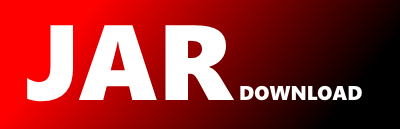
com.pulumi.azurenative.synapse.outputs.GetBigDataPoolResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.synapse.outputs;
import com.pulumi.azurenative.synapse.outputs.AutoPausePropertiesResponse;
import com.pulumi.azurenative.synapse.outputs.AutoScalePropertiesResponse;
import com.pulumi.azurenative.synapse.outputs.DynamicExecutorAllocationResponse;
import com.pulumi.azurenative.synapse.outputs.LibraryInfoResponse;
import com.pulumi.azurenative.synapse.outputs.LibraryRequirementsResponse;
import com.pulumi.azurenative.synapse.outputs.SparkConfigPropertiesResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetBigDataPoolResult {
/**
* @return Auto-pausing properties
*
*/
private @Nullable AutoPausePropertiesResponse autoPause;
/**
* @return Auto-scaling properties
*
*/
private @Nullable AutoScalePropertiesResponse autoScale;
/**
* @return The cache size
*
*/
private @Nullable Integer cacheSize;
/**
* @return The time when the Big Data pool was created.
*
*/
private String creationDate;
/**
* @return List of custom libraries/packages associated with the spark pool.
*
*/
private @Nullable List customLibraries;
/**
* @return The default folder where Spark logs will be written.
*
*/
private @Nullable String defaultSparkLogFolder;
/**
* @return Dynamic Executor Allocation
*
*/
private @Nullable DynamicExecutorAllocationResponse dynamicExecutorAllocation;
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
private String id;
/**
* @return Whether autotune is required or not.
*
*/
private @Nullable Boolean isAutotuneEnabled;
/**
* @return Whether compute isolation is required or not.
*
*/
private @Nullable Boolean isComputeIsolationEnabled;
/**
* @return The time when the Big Data pool was updated successfully.
*
*/
private String lastSucceededTimestamp;
/**
* @return Library version requirements
*
*/
private @Nullable LibraryRequirementsResponse libraryRequirements;
/**
* @return The geo-location where the resource lives
*
*/
private String location;
/**
* @return The name of the resource
*
*/
private String name;
/**
* @return The number of nodes in the Big Data pool.
*
*/
private @Nullable Integer nodeCount;
/**
* @return The level of compute power that each node in the Big Data pool has.
*
*/
private @Nullable String nodeSize;
/**
* @return The kind of nodes that the Big Data pool provides.
*
*/
private @Nullable String nodeSizeFamily;
/**
* @return The state of the Big Data pool.
*
*/
private @Nullable String provisioningState;
/**
* @return Whether session level packages enabled.
*
*/
private @Nullable Boolean sessionLevelPackagesEnabled;
/**
* @return Spark configuration file to specify additional properties
*
*/
private @Nullable SparkConfigPropertiesResponse sparkConfigProperties;
/**
* @return The Spark events folder
*
*/
private @Nullable String sparkEventsFolder;
/**
* @return The Apache Spark version.
*
*/
private @Nullable String sparkVersion;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
private String type;
private GetBigDataPoolResult() {}
/**
* @return Auto-pausing properties
*
*/
public Optional autoPause() {
return Optional.ofNullable(this.autoPause);
}
/**
* @return Auto-scaling properties
*
*/
public Optional autoScale() {
return Optional.ofNullable(this.autoScale);
}
/**
* @return The cache size
*
*/
public Optional cacheSize() {
return Optional.ofNullable(this.cacheSize);
}
/**
* @return The time when the Big Data pool was created.
*
*/
public String creationDate() {
return this.creationDate;
}
/**
* @return List of custom libraries/packages associated with the spark pool.
*
*/
public List customLibraries() {
return this.customLibraries == null ? List.of() : this.customLibraries;
}
/**
* @return The default folder where Spark logs will be written.
*
*/
public Optional defaultSparkLogFolder() {
return Optional.ofNullable(this.defaultSparkLogFolder);
}
/**
* @return Dynamic Executor Allocation
*
*/
public Optional dynamicExecutorAllocation() {
return Optional.ofNullable(this.dynamicExecutorAllocation);
}
/**
* @return Fully qualified resource ID for the resource. Ex - /subscriptions/{subscriptionId}/resourceGroups/{resourceGroupName}/providers/{resourceProviderNamespace}/{resourceType}/{resourceName}
*
*/
public String id() {
return this.id;
}
/**
* @return Whether autotune is required or not.
*
*/
public Optional isAutotuneEnabled() {
return Optional.ofNullable(this.isAutotuneEnabled);
}
/**
* @return Whether compute isolation is required or not.
*
*/
public Optional isComputeIsolationEnabled() {
return Optional.ofNullable(this.isComputeIsolationEnabled);
}
/**
* @return The time when the Big Data pool was updated successfully.
*
*/
public String lastSucceededTimestamp() {
return this.lastSucceededTimestamp;
}
/**
* @return Library version requirements
*
*/
public Optional libraryRequirements() {
return Optional.ofNullable(this.libraryRequirements);
}
/**
* @return The geo-location where the resource lives
*
*/
public String location() {
return this.location;
}
/**
* @return The name of the resource
*
*/
public String name() {
return this.name;
}
/**
* @return The number of nodes in the Big Data pool.
*
*/
public Optional nodeCount() {
return Optional.ofNullable(this.nodeCount);
}
/**
* @return The level of compute power that each node in the Big Data pool has.
*
*/
public Optional nodeSize() {
return Optional.ofNullable(this.nodeSize);
}
/**
* @return The kind of nodes that the Big Data pool provides.
*
*/
public Optional nodeSizeFamily() {
return Optional.ofNullable(this.nodeSizeFamily);
}
/**
* @return The state of the Big Data pool.
*
*/
public Optional provisioningState() {
return Optional.ofNullable(this.provisioningState);
}
/**
* @return Whether session level packages enabled.
*
*/
public Optional sessionLevelPackagesEnabled() {
return Optional.ofNullable(this.sessionLevelPackagesEnabled);
}
/**
* @return Spark configuration file to specify additional properties
*
*/
public Optional sparkConfigProperties() {
return Optional.ofNullable(this.sparkConfigProperties);
}
/**
* @return The Spark events folder
*
*/
public Optional sparkEventsFolder() {
return Optional.ofNullable(this.sparkEventsFolder);
}
/**
* @return The Apache Spark version.
*
*/
public Optional sparkVersion() {
return Optional.ofNullable(this.sparkVersion);
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return The type of the resource. E.g. "Microsoft.Compute/virtualMachines" or "Microsoft.Storage/storageAccounts"
*
*/
public String type() {
return this.type;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetBigDataPoolResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable AutoPausePropertiesResponse autoPause;
private @Nullable AutoScalePropertiesResponse autoScale;
private @Nullable Integer cacheSize;
private String creationDate;
private @Nullable List customLibraries;
private @Nullable String defaultSparkLogFolder;
private @Nullable DynamicExecutorAllocationResponse dynamicExecutorAllocation;
private String id;
private @Nullable Boolean isAutotuneEnabled;
private @Nullable Boolean isComputeIsolationEnabled;
private String lastSucceededTimestamp;
private @Nullable LibraryRequirementsResponse libraryRequirements;
private String location;
private String name;
private @Nullable Integer nodeCount;
private @Nullable String nodeSize;
private @Nullable String nodeSizeFamily;
private @Nullable String provisioningState;
private @Nullable Boolean sessionLevelPackagesEnabled;
private @Nullable SparkConfigPropertiesResponse sparkConfigProperties;
private @Nullable String sparkEventsFolder;
private @Nullable String sparkVersion;
private @Nullable Map tags;
private String type;
public Builder() {}
public Builder(GetBigDataPoolResult defaults) {
Objects.requireNonNull(defaults);
this.autoPause = defaults.autoPause;
this.autoScale = defaults.autoScale;
this.cacheSize = defaults.cacheSize;
this.creationDate = defaults.creationDate;
this.customLibraries = defaults.customLibraries;
this.defaultSparkLogFolder = defaults.defaultSparkLogFolder;
this.dynamicExecutorAllocation = defaults.dynamicExecutorAllocation;
this.id = defaults.id;
this.isAutotuneEnabled = defaults.isAutotuneEnabled;
this.isComputeIsolationEnabled = defaults.isComputeIsolationEnabled;
this.lastSucceededTimestamp = defaults.lastSucceededTimestamp;
this.libraryRequirements = defaults.libraryRequirements;
this.location = defaults.location;
this.name = defaults.name;
this.nodeCount = defaults.nodeCount;
this.nodeSize = defaults.nodeSize;
this.nodeSizeFamily = defaults.nodeSizeFamily;
this.provisioningState = defaults.provisioningState;
this.sessionLevelPackagesEnabled = defaults.sessionLevelPackagesEnabled;
this.sparkConfigProperties = defaults.sparkConfigProperties;
this.sparkEventsFolder = defaults.sparkEventsFolder;
this.sparkVersion = defaults.sparkVersion;
this.tags = defaults.tags;
this.type = defaults.type;
}
@CustomType.Setter
public Builder autoPause(@Nullable AutoPausePropertiesResponse autoPause) {
this.autoPause = autoPause;
return this;
}
@CustomType.Setter
public Builder autoScale(@Nullable AutoScalePropertiesResponse autoScale) {
this.autoScale = autoScale;
return this;
}
@CustomType.Setter
public Builder cacheSize(@Nullable Integer cacheSize) {
this.cacheSize = cacheSize;
return this;
}
@CustomType.Setter
public Builder creationDate(String creationDate) {
if (creationDate == null) {
throw new MissingRequiredPropertyException("GetBigDataPoolResult", "creationDate");
}
this.creationDate = creationDate;
return this;
}
@CustomType.Setter
public Builder customLibraries(@Nullable List customLibraries) {
this.customLibraries = customLibraries;
return this;
}
public Builder customLibraries(LibraryInfoResponse... customLibraries) {
return customLibraries(List.of(customLibraries));
}
@CustomType.Setter
public Builder defaultSparkLogFolder(@Nullable String defaultSparkLogFolder) {
this.defaultSparkLogFolder = defaultSparkLogFolder;
return this;
}
@CustomType.Setter
public Builder dynamicExecutorAllocation(@Nullable DynamicExecutorAllocationResponse dynamicExecutorAllocation) {
this.dynamicExecutorAllocation = dynamicExecutorAllocation;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetBigDataPoolResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder isAutotuneEnabled(@Nullable Boolean isAutotuneEnabled) {
this.isAutotuneEnabled = isAutotuneEnabled;
return this;
}
@CustomType.Setter
public Builder isComputeIsolationEnabled(@Nullable Boolean isComputeIsolationEnabled) {
this.isComputeIsolationEnabled = isComputeIsolationEnabled;
return this;
}
@CustomType.Setter
public Builder lastSucceededTimestamp(String lastSucceededTimestamp) {
if (lastSucceededTimestamp == null) {
throw new MissingRequiredPropertyException("GetBigDataPoolResult", "lastSucceededTimestamp");
}
this.lastSucceededTimestamp = lastSucceededTimestamp;
return this;
}
@CustomType.Setter
public Builder libraryRequirements(@Nullable LibraryRequirementsResponse libraryRequirements) {
this.libraryRequirements = libraryRequirements;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetBigDataPoolResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetBigDataPoolResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder nodeCount(@Nullable Integer nodeCount) {
this.nodeCount = nodeCount;
return this;
}
@CustomType.Setter
public Builder nodeSize(@Nullable String nodeSize) {
this.nodeSize = nodeSize;
return this;
}
@CustomType.Setter
public Builder nodeSizeFamily(@Nullable String nodeSizeFamily) {
this.nodeSizeFamily = nodeSizeFamily;
return this;
}
@CustomType.Setter
public Builder provisioningState(@Nullable String provisioningState) {
this.provisioningState = provisioningState;
return this;
}
@CustomType.Setter
public Builder sessionLevelPackagesEnabled(@Nullable Boolean sessionLevelPackagesEnabled) {
this.sessionLevelPackagesEnabled = sessionLevelPackagesEnabled;
return this;
}
@CustomType.Setter
public Builder sparkConfigProperties(@Nullable SparkConfigPropertiesResponse sparkConfigProperties) {
this.sparkConfigProperties = sparkConfigProperties;
return this;
}
@CustomType.Setter
public Builder sparkEventsFolder(@Nullable String sparkEventsFolder) {
this.sparkEventsFolder = sparkEventsFolder;
return this;
}
@CustomType.Setter
public Builder sparkVersion(@Nullable String sparkVersion) {
this.sparkVersion = sparkVersion;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetBigDataPoolResult", "type");
}
this.type = type;
return this;
}
public GetBigDataPoolResult build() {
final var _resultValue = new GetBigDataPoolResult();
_resultValue.autoPause = autoPause;
_resultValue.autoScale = autoScale;
_resultValue.cacheSize = cacheSize;
_resultValue.creationDate = creationDate;
_resultValue.customLibraries = customLibraries;
_resultValue.defaultSparkLogFolder = defaultSparkLogFolder;
_resultValue.dynamicExecutorAllocation = dynamicExecutorAllocation;
_resultValue.id = id;
_resultValue.isAutotuneEnabled = isAutotuneEnabled;
_resultValue.isComputeIsolationEnabled = isComputeIsolationEnabled;
_resultValue.lastSucceededTimestamp = lastSucceededTimestamp;
_resultValue.libraryRequirements = libraryRequirements;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.nodeCount = nodeCount;
_resultValue.nodeSize = nodeSize;
_resultValue.nodeSizeFamily = nodeSizeFamily;
_resultValue.provisioningState = provisioningState;
_resultValue.sessionLevelPackagesEnabled = sessionLevelPackagesEnabled;
_resultValue.sparkConfigProperties = sparkConfigProperties;
_resultValue.sparkEventsFolder = sparkEventsFolder;
_resultValue.sparkVersion = sparkVersion;
_resultValue.tags = tags;
_resultValue.type = type;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy