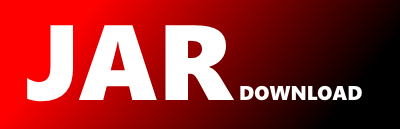
com.pulumi.azurenative.synapse.SqlPoolArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.synapse;
import com.pulumi.azurenative.synapse.enums.CreateMode;
import com.pulumi.azurenative.synapse.enums.StorageAccountType;
import com.pulumi.azurenative.synapse.inputs.SkuArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Double;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class SqlPoolArgs extends com.pulumi.resources.ResourceArgs {
public static final SqlPoolArgs Empty = new SqlPoolArgs();
/**
* Collation mode
*
*/
@Import(name="collation")
private @Nullable Output collation;
/**
* @return Collation mode
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy