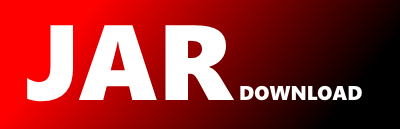
com.pulumi.azure.datafactory.outputs.LinkedServiceAzureDatabricksNewClusterConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure Show documentation
Show all versions of azure Show documentation
A Pulumi package for creating and managing Microsoft Azure cloud resources, based on the Terraform azurerm provider. We recommend using the [Azure Native provider](https://github.com/pulumi/pulumi-azure-native) to provision Azure infrastructure. Azure Native provides complete coverage of Azure resources and same-day access to new resources and resource updates.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.datafactory.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class LinkedServiceAzureDatabricksNewClusterConfig {
/**
* @return Spark version of a the cluster.
*
*/
private String clusterVersion;
/**
* @return Tags for the cluster resource.
*
*/
private @Nullable Map customTags;
/**
* @return Driver node type for the cluster.
*
*/
private @Nullable String driverNodeType;
/**
* @return User defined initialization scripts for the cluster.
*
*/
private @Nullable List initScripts;
/**
* @return Location to deliver Spark driver, worker, and event logs.
*
*/
private @Nullable String logDestination;
/**
* @return Specifies the maximum number of worker nodes. It should be between 1 and 25000.
*
*/
private @Nullable Integer maxNumberOfWorkers;
/**
* @return Specifies the minimum number of worker nodes. It should be between 1 and 25000. It defaults to `1`.
*
*/
private @Nullable Integer minNumberOfWorkers;
/**
* @return Node type for the new cluster.
*
*/
private String nodeType;
/**
* @return User-specified Spark configuration variables key-value pairs.
*
*/
private @Nullable Map sparkConfig;
/**
* @return User-specified Spark environment variables key-value pairs.
*
*/
private @Nullable Map sparkEnvironmentVariables;
private LinkedServiceAzureDatabricksNewClusterConfig() {}
/**
* @return Spark version of a the cluster.
*
*/
public String clusterVersion() {
return this.clusterVersion;
}
/**
* @return Tags for the cluster resource.
*
*/
public Map customTags() {
return this.customTags == null ? Map.of() : this.customTags;
}
/**
* @return Driver node type for the cluster.
*
*/
public Optional driverNodeType() {
return Optional.ofNullable(this.driverNodeType);
}
/**
* @return User defined initialization scripts for the cluster.
*
*/
public List initScripts() {
return this.initScripts == null ? List.of() : this.initScripts;
}
/**
* @return Location to deliver Spark driver, worker, and event logs.
*
*/
public Optional logDestination() {
return Optional.ofNullable(this.logDestination);
}
/**
* @return Specifies the maximum number of worker nodes. It should be between 1 and 25000.
*
*/
public Optional maxNumberOfWorkers() {
return Optional.ofNullable(this.maxNumberOfWorkers);
}
/**
* @return Specifies the minimum number of worker nodes. It should be between 1 and 25000. It defaults to `1`.
*
*/
public Optional minNumberOfWorkers() {
return Optional.ofNullable(this.minNumberOfWorkers);
}
/**
* @return Node type for the new cluster.
*
*/
public String nodeType() {
return this.nodeType;
}
/**
* @return User-specified Spark configuration variables key-value pairs.
*
*/
public Map sparkConfig() {
return this.sparkConfig == null ? Map.of() : this.sparkConfig;
}
/**
* @return User-specified Spark environment variables key-value pairs.
*
*/
public Map sparkEnvironmentVariables() {
return this.sparkEnvironmentVariables == null ? Map.of() : this.sparkEnvironmentVariables;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(LinkedServiceAzureDatabricksNewClusterConfig defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String clusterVersion;
private @Nullable Map customTags;
private @Nullable String driverNodeType;
private @Nullable List initScripts;
private @Nullable String logDestination;
private @Nullable Integer maxNumberOfWorkers;
private @Nullable Integer minNumberOfWorkers;
private String nodeType;
private @Nullable Map sparkConfig;
private @Nullable Map sparkEnvironmentVariables;
public Builder() {}
public Builder(LinkedServiceAzureDatabricksNewClusterConfig defaults) {
Objects.requireNonNull(defaults);
this.clusterVersion = defaults.clusterVersion;
this.customTags = defaults.customTags;
this.driverNodeType = defaults.driverNodeType;
this.initScripts = defaults.initScripts;
this.logDestination = defaults.logDestination;
this.maxNumberOfWorkers = defaults.maxNumberOfWorkers;
this.minNumberOfWorkers = defaults.minNumberOfWorkers;
this.nodeType = defaults.nodeType;
this.sparkConfig = defaults.sparkConfig;
this.sparkEnvironmentVariables = defaults.sparkEnvironmentVariables;
}
@CustomType.Setter
public Builder clusterVersion(String clusterVersion) {
if (clusterVersion == null) {
throw new MissingRequiredPropertyException("LinkedServiceAzureDatabricksNewClusterConfig", "clusterVersion");
}
this.clusterVersion = clusterVersion;
return this;
}
@CustomType.Setter
public Builder customTags(@Nullable Map customTags) {
this.customTags = customTags;
return this;
}
@CustomType.Setter
public Builder driverNodeType(@Nullable String driverNodeType) {
this.driverNodeType = driverNodeType;
return this;
}
@CustomType.Setter
public Builder initScripts(@Nullable List initScripts) {
this.initScripts = initScripts;
return this;
}
public Builder initScripts(String... initScripts) {
return initScripts(List.of(initScripts));
}
@CustomType.Setter
public Builder logDestination(@Nullable String logDestination) {
this.logDestination = logDestination;
return this;
}
@CustomType.Setter
public Builder maxNumberOfWorkers(@Nullable Integer maxNumberOfWorkers) {
this.maxNumberOfWorkers = maxNumberOfWorkers;
return this;
}
@CustomType.Setter
public Builder minNumberOfWorkers(@Nullable Integer minNumberOfWorkers) {
this.minNumberOfWorkers = minNumberOfWorkers;
return this;
}
@CustomType.Setter
public Builder nodeType(String nodeType) {
if (nodeType == null) {
throw new MissingRequiredPropertyException("LinkedServiceAzureDatabricksNewClusterConfig", "nodeType");
}
this.nodeType = nodeType;
return this;
}
@CustomType.Setter
public Builder sparkConfig(@Nullable Map sparkConfig) {
this.sparkConfig = sparkConfig;
return this;
}
@CustomType.Setter
public Builder sparkEnvironmentVariables(@Nullable Map sparkEnvironmentVariables) {
this.sparkEnvironmentVariables = sparkEnvironmentVariables;
return this;
}
public LinkedServiceAzureDatabricksNewClusterConfig build() {
final var _resultValue = new LinkedServiceAzureDatabricksNewClusterConfig();
_resultValue.clusterVersion = clusterVersion;
_resultValue.customTags = customTags;
_resultValue.driverNodeType = driverNodeType;
_resultValue.initScripts = initScripts;
_resultValue.logDestination = logDestination;
_resultValue.maxNumberOfWorkers = maxNumberOfWorkers;
_resultValue.minNumberOfWorkers = minNumberOfWorkers;
_resultValue.nodeType = nodeType;
_resultValue.sparkConfig = sparkConfig;
_resultValue.sparkEnvironmentVariables = sparkEnvironmentVariables;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy