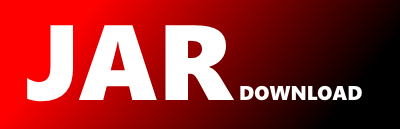
com.pulumi.azurenative.web.outputs.GetCertificateResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.web.outputs;
import com.pulumi.azurenative.web.outputs.HostingEnvironmentProfileResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetCertificateResult {
/**
* @return CNAME of the certificate to be issued via free certificate
*
*/
private @Nullable String canonicalName;
/**
* @return Raw bytes of .cer file
*
*/
private String cerBlob;
/**
* @return Method of domain validation for free cert
*
*/
private @Nullable String domainValidationMethod;
/**
* @return Certificate expiration date.
*
*/
private String expirationDate;
/**
* @return Friendly name of the certificate.
*
*/
private String friendlyName;
/**
* @return Host names the certificate applies to.
*
*/
private @Nullable List hostNames;
/**
* @return Specification for the App Service Environment to use for the certificate.
*
*/
private HostingEnvironmentProfileResponse hostingEnvironmentProfile;
/**
* @return Resource Id.
*
*/
private String id;
/**
* @return Certificate issue Date.
*
*/
private String issueDate;
/**
* @return Certificate issuer.
*
*/
private String issuer;
/**
* @return Key Vault Csm resource Id.
*
*/
private @Nullable String keyVaultId;
/**
* @return Key Vault secret name.
*
*/
private @Nullable String keyVaultSecretName;
/**
* @return Status of the Key Vault secret.
*
*/
private String keyVaultSecretStatus;
/**
* @return Kind of resource.
*
*/
private @Nullable String kind;
/**
* @return Resource Location.
*
*/
private String location;
/**
* @return Resource Name.
*
*/
private String name;
/**
* @return Pfx blob.
*
*/
private @Nullable String pfxBlob;
/**
* @return Public key hash.
*
*/
private String publicKeyHash;
/**
* @return Self link.
*
*/
private String selfLink;
/**
* @return Resource ID of the associated App Service plan, formatted as: "/subscriptions/{subscriptionID}/resourceGroups/{groupName}/providers/Microsoft.Web/serverfarms/{appServicePlanName}".
*
*/
private @Nullable String serverFarmId;
/**
* @return App name.
*
*/
private String siteName;
/**
* @return Subject name of the certificate.
*
*/
private String subjectName;
/**
* @return Resource tags.
*
*/
private @Nullable Map tags;
/**
* @return Certificate thumbprint.
*
*/
private String thumbprint;
/**
* @return Resource type.
*
*/
private String type;
/**
* @return Is the certificate valid?.
*
*/
private Boolean valid;
private GetCertificateResult() {}
/**
* @return CNAME of the certificate to be issued via free certificate
*
*/
public Optional canonicalName() {
return Optional.ofNullable(this.canonicalName);
}
/**
* @return Raw bytes of .cer file
*
*/
public String cerBlob() {
return this.cerBlob;
}
/**
* @return Method of domain validation for free cert
*
*/
public Optional domainValidationMethod() {
return Optional.ofNullable(this.domainValidationMethod);
}
/**
* @return Certificate expiration date.
*
*/
public String expirationDate() {
return this.expirationDate;
}
/**
* @return Friendly name of the certificate.
*
*/
public String friendlyName() {
return this.friendlyName;
}
/**
* @return Host names the certificate applies to.
*
*/
public List hostNames() {
return this.hostNames == null ? List.of() : this.hostNames;
}
/**
* @return Specification for the App Service Environment to use for the certificate.
*
*/
public HostingEnvironmentProfileResponse hostingEnvironmentProfile() {
return this.hostingEnvironmentProfile;
}
/**
* @return Resource Id.
*
*/
public String id() {
return this.id;
}
/**
* @return Certificate issue Date.
*
*/
public String issueDate() {
return this.issueDate;
}
/**
* @return Certificate issuer.
*
*/
public String issuer() {
return this.issuer;
}
/**
* @return Key Vault Csm resource Id.
*
*/
public Optional keyVaultId() {
return Optional.ofNullable(this.keyVaultId);
}
/**
* @return Key Vault secret name.
*
*/
public Optional keyVaultSecretName() {
return Optional.ofNullable(this.keyVaultSecretName);
}
/**
* @return Status of the Key Vault secret.
*
*/
public String keyVaultSecretStatus() {
return this.keyVaultSecretStatus;
}
/**
* @return Kind of resource.
*
*/
public Optional kind() {
return Optional.ofNullable(this.kind);
}
/**
* @return Resource Location.
*
*/
public String location() {
return this.location;
}
/**
* @return Resource Name.
*
*/
public String name() {
return this.name;
}
/**
* @return Pfx blob.
*
*/
public Optional pfxBlob() {
return Optional.ofNullable(this.pfxBlob);
}
/**
* @return Public key hash.
*
*/
public String publicKeyHash() {
return this.publicKeyHash;
}
/**
* @return Self link.
*
*/
public String selfLink() {
return this.selfLink;
}
/**
* @return Resource ID of the associated App Service plan, formatted as: "/subscriptions/{subscriptionID}/resourceGroups/{groupName}/providers/Microsoft.Web/serverfarms/{appServicePlanName}".
*
*/
public Optional serverFarmId() {
return Optional.ofNullable(this.serverFarmId);
}
/**
* @return App name.
*
*/
public String siteName() {
return this.siteName;
}
/**
* @return Subject name of the certificate.
*
*/
public String subjectName() {
return this.subjectName;
}
/**
* @return Resource tags.
*
*/
public Map tags() {
return this.tags == null ? Map.of() : this.tags;
}
/**
* @return Certificate thumbprint.
*
*/
public String thumbprint() {
return this.thumbprint;
}
/**
* @return Resource type.
*
*/
public String type() {
return this.type;
}
/**
* @return Is the certificate valid?.
*
*/
public Boolean valid() {
return this.valid;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetCertificateResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String canonicalName;
private String cerBlob;
private @Nullable String domainValidationMethod;
private String expirationDate;
private String friendlyName;
private @Nullable List hostNames;
private HostingEnvironmentProfileResponse hostingEnvironmentProfile;
private String id;
private String issueDate;
private String issuer;
private @Nullable String keyVaultId;
private @Nullable String keyVaultSecretName;
private String keyVaultSecretStatus;
private @Nullable String kind;
private String location;
private String name;
private @Nullable String pfxBlob;
private String publicKeyHash;
private String selfLink;
private @Nullable String serverFarmId;
private String siteName;
private String subjectName;
private @Nullable Map tags;
private String thumbprint;
private String type;
private Boolean valid;
public Builder() {}
public Builder(GetCertificateResult defaults) {
Objects.requireNonNull(defaults);
this.canonicalName = defaults.canonicalName;
this.cerBlob = defaults.cerBlob;
this.domainValidationMethod = defaults.domainValidationMethod;
this.expirationDate = defaults.expirationDate;
this.friendlyName = defaults.friendlyName;
this.hostNames = defaults.hostNames;
this.hostingEnvironmentProfile = defaults.hostingEnvironmentProfile;
this.id = defaults.id;
this.issueDate = defaults.issueDate;
this.issuer = defaults.issuer;
this.keyVaultId = defaults.keyVaultId;
this.keyVaultSecretName = defaults.keyVaultSecretName;
this.keyVaultSecretStatus = defaults.keyVaultSecretStatus;
this.kind = defaults.kind;
this.location = defaults.location;
this.name = defaults.name;
this.pfxBlob = defaults.pfxBlob;
this.publicKeyHash = defaults.publicKeyHash;
this.selfLink = defaults.selfLink;
this.serverFarmId = defaults.serverFarmId;
this.siteName = defaults.siteName;
this.subjectName = defaults.subjectName;
this.tags = defaults.tags;
this.thumbprint = defaults.thumbprint;
this.type = defaults.type;
this.valid = defaults.valid;
}
@CustomType.Setter
public Builder canonicalName(@Nullable String canonicalName) {
this.canonicalName = canonicalName;
return this;
}
@CustomType.Setter
public Builder cerBlob(String cerBlob) {
if (cerBlob == null) {
throw new MissingRequiredPropertyException("GetCertificateResult", "cerBlob");
}
this.cerBlob = cerBlob;
return this;
}
@CustomType.Setter
public Builder domainValidationMethod(@Nullable String domainValidationMethod) {
this.domainValidationMethod = domainValidationMethod;
return this;
}
@CustomType.Setter
public Builder expirationDate(String expirationDate) {
if (expirationDate == null) {
throw new MissingRequiredPropertyException("GetCertificateResult", "expirationDate");
}
this.expirationDate = expirationDate;
return this;
}
@CustomType.Setter
public Builder friendlyName(String friendlyName) {
if (friendlyName == null) {
throw new MissingRequiredPropertyException("GetCertificateResult", "friendlyName");
}
this.friendlyName = friendlyName;
return this;
}
@CustomType.Setter
public Builder hostNames(@Nullable List hostNames) {
this.hostNames = hostNames;
return this;
}
public Builder hostNames(String... hostNames) {
return hostNames(List.of(hostNames));
}
@CustomType.Setter
public Builder hostingEnvironmentProfile(HostingEnvironmentProfileResponse hostingEnvironmentProfile) {
if (hostingEnvironmentProfile == null) {
throw new MissingRequiredPropertyException("GetCertificateResult", "hostingEnvironmentProfile");
}
this.hostingEnvironmentProfile = hostingEnvironmentProfile;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetCertificateResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder issueDate(String issueDate) {
if (issueDate == null) {
throw new MissingRequiredPropertyException("GetCertificateResult", "issueDate");
}
this.issueDate = issueDate;
return this;
}
@CustomType.Setter
public Builder issuer(String issuer) {
if (issuer == null) {
throw new MissingRequiredPropertyException("GetCertificateResult", "issuer");
}
this.issuer = issuer;
return this;
}
@CustomType.Setter
public Builder keyVaultId(@Nullable String keyVaultId) {
this.keyVaultId = keyVaultId;
return this;
}
@CustomType.Setter
public Builder keyVaultSecretName(@Nullable String keyVaultSecretName) {
this.keyVaultSecretName = keyVaultSecretName;
return this;
}
@CustomType.Setter
public Builder keyVaultSecretStatus(String keyVaultSecretStatus) {
if (keyVaultSecretStatus == null) {
throw new MissingRequiredPropertyException("GetCertificateResult", "keyVaultSecretStatus");
}
this.keyVaultSecretStatus = keyVaultSecretStatus;
return this;
}
@CustomType.Setter
public Builder kind(@Nullable String kind) {
this.kind = kind;
return this;
}
@CustomType.Setter
public Builder location(String location) {
if (location == null) {
throw new MissingRequiredPropertyException("GetCertificateResult", "location");
}
this.location = location;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetCertificateResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder pfxBlob(@Nullable String pfxBlob) {
this.pfxBlob = pfxBlob;
return this;
}
@CustomType.Setter
public Builder publicKeyHash(String publicKeyHash) {
if (publicKeyHash == null) {
throw new MissingRequiredPropertyException("GetCertificateResult", "publicKeyHash");
}
this.publicKeyHash = publicKeyHash;
return this;
}
@CustomType.Setter
public Builder selfLink(String selfLink) {
if (selfLink == null) {
throw new MissingRequiredPropertyException("GetCertificateResult", "selfLink");
}
this.selfLink = selfLink;
return this;
}
@CustomType.Setter
public Builder serverFarmId(@Nullable String serverFarmId) {
this.serverFarmId = serverFarmId;
return this;
}
@CustomType.Setter
public Builder siteName(String siteName) {
if (siteName == null) {
throw new MissingRequiredPropertyException("GetCertificateResult", "siteName");
}
this.siteName = siteName;
return this;
}
@CustomType.Setter
public Builder subjectName(String subjectName) {
if (subjectName == null) {
throw new MissingRequiredPropertyException("GetCertificateResult", "subjectName");
}
this.subjectName = subjectName;
return this;
}
@CustomType.Setter
public Builder tags(@Nullable Map tags) {
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder thumbprint(String thumbprint) {
if (thumbprint == null) {
throw new MissingRequiredPropertyException("GetCertificateResult", "thumbprint");
}
this.thumbprint = thumbprint;
return this;
}
@CustomType.Setter
public Builder type(String type) {
if (type == null) {
throw new MissingRequiredPropertyException("GetCertificateResult", "type");
}
this.type = type;
return this;
}
@CustomType.Setter
public Builder valid(Boolean valid) {
if (valid == null) {
throw new MissingRequiredPropertyException("GetCertificateResult", "valid");
}
this.valid = valid;
return this;
}
public GetCertificateResult build() {
final var _resultValue = new GetCertificateResult();
_resultValue.canonicalName = canonicalName;
_resultValue.cerBlob = cerBlob;
_resultValue.domainValidationMethod = domainValidationMethod;
_resultValue.expirationDate = expirationDate;
_resultValue.friendlyName = friendlyName;
_resultValue.hostNames = hostNames;
_resultValue.hostingEnvironmentProfile = hostingEnvironmentProfile;
_resultValue.id = id;
_resultValue.issueDate = issueDate;
_resultValue.issuer = issuer;
_resultValue.keyVaultId = keyVaultId;
_resultValue.keyVaultSecretName = keyVaultSecretName;
_resultValue.keyVaultSecretStatus = keyVaultSecretStatus;
_resultValue.kind = kind;
_resultValue.location = location;
_resultValue.name = name;
_resultValue.pfxBlob = pfxBlob;
_resultValue.publicKeyHash = publicKeyHash;
_resultValue.selfLink = selfLink;
_resultValue.serverFarmId = serverFarmId;
_resultValue.siteName = siteName;
_resultValue.subjectName = subjectName;
_resultValue.tags = tags;
_resultValue.thumbprint = thumbprint;
_resultValue.type = type;
_resultValue.valid = valid;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy