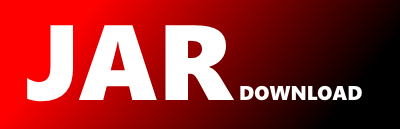
com.pulumi.azure.streamanalytics.inputs.OutputPowerbiState Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure Show documentation
Show all versions of azure Show documentation
A Pulumi package for creating and managing Microsoft Azure cloud resources, based on the Terraform azurerm provider. We recommend using the [Azure Native provider](https://github.com/pulumi/pulumi-azure-native) to provision Azure infrastructure. Azure Native provides complete coverage of Azure resources and same-day access to new resources and resource updates.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.streamanalytics.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class OutputPowerbiState extends com.pulumi.resources.ResourceArgs {
public static final OutputPowerbiState Empty = new OutputPowerbiState();
/**
* The name of the Power BI dataset.
*
*/
@Import(name="dataset")
private @Nullable Output dataset;
/**
* @return The name of the Power BI dataset.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy