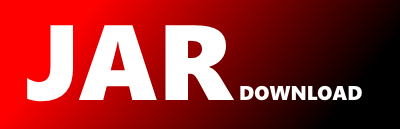
com.pulumi.azurenative.testbase.outputs.CommandResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.testbase.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class CommandResponse {
/**
* @return The action of the command.
*
*/
private String action;
/**
* @return Specifies whether to run the command even if a previous command is failed.
*
*/
private @Nullable Boolean alwaysRun;
/**
* @return Specifies whether to apply update before the command.
*
*/
private @Nullable Boolean applyUpdateBefore;
/**
* @return The content of the command. The content depends on source type.
*
*/
private String content;
/**
* @return The type of command content.
*
*/
private String contentType;
/**
* @return Specifies whether to enroll Intune before the command.
*
*/
private @Nullable Boolean enrollIntuneBefore;
/**
* @return Specifies whether to install first party applications before running the command.
*
*/
private @Nullable Boolean install1PAppBefore;
/**
* @return Specifies the max run time of the command.
*
*/
private @Nullable Integer maxRunTime;
/**
* @return The name of the command.
*
*/
private String name;
/**
* @return Specifies whether the command is assigned to be executed after in-place upgrade.
*
*/
private @Nullable Boolean postUpgrade;
/**
* @return Specifies whether the command is assigned to be executed before in-place upgrade.
*
*/
private @Nullable Boolean preUpgrade;
/**
* @return Specifies whether to restart the VM after the command executed.
*
*/
private @Nullable Boolean restartAfter;
/**
* @return Specifies whether to run the command in interactive mode.
*
*/
private @Nullable Boolean runAsInteractive;
/**
* @return Specifies whether to run the command as administrator.
*
*/
private @Nullable Boolean runElevated;
private CommandResponse() {}
/**
* @return The action of the command.
*
*/
public String action() {
return this.action;
}
/**
* @return Specifies whether to run the command even if a previous command is failed.
*
*/
public Optional alwaysRun() {
return Optional.ofNullable(this.alwaysRun);
}
/**
* @return Specifies whether to apply update before the command.
*
*/
public Optional applyUpdateBefore() {
return Optional.ofNullable(this.applyUpdateBefore);
}
/**
* @return The content of the command. The content depends on source type.
*
*/
public String content() {
return this.content;
}
/**
* @return The type of command content.
*
*/
public String contentType() {
return this.contentType;
}
/**
* @return Specifies whether to enroll Intune before the command.
*
*/
public Optional enrollIntuneBefore() {
return Optional.ofNullable(this.enrollIntuneBefore);
}
/**
* @return Specifies whether to install first party applications before running the command.
*
*/
public Optional install1PAppBefore() {
return Optional.ofNullable(this.install1PAppBefore);
}
/**
* @return Specifies the max run time of the command.
*
*/
public Optional maxRunTime() {
return Optional.ofNullable(this.maxRunTime);
}
/**
* @return The name of the command.
*
*/
public String name() {
return this.name;
}
/**
* @return Specifies whether the command is assigned to be executed after in-place upgrade.
*
*/
public Optional postUpgrade() {
return Optional.ofNullable(this.postUpgrade);
}
/**
* @return Specifies whether the command is assigned to be executed before in-place upgrade.
*
*/
public Optional preUpgrade() {
return Optional.ofNullable(this.preUpgrade);
}
/**
* @return Specifies whether to restart the VM after the command executed.
*
*/
public Optional restartAfter() {
return Optional.ofNullable(this.restartAfter);
}
/**
* @return Specifies whether to run the command in interactive mode.
*
*/
public Optional runAsInteractive() {
return Optional.ofNullable(this.runAsInteractive);
}
/**
* @return Specifies whether to run the command as administrator.
*
*/
public Optional runElevated() {
return Optional.ofNullable(this.runElevated);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(CommandResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String action;
private @Nullable Boolean alwaysRun;
private @Nullable Boolean applyUpdateBefore;
private String content;
private String contentType;
private @Nullable Boolean enrollIntuneBefore;
private @Nullable Boolean install1PAppBefore;
private @Nullable Integer maxRunTime;
private String name;
private @Nullable Boolean postUpgrade;
private @Nullable Boolean preUpgrade;
private @Nullable Boolean restartAfter;
private @Nullable Boolean runAsInteractive;
private @Nullable Boolean runElevated;
public Builder() {}
public Builder(CommandResponse defaults) {
Objects.requireNonNull(defaults);
this.action = defaults.action;
this.alwaysRun = defaults.alwaysRun;
this.applyUpdateBefore = defaults.applyUpdateBefore;
this.content = defaults.content;
this.contentType = defaults.contentType;
this.enrollIntuneBefore = defaults.enrollIntuneBefore;
this.install1PAppBefore = defaults.install1PAppBefore;
this.maxRunTime = defaults.maxRunTime;
this.name = defaults.name;
this.postUpgrade = defaults.postUpgrade;
this.preUpgrade = defaults.preUpgrade;
this.restartAfter = defaults.restartAfter;
this.runAsInteractive = defaults.runAsInteractive;
this.runElevated = defaults.runElevated;
}
@CustomType.Setter
public Builder action(String action) {
if (action == null) {
throw new MissingRequiredPropertyException("CommandResponse", "action");
}
this.action = action;
return this;
}
@CustomType.Setter
public Builder alwaysRun(@Nullable Boolean alwaysRun) {
this.alwaysRun = alwaysRun;
return this;
}
@CustomType.Setter
public Builder applyUpdateBefore(@Nullable Boolean applyUpdateBefore) {
this.applyUpdateBefore = applyUpdateBefore;
return this;
}
@CustomType.Setter
public Builder content(String content) {
if (content == null) {
throw new MissingRequiredPropertyException("CommandResponse", "content");
}
this.content = content;
return this;
}
@CustomType.Setter
public Builder contentType(String contentType) {
if (contentType == null) {
throw new MissingRequiredPropertyException("CommandResponse", "contentType");
}
this.contentType = contentType;
return this;
}
@CustomType.Setter
public Builder enrollIntuneBefore(@Nullable Boolean enrollIntuneBefore) {
this.enrollIntuneBefore = enrollIntuneBefore;
return this;
}
@CustomType.Setter
public Builder install1PAppBefore(@Nullable Boolean install1PAppBefore) {
this.install1PAppBefore = install1PAppBefore;
return this;
}
@CustomType.Setter
public Builder maxRunTime(@Nullable Integer maxRunTime) {
this.maxRunTime = maxRunTime;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("CommandResponse", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder postUpgrade(@Nullable Boolean postUpgrade) {
this.postUpgrade = postUpgrade;
return this;
}
@CustomType.Setter
public Builder preUpgrade(@Nullable Boolean preUpgrade) {
this.preUpgrade = preUpgrade;
return this;
}
@CustomType.Setter
public Builder restartAfter(@Nullable Boolean restartAfter) {
this.restartAfter = restartAfter;
return this;
}
@CustomType.Setter
public Builder runAsInteractive(@Nullable Boolean runAsInteractive) {
this.runAsInteractive = runAsInteractive;
return this;
}
@CustomType.Setter
public Builder runElevated(@Nullable Boolean runElevated) {
this.runElevated = runElevated;
return this;
}
public CommandResponse build() {
final var _resultValue = new CommandResponse();
_resultValue.action = action;
_resultValue.alwaysRun = alwaysRun;
_resultValue.applyUpdateBefore = applyUpdateBefore;
_resultValue.content = content;
_resultValue.contentType = contentType;
_resultValue.enrollIntuneBefore = enrollIntuneBefore;
_resultValue.install1PAppBefore = install1PAppBefore;
_resultValue.maxRunTime = maxRunTime;
_resultValue.name = name;
_resultValue.postUpgrade = postUpgrade;
_resultValue.preUpgrade = preUpgrade;
_resultValue.restartAfter = restartAfter;
_resultValue.runAsInteractive = runAsInteractive;
_resultValue.runElevated = runElevated;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy