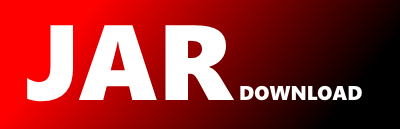
com.pulumi.azurenative.webpubsub.WebpubsubFunctions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.webpubsub;
import com.pulumi.azurenative.Utilities;
import com.pulumi.azurenative.webpubsub.inputs.GetWebPubSubArgs;
import com.pulumi.azurenative.webpubsub.inputs.GetWebPubSubCustomCertificateArgs;
import com.pulumi.azurenative.webpubsub.inputs.GetWebPubSubCustomCertificatePlainArgs;
import com.pulumi.azurenative.webpubsub.inputs.GetWebPubSubCustomDomainArgs;
import com.pulumi.azurenative.webpubsub.inputs.GetWebPubSubCustomDomainPlainArgs;
import com.pulumi.azurenative.webpubsub.inputs.GetWebPubSubHubArgs;
import com.pulumi.azurenative.webpubsub.inputs.GetWebPubSubHubPlainArgs;
import com.pulumi.azurenative.webpubsub.inputs.GetWebPubSubPlainArgs;
import com.pulumi.azurenative.webpubsub.inputs.GetWebPubSubPrivateEndpointConnectionArgs;
import com.pulumi.azurenative.webpubsub.inputs.GetWebPubSubPrivateEndpointConnectionPlainArgs;
import com.pulumi.azurenative.webpubsub.inputs.GetWebPubSubReplicaArgs;
import com.pulumi.azurenative.webpubsub.inputs.GetWebPubSubReplicaPlainArgs;
import com.pulumi.azurenative.webpubsub.inputs.GetWebPubSubSharedPrivateLinkResourceArgs;
import com.pulumi.azurenative.webpubsub.inputs.GetWebPubSubSharedPrivateLinkResourcePlainArgs;
import com.pulumi.azurenative.webpubsub.inputs.ListWebPubSubKeysArgs;
import com.pulumi.azurenative.webpubsub.inputs.ListWebPubSubKeysPlainArgs;
import com.pulumi.azurenative.webpubsub.outputs.GetWebPubSubCustomCertificateResult;
import com.pulumi.azurenative.webpubsub.outputs.GetWebPubSubCustomDomainResult;
import com.pulumi.azurenative.webpubsub.outputs.GetWebPubSubHubResult;
import com.pulumi.azurenative.webpubsub.outputs.GetWebPubSubPrivateEndpointConnectionResult;
import com.pulumi.azurenative.webpubsub.outputs.GetWebPubSubReplicaResult;
import com.pulumi.azurenative.webpubsub.outputs.GetWebPubSubResult;
import com.pulumi.azurenative.webpubsub.outputs.GetWebPubSubSharedPrivateLinkResourceResult;
import com.pulumi.azurenative.webpubsub.outputs.ListWebPubSubKeysResult;
import com.pulumi.core.Output;
import com.pulumi.core.TypeShape;
import com.pulumi.deployment.Deployment;
import com.pulumi.deployment.InvokeOptions;
import java.util.concurrent.CompletableFuture;
public final class WebpubsubFunctions {
/**
* Get the resource and its properties.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2021-04-01-preview, 2021-06-01-preview, 2021-09-01-preview, 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static Output getWebPubSub(GetWebPubSubArgs args) {
return getWebPubSub(args, InvokeOptions.Empty);
}
/**
* Get the resource and its properties.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2021-04-01-preview, 2021-06-01-preview, 2021-09-01-preview, 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getWebPubSubPlain(GetWebPubSubPlainArgs args) {
return getWebPubSubPlain(args, InvokeOptions.Empty);
}
/**
* Get the resource and its properties.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2021-04-01-preview, 2021-06-01-preview, 2021-09-01-preview, 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static Output getWebPubSub(GetWebPubSubArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:webpubsub:getWebPubSub", TypeShape.of(GetWebPubSubResult.class), args, Utilities.withVersion(options));
}
/**
* Get the resource and its properties.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2021-04-01-preview, 2021-06-01-preview, 2021-09-01-preview, 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getWebPubSubPlain(GetWebPubSubPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:webpubsub:getWebPubSub", TypeShape.of(GetWebPubSubResult.class), args, Utilities.withVersion(options));
}
/**
* Get a custom certificate.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static Output getWebPubSubCustomCertificate(GetWebPubSubCustomCertificateArgs args) {
return getWebPubSubCustomCertificate(args, InvokeOptions.Empty);
}
/**
* Get a custom certificate.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getWebPubSubCustomCertificatePlain(GetWebPubSubCustomCertificatePlainArgs args) {
return getWebPubSubCustomCertificatePlain(args, InvokeOptions.Empty);
}
/**
* Get a custom certificate.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static Output getWebPubSubCustomCertificate(GetWebPubSubCustomCertificateArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:webpubsub:getWebPubSubCustomCertificate", TypeShape.of(GetWebPubSubCustomCertificateResult.class), args, Utilities.withVersion(options));
}
/**
* Get a custom certificate.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getWebPubSubCustomCertificatePlain(GetWebPubSubCustomCertificatePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:webpubsub:getWebPubSubCustomCertificate", TypeShape.of(GetWebPubSubCustomCertificateResult.class), args, Utilities.withVersion(options));
}
/**
* Get a custom domain.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static Output getWebPubSubCustomDomain(GetWebPubSubCustomDomainArgs args) {
return getWebPubSubCustomDomain(args, InvokeOptions.Empty);
}
/**
* Get a custom domain.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getWebPubSubCustomDomainPlain(GetWebPubSubCustomDomainPlainArgs args) {
return getWebPubSubCustomDomainPlain(args, InvokeOptions.Empty);
}
/**
* Get a custom domain.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static Output getWebPubSubCustomDomain(GetWebPubSubCustomDomainArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:webpubsub:getWebPubSubCustomDomain", TypeShape.of(GetWebPubSubCustomDomainResult.class), args, Utilities.withVersion(options));
}
/**
* Get a custom domain.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getWebPubSubCustomDomainPlain(GetWebPubSubCustomDomainPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:webpubsub:getWebPubSubCustomDomain", TypeShape.of(GetWebPubSubCustomDomainResult.class), args, Utilities.withVersion(options));
}
/**
* Get a hub setting.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static Output getWebPubSubHub(GetWebPubSubHubArgs args) {
return getWebPubSubHub(args, InvokeOptions.Empty);
}
/**
* Get a hub setting.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getWebPubSubHubPlain(GetWebPubSubHubPlainArgs args) {
return getWebPubSubHubPlain(args, InvokeOptions.Empty);
}
/**
* Get a hub setting.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static Output getWebPubSubHub(GetWebPubSubHubArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:webpubsub:getWebPubSubHub", TypeShape.of(GetWebPubSubHubResult.class), args, Utilities.withVersion(options));
}
/**
* Get a hub setting.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getWebPubSubHubPlain(GetWebPubSubHubPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:webpubsub:getWebPubSubHub", TypeShape.of(GetWebPubSubHubResult.class), args, Utilities.withVersion(options));
}
/**
* Get the specified private endpoint connection
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static Output getWebPubSubPrivateEndpointConnection(GetWebPubSubPrivateEndpointConnectionArgs args) {
return getWebPubSubPrivateEndpointConnection(args, InvokeOptions.Empty);
}
/**
* Get the specified private endpoint connection
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getWebPubSubPrivateEndpointConnectionPlain(GetWebPubSubPrivateEndpointConnectionPlainArgs args) {
return getWebPubSubPrivateEndpointConnectionPlain(args, InvokeOptions.Empty);
}
/**
* Get the specified private endpoint connection
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static Output getWebPubSubPrivateEndpointConnection(GetWebPubSubPrivateEndpointConnectionArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:webpubsub:getWebPubSubPrivateEndpointConnection", TypeShape.of(GetWebPubSubPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Get the specified private endpoint connection
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getWebPubSubPrivateEndpointConnectionPlain(GetWebPubSubPrivateEndpointConnectionPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:webpubsub:getWebPubSubPrivateEndpointConnection", TypeShape.of(GetWebPubSubPrivateEndpointConnectionResult.class), args, Utilities.withVersion(options));
}
/**
* Get the replica and its properties.
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static Output getWebPubSubReplica(GetWebPubSubReplicaArgs args) {
return getWebPubSubReplica(args, InvokeOptions.Empty);
}
/**
* Get the replica and its properties.
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getWebPubSubReplicaPlain(GetWebPubSubReplicaPlainArgs args) {
return getWebPubSubReplicaPlain(args, InvokeOptions.Empty);
}
/**
* Get the replica and its properties.
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static Output getWebPubSubReplica(GetWebPubSubReplicaArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:webpubsub:getWebPubSubReplica", TypeShape.of(GetWebPubSubReplicaResult.class), args, Utilities.withVersion(options));
}
/**
* Get the replica and its properties.
* Azure REST API version: 2023-03-01-preview.
*
* Other available API versions: 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getWebPubSubReplicaPlain(GetWebPubSubReplicaPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:webpubsub:getWebPubSubReplica", TypeShape.of(GetWebPubSubReplicaResult.class), args, Utilities.withVersion(options));
}
/**
* Get the specified shared private link resource
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static Output getWebPubSubSharedPrivateLinkResource(GetWebPubSubSharedPrivateLinkResourceArgs args) {
return getWebPubSubSharedPrivateLinkResource(args, InvokeOptions.Empty);
}
/**
* Get the specified shared private link resource
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getWebPubSubSharedPrivateLinkResourcePlain(GetWebPubSubSharedPrivateLinkResourcePlainArgs args) {
return getWebPubSubSharedPrivateLinkResourcePlain(args, InvokeOptions.Empty);
}
/**
* Get the specified shared private link resource
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static Output getWebPubSubSharedPrivateLinkResource(GetWebPubSubSharedPrivateLinkResourceArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:webpubsub:getWebPubSubSharedPrivateLinkResource", TypeShape.of(GetWebPubSubSharedPrivateLinkResourceResult.class), args, Utilities.withVersion(options));
}
/**
* Get the specified shared private link resource
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture getWebPubSubSharedPrivateLinkResourcePlain(GetWebPubSubSharedPrivateLinkResourcePlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:webpubsub:getWebPubSubSharedPrivateLinkResource", TypeShape.of(GetWebPubSubSharedPrivateLinkResourceResult.class), args, Utilities.withVersion(options));
}
/**
* Get the access keys of the resource.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2021-04-01-preview, 2021-06-01-preview, 2021-09-01-preview, 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static Output listWebPubSubKeys(ListWebPubSubKeysArgs args) {
return listWebPubSubKeys(args, InvokeOptions.Empty);
}
/**
* Get the access keys of the resource.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2021-04-01-preview, 2021-06-01-preview, 2021-09-01-preview, 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture listWebPubSubKeysPlain(ListWebPubSubKeysPlainArgs args) {
return listWebPubSubKeysPlain(args, InvokeOptions.Empty);
}
/**
* Get the access keys of the resource.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2021-04-01-preview, 2021-06-01-preview, 2021-09-01-preview, 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static Output listWebPubSubKeys(ListWebPubSubKeysArgs args, InvokeOptions options) {
return Deployment.getInstance().invoke("azure-native:webpubsub:listWebPubSubKeys", TypeShape.of(ListWebPubSubKeysResult.class), args, Utilities.withVersion(options));
}
/**
* Get the access keys of the resource.
* Azure REST API version: 2023-02-01.
*
* Other available API versions: 2021-04-01-preview, 2021-06-01-preview, 2021-09-01-preview, 2023-03-01-preview, 2023-06-01-preview, 2023-08-01-preview, 2024-01-01-preview, 2024-03-01, 2024-04-01-preview, 2024-08-01-preview.
*
*/
public static CompletableFuture listWebPubSubKeysPlain(ListWebPubSubKeysPlainArgs args, InvokeOptions options) {
return Deployment.getInstance().invokeAsync("azure-native:webpubsub:listWebPubSubKeys", TypeShape.of(ListWebPubSubKeysResult.class), args, Utilities.withVersion(options));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy