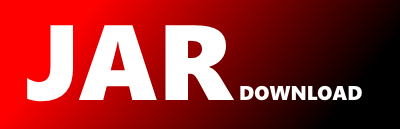
com.pulumi.azurenative.videoanalyzer.inputs.VideoSinkArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.videoanalyzer.inputs;
import com.pulumi.azurenative.videoanalyzer.inputs.NodeInputArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.VideoCreationPropertiesArgs;
import com.pulumi.azurenative.videoanalyzer.inputs.VideoPublishingOptionsArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Video sink in a live topology allows for video and audio to be captured, optionally archived, and published via a video resource. If archiving is enabled, this results in a video of type 'archive'. If used in a batch topology, this allows for video and audio to be stored as a file, and published via a video resource of type 'file'
*
*/
public final class VideoSinkArgs extends com.pulumi.resources.ResourceArgs {
public static final VideoSinkArgs Empty = new VideoSinkArgs();
/**
* An array of upstream node references within the topology to be used as inputs for this node.
*
*/
@Import(name="inputs", required=true)
private Output> inputs;
/**
* @return An array of upstream node references within the topology to be used as inputs for this node.
*
*/
public Output> inputs() {
return this.inputs;
}
/**
* Node name. Must be unique within the topology.
*
*/
@Import(name="name", required=true)
private Output name;
/**
* @return Node name. Must be unique within the topology.
*
*/
public Output name() {
return this.name;
}
/**
* The discriminator for derived types.
* Expected value is '#Microsoft.VideoAnalyzer.VideoSink'.
*
*/
@Import(name="type", required=true)
private Output type;
/**
* @return The discriminator for derived types.
* Expected value is '#Microsoft.VideoAnalyzer.VideoSink'.
*
*/
public Output type() {
return this.type;
}
/**
* Optional video properties to be used in case a new video resource needs to be created on the service.
*
*/
@Import(name="videoCreationProperties")
private @Nullable Output videoCreationProperties;
/**
* @return Optional video properties to be used in case a new video resource needs to be created on the service.
*
*/
public Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy