build() {
final var _resultValue = new GetImportJobResult();
_resultValue.blobsImportedPerSecond = blobsImportedPerSecond;
_resultValue.blobsWalkedPerSecond = blobsWalkedPerSecond;
_resultValue.conflictResolutionMode = conflictResolutionMode;
_resultValue.id = id;
_resultValue.importPrefixes = importPrefixes;
_resultValue.lastCompletionTime = lastCompletionTime;
_resultValue.lastStartedTime = lastStartedTime;
_resultValue.location = location;
_resultValue.maximumErrors = maximumErrors;
_resultValue.name = name;
_resultValue.provisioningState = provisioningState;
_resultValue.state = state;
_resultValue.statusMessage = statusMessage;
_resultValue.systemData = systemData;
_resultValue.tags = tags;
_resultValue.totalBlobsImported = totalBlobsImported;
_resultValue.totalBlobsWalked = totalBlobsWalked;
_resultValue.totalConflicts = totalConflicts;
_resultValue.totalErrors = totalErrors;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy