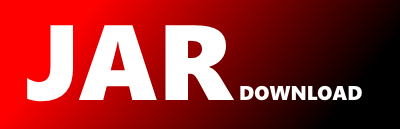
com.pulumi.azurenative.workloads.outputs.SimpleSchedulePolicyResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure-native Show documentation
Show all versions of azure-native Show documentation
A native Pulumi package for creating and managing Azure resources.
The newest version!
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azurenative.workloads.outputs;
import com.pulumi.azurenative.workloads.outputs.HourlyScheduleResponse;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class SimpleSchedulePolicyResponse {
/**
* @return Hourly Schedule of this Policy
*
*/
private @Nullable HourlyScheduleResponse hourlySchedule;
/**
* @return This property will be used as the discriminator for deciding the specific types in the polymorphic chain of types.
* Expected value is 'SimpleSchedulePolicy'.
*
*/
private String schedulePolicyType;
/**
* @return List of days of week this schedule has to be run.
*
*/
private @Nullable List scheduleRunDays;
/**
* @return Frequency of the schedule operation of this policy.
*
*/
private @Nullable String scheduleRunFrequency;
/**
* @return List of times of day this schedule has to be run.
*
*/
private @Nullable List scheduleRunTimes;
/**
* @return At every number weeks this schedule has to be run.
*
*/
private @Nullable Integer scheduleWeeklyFrequency;
private SimpleSchedulePolicyResponse() {}
/**
* @return Hourly Schedule of this Policy
*
*/
public Optional hourlySchedule() {
return Optional.ofNullable(this.hourlySchedule);
}
/**
* @return This property will be used as the discriminator for deciding the specific types in the polymorphic chain of types.
* Expected value is 'SimpleSchedulePolicy'.
*
*/
public String schedulePolicyType() {
return this.schedulePolicyType;
}
/**
* @return List of days of week this schedule has to be run.
*
*/
public List scheduleRunDays() {
return this.scheduleRunDays == null ? List.of() : this.scheduleRunDays;
}
/**
* @return Frequency of the schedule operation of this policy.
*
*/
public Optional scheduleRunFrequency() {
return Optional.ofNullable(this.scheduleRunFrequency);
}
/**
* @return List of times of day this schedule has to be run.
*
*/
public List scheduleRunTimes() {
return this.scheduleRunTimes == null ? List.of() : this.scheduleRunTimes;
}
/**
* @return At every number weeks this schedule has to be run.
*
*/
public Optional scheduleWeeklyFrequency() {
return Optional.ofNullable(this.scheduleWeeklyFrequency);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(SimpleSchedulePolicyResponse defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable HourlyScheduleResponse hourlySchedule;
private String schedulePolicyType;
private @Nullable List scheduleRunDays;
private @Nullable String scheduleRunFrequency;
private @Nullable List scheduleRunTimes;
private @Nullable Integer scheduleWeeklyFrequency;
public Builder() {}
public Builder(SimpleSchedulePolicyResponse defaults) {
Objects.requireNonNull(defaults);
this.hourlySchedule = defaults.hourlySchedule;
this.schedulePolicyType = defaults.schedulePolicyType;
this.scheduleRunDays = defaults.scheduleRunDays;
this.scheduleRunFrequency = defaults.scheduleRunFrequency;
this.scheduleRunTimes = defaults.scheduleRunTimes;
this.scheduleWeeklyFrequency = defaults.scheduleWeeklyFrequency;
}
@CustomType.Setter
public Builder hourlySchedule(@Nullable HourlyScheduleResponse hourlySchedule) {
this.hourlySchedule = hourlySchedule;
return this;
}
@CustomType.Setter
public Builder schedulePolicyType(String schedulePolicyType) {
if (schedulePolicyType == null) {
throw new MissingRequiredPropertyException("SimpleSchedulePolicyResponse", "schedulePolicyType");
}
this.schedulePolicyType = schedulePolicyType;
return this;
}
@CustomType.Setter
public Builder scheduleRunDays(@Nullable List scheduleRunDays) {
this.scheduleRunDays = scheduleRunDays;
return this;
}
public Builder scheduleRunDays(String... scheduleRunDays) {
return scheduleRunDays(List.of(scheduleRunDays));
}
@CustomType.Setter
public Builder scheduleRunFrequency(@Nullable String scheduleRunFrequency) {
this.scheduleRunFrequency = scheduleRunFrequency;
return this;
}
@CustomType.Setter
public Builder scheduleRunTimes(@Nullable List scheduleRunTimes) {
this.scheduleRunTimes = scheduleRunTimes;
return this;
}
public Builder scheduleRunTimes(String... scheduleRunTimes) {
return scheduleRunTimes(List.of(scheduleRunTimes));
}
@CustomType.Setter
public Builder scheduleWeeklyFrequency(@Nullable Integer scheduleWeeklyFrequency) {
this.scheduleWeeklyFrequency = scheduleWeeklyFrequency;
return this;
}
public SimpleSchedulePolicyResponse build() {
final var _resultValue = new SimpleSchedulePolicyResponse();
_resultValue.hourlySchedule = hourlySchedule;
_resultValue.schedulePolicyType = schedulePolicyType;
_resultValue.scheduleRunDays = scheduleRunDays;
_resultValue.scheduleRunFrequency = scheduleRunFrequency;
_resultValue.scheduleRunTimes = scheduleRunTimes;
_resultValue.scheduleWeeklyFrequency = scheduleWeeklyFrequency;
return _resultValue;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy