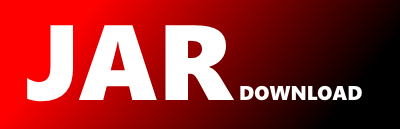
com.pulumi.azurenative.testbase.DraftPackageArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.testbase;
import com.pulumi.azurenative.testbase.enums.DraftPackageSourceType;
import com.pulumi.azurenative.testbase.enums.TestType;
import com.pulumi.azurenative.testbase.inputs.DraftPackageIntuneAppMetadataArgs;
import com.pulumi.azurenative.testbase.inputs.FirstPartyAppDefinitionArgs;
import com.pulumi.azurenative.testbase.inputs.GalleryAppDefinitionArgs;
import com.pulumi.azurenative.testbase.inputs.HighlightedFileArgs;
import com.pulumi.azurenative.testbase.inputs.InplaceUpgradeOSInfoArgs;
import com.pulumi.azurenative.testbase.inputs.IntuneEnrollmentMetadataArgs;
import com.pulumi.azurenative.testbase.inputs.TabStateArgs;
import com.pulumi.azurenative.testbase.inputs.TargetOSInfoArgs;
import com.pulumi.azurenative.testbase.inputs.TestArgs;
import com.pulumi.core.Either;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class DraftPackageArgs extends com.pulumi.resources.ResourceArgs {
public static final DraftPackageArgs Empty = new DraftPackageArgs();
/**
* The name of the app file.
*
*/
@Import(name="appFileName")
private @Nullable Output appFileName;
/**
* @return The name of the app file.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy