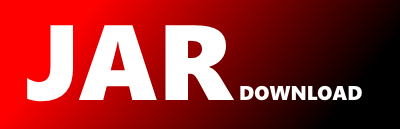
com.pulumi.azure.appservice.LinuxFunctionAppSlot Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.appservice;
import com.pulumi.azure.Utilities;
import com.pulumi.azure.appservice.LinuxFunctionAppSlotArgs;
import com.pulumi.azure.appservice.inputs.LinuxFunctionAppSlotState;
import com.pulumi.azure.appservice.outputs.LinuxFunctionAppSlotAuthSettings;
import com.pulumi.azure.appservice.outputs.LinuxFunctionAppSlotAuthSettingsV2;
import com.pulumi.azure.appservice.outputs.LinuxFunctionAppSlotBackup;
import com.pulumi.azure.appservice.outputs.LinuxFunctionAppSlotConnectionString;
import com.pulumi.azure.appservice.outputs.LinuxFunctionAppSlotIdentity;
import com.pulumi.azure.appservice.outputs.LinuxFunctionAppSlotSiteConfig;
import com.pulumi.azure.appservice.outputs.LinuxFunctionAppSlotSiteCredential;
import com.pulumi.azure.appservice.outputs.LinuxFunctionAppSlotStorageAccount;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages a Linux Function App Slot.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.storage.Account;
* import com.pulumi.azure.storage.AccountArgs;
* import com.pulumi.azure.appservice.ServicePlan;
* import com.pulumi.azure.appservice.ServicePlanArgs;
* import com.pulumi.azure.appservice.LinuxFunctionApp;
* import com.pulumi.azure.appservice.LinuxFunctionAppArgs;
* import com.pulumi.azure.appservice.inputs.LinuxFunctionAppSiteConfigArgs;
* import com.pulumi.azure.appservice.LinuxFunctionAppSlot;
* import com.pulumi.azure.appservice.LinuxFunctionAppSlotArgs;
* import com.pulumi.azure.appservice.inputs.LinuxFunctionAppSlotSiteConfigArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
*
* var exampleAccount = new Account("exampleAccount", AccountArgs.builder()
* .name("linuxfunctionappsa")
* .resourceGroupName(example.name())
* .location(example.location())
* .accountTier("Standard")
* .accountReplicationType("LRS")
* .build());
*
* var exampleServicePlan = new ServicePlan("exampleServicePlan", ServicePlanArgs.builder()
* .name("example-app-service-plan")
* .resourceGroupName(example.name())
* .location(example.location())
* .osType("Linux")
* .skuName("Y1")
* .build());
*
* var exampleLinuxFunctionApp = new LinuxFunctionApp("exampleLinuxFunctionApp", LinuxFunctionAppArgs.builder()
* .name("example-linux-function-app")
* .resourceGroupName(example.name())
* .location(example.location())
* .servicePlanId(exampleServicePlan.id())
* .storageAccountName(exampleAccount.name())
* .siteConfig()
* .build());
*
* var exampleLinuxFunctionAppSlot = new LinuxFunctionAppSlot("exampleLinuxFunctionAppSlot", LinuxFunctionAppSlotArgs.builder()
* .name("example-linux-function-app-slot")
* .functionAppId(exampleLinuxFunctionApp.id())
* .storageAccountName(exampleAccount.name())
* .siteConfig()
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* A Linux Function App Slot can be imported using the `resource id`, e.g.
*
* ```sh
* $ pulumi import azure:appservice/linuxFunctionAppSlot:LinuxFunctionAppSlot example "/subscriptions/12345678-1234-9876-4563-123456789012/resourceGroups/resGroup1/providers/Microsoft.Web/sites/site1/slots/slot1"
* ```
*
*/
@ResourceType(type="azure:appservice/linuxFunctionAppSlot:LinuxFunctionAppSlot")
public class LinuxFunctionAppSlot extends com.pulumi.resources.CustomResource {
/**
* A map of key-value pairs for [App Settings](https://docs.microsoft.com/azure/azure-functions/functions-app-settings) and custom values.
*
*/
@Export(name="appSettings", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> appSettings;
/**
* @return A map of key-value pairs for [App Settings](https://docs.microsoft.com/azure/azure-functions/functions-app-settings) and custom values.
*
*/
public Output>> appSettings() {
return Codegen.optional(this.appSettings);
}
/**
* an `auth_settings` block as detailed below.
*
*/
@Export(name="authSettings", refs={LinuxFunctionAppSlotAuthSettings.class}, tree="[0]")
private Output* @Nullable */ LinuxFunctionAppSlotAuthSettings> authSettings;
/**
* @return an `auth_settings` block as detailed below.
*
*/
public Output> authSettings() {
return Codegen.optional(this.authSettings);
}
/**
* an `auth_settings_v2` block as detailed below.
*
*/
@Export(name="authSettingsV2", refs={LinuxFunctionAppSlotAuthSettingsV2.class}, tree="[0]")
private Output* @Nullable */ LinuxFunctionAppSlotAuthSettingsV2> authSettingsV2;
/**
* @return an `auth_settings_v2` block as detailed below.
*
*/
public Output> authSettingsV2() {
return Codegen.optional(this.authSettingsV2);
}
/**
* a `backup` block as detailed below.
*
*/
@Export(name="backup", refs={LinuxFunctionAppSlotBackup.class}, tree="[0]")
private Output* @Nullable */ LinuxFunctionAppSlotBackup> backup;
/**
* @return a `backup` block as detailed below.
*
*/
public Output> backup() {
return Codegen.optional(this.backup);
}
/**
* Should built in logging be enabled. Configures `AzureWebJobsDashboard` app setting based on the configured storage setting. Defaults to `true`.
*
*/
@Export(name="builtinLoggingEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> builtinLoggingEnabled;
/**
* @return Should built in logging be enabled. Configures `AzureWebJobsDashboard` app setting based on the configured storage setting. Defaults to `true`.
*
*/
public Output> builtinLoggingEnabled() {
return Codegen.optional(this.builtinLoggingEnabled);
}
/**
* Should the Function App Slot use Client Certificates.
*
*/
@Export(name="clientCertificateEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> clientCertificateEnabled;
/**
* @return Should the Function App Slot use Client Certificates.
*
*/
public Output> clientCertificateEnabled() {
return Codegen.optional(this.clientCertificateEnabled);
}
/**
* Paths to exclude when using client certificates, separated by ;
*
*/
@Export(name="clientCertificateExclusionPaths", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> clientCertificateExclusionPaths;
/**
* @return Paths to exclude when using client certificates, separated by ;
*
*/
public Output> clientCertificateExclusionPaths() {
return Codegen.optional(this.clientCertificateExclusionPaths);
}
/**
* The mode of the Function App Slot's client certificates requirement for incoming requests. Possible values are `Required`, `Optional`, and `OptionalInteractiveUser`. Defaults to `Optional`.
*
*/
@Export(name="clientCertificateMode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> clientCertificateMode;
/**
* @return The mode of the Function App Slot's client certificates requirement for incoming requests. Possible values are `Required`, `Optional`, and `OptionalInteractiveUser`. Defaults to `Optional`.
*
*/
public Output> clientCertificateMode() {
return Codegen.optional(this.clientCertificateMode);
}
/**
* a `connection_string` block as detailed below.
*
*/
@Export(name="connectionStrings", refs={List.class,LinuxFunctionAppSlotConnectionString.class}, tree="[0,1]")
private Output* @Nullable */ List> connectionStrings;
/**
* @return a `connection_string` block as detailed below.
*
*/
public Output>> connectionStrings() {
return Codegen.optional(this.connectionStrings);
}
/**
* Force disable the content share settings.
*
*/
@Export(name="contentShareForceDisabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> contentShareForceDisabled;
/**
* @return Force disable the content share settings.
*
*/
public Output> contentShareForceDisabled() {
return Codegen.optional(this.contentShareForceDisabled);
}
/**
* The identifier used by App Service to perform domain ownership verification via DNS TXT record.
*
*/
@Export(name="customDomainVerificationId", refs={String.class}, tree="[0]")
private Output customDomainVerificationId;
/**
* @return The identifier used by App Service to perform domain ownership verification via DNS TXT record.
*
*/
public Output customDomainVerificationId() {
return this.customDomainVerificationId;
}
/**
* The amount of memory in gigabyte-seconds that your application is allowed to consume per day. Setting this value only affects function apps in Consumption Plans. Defaults to `0`.
*
*/
@Export(name="dailyMemoryTimeQuota", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> dailyMemoryTimeQuota;
/**
* @return The amount of memory in gigabyte-seconds that your application is allowed to consume per day. Setting this value only affects function apps in Consumption Plans. Defaults to `0`.
*
*/
public Output> dailyMemoryTimeQuota() {
return Codegen.optional(this.dailyMemoryTimeQuota);
}
/**
* The default hostname of the Linux Function App Slot.
*
*/
@Export(name="defaultHostname", refs={String.class}, tree="[0]")
private Output defaultHostname;
/**
* @return The default hostname of the Linux Function App Slot.
*
*/
public Output defaultHostname() {
return this.defaultHostname;
}
/**
* Is the Linux Function App Slot enabled. Defaults to `true`.
*
*/
@Export(name="enabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> enabled;
/**
* @return Is the Linux Function App Slot enabled. Defaults to `true`.
*
*/
public Output> enabled() {
return Codegen.optional(this.enabled);
}
/**
* Are the default FTP Basic Authentication publishing credentials enabled. Defaults to `true`.
*
*/
@Export(name="ftpPublishBasicAuthenticationEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> ftpPublishBasicAuthenticationEnabled;
/**
* @return Are the default FTP Basic Authentication publishing credentials enabled. Defaults to `true`.
*
*/
public Output> ftpPublishBasicAuthenticationEnabled() {
return Codegen.optional(this.ftpPublishBasicAuthenticationEnabled);
}
/**
* The ID of the Linux Function App this Slot is a member of. Changing this forces a new resource to be created.
*
*/
@Export(name="functionAppId", refs={String.class}, tree="[0]")
private Output functionAppId;
/**
* @return The ID of the Linux Function App this Slot is a member of. Changing this forces a new resource to be created.
*
*/
public Output functionAppId() {
return this.functionAppId;
}
/**
* The runtime version associated with the Function App Slot. Defaults to `~4`.
*
*/
@Export(name="functionsExtensionVersion", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> functionsExtensionVersion;
/**
* @return The runtime version associated with the Function App Slot. Defaults to `~4`.
*
*/
public Output> functionsExtensionVersion() {
return Codegen.optional(this.functionsExtensionVersion);
}
/**
* The ID of the App Service Environment used by Function App Slot.
*
*/
@Export(name="hostingEnvironmentId", refs={String.class}, tree="[0]")
private Output hostingEnvironmentId;
/**
* @return The ID of the App Service Environment used by Function App Slot.
*
*/
public Output hostingEnvironmentId() {
return this.hostingEnvironmentId;
}
/**
* Can the Function App Slot only be accessed via HTTPS?. Defaults to `false`.
*
*/
@Export(name="httpsOnly", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> httpsOnly;
/**
* @return Can the Function App Slot only be accessed via HTTPS?. Defaults to `false`.
*
*/
public Output> httpsOnly() {
return Codegen.optional(this.httpsOnly);
}
/**
* An `identity` block as detailed below.
*
*/
@Export(name="identity", refs={LinuxFunctionAppSlotIdentity.class}, tree="[0]")
private Output* @Nullable */ LinuxFunctionAppSlotIdentity> identity;
/**
* @return An `identity` block as detailed below.
*
*/
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* The User Assigned Identity ID used for accessing KeyVault secrets. The identity must be assigned to the application in the `identity` block. [For more information see - Access vaults with a user-assigned identity](https://docs.microsoft.com/azure/app-service/app-service-key-vault-references#access-vaults-with-a-user-assigned-identity)
*
*/
@Export(name="keyVaultReferenceIdentityId", refs={String.class}, tree="[0]")
private Output keyVaultReferenceIdentityId;
/**
* @return The User Assigned Identity ID used for accessing KeyVault secrets. The identity must be assigned to the application in the `identity` block. [For more information see - Access vaults with a user-assigned identity](https://docs.microsoft.com/azure/app-service/app-service-key-vault-references#access-vaults-with-a-user-assigned-identity)
*
*/
public Output keyVaultReferenceIdentityId() {
return this.keyVaultReferenceIdentityId;
}
/**
* The Kind value for this Linux Function App Slot.
*
*/
@Export(name="kind", refs={String.class}, tree="[0]")
private Output kind;
/**
* @return The Kind value for this Linux Function App Slot.
*
*/
public Output kind() {
return this.kind;
}
/**
* Specifies the name of the Function App Slot. Changing this forces a new resource to be created.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Specifies the name of the Function App Slot. Changing this forces a new resource to be created.
*
*/
public Output name() {
return this.name;
}
/**
* A list of outbound IP addresses. For example `["52.23.25.3", "52.143.43.12"]`
*
*/
@Export(name="outboundIpAddressLists", refs={List.class,String.class}, tree="[0,1]")
private Output> outboundIpAddressLists;
/**
* @return A list of outbound IP addresses. For example `["52.23.25.3", "52.143.43.12"]`
*
*/
public Output> outboundIpAddressLists() {
return this.outboundIpAddressLists;
}
/**
* A comma separated list of outbound IP addresses as a string. For example `52.23.25.3,52.143.43.12`.
*
*/
@Export(name="outboundIpAddresses", refs={String.class}, tree="[0]")
private Output outboundIpAddresses;
/**
* @return A comma separated list of outbound IP addresses as a string. For example `52.23.25.3,52.143.43.12`.
*
*/
public Output outboundIpAddresses() {
return this.outboundIpAddresses;
}
/**
* A list of possible outbound IP addresses, not all of which are necessarily in use. This is a superset of `outbound_ip_address_list`. For example `["52.23.25.3", "52.143.43.12"]`.
*
*/
@Export(name="possibleOutboundIpAddressLists", refs={List.class,String.class}, tree="[0,1]")
private Output> possibleOutboundIpAddressLists;
/**
* @return A list of possible outbound IP addresses, not all of which are necessarily in use. This is a superset of `outbound_ip_address_list`. For example `["52.23.25.3", "52.143.43.12"]`.
*
*/
public Output> possibleOutboundIpAddressLists() {
return this.possibleOutboundIpAddressLists;
}
/**
* A comma separated list of possible outbound IP addresses as a string. For example `52.23.25.3,52.143.43.12,52.143.43.17`. This is a superset of `outbound_ip_addresses`. For example `["52.23.25.3", "52.143.43.12","52.143.43.17"]`.
*
*/
@Export(name="possibleOutboundIpAddresses", refs={String.class}, tree="[0]")
private Output possibleOutboundIpAddresses;
/**
* @return A comma separated list of possible outbound IP addresses as a string. For example `52.23.25.3,52.143.43.12,52.143.43.17`. This is a superset of `outbound_ip_addresses`. For example `["52.23.25.3", "52.143.43.12","52.143.43.17"]`.
*
*/
public Output possibleOutboundIpAddresses() {
return this.possibleOutboundIpAddresses;
}
/**
* Should public network access be enabled for the Function App. Defaults to `true`.
*
*/
@Export(name="publicNetworkAccessEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> publicNetworkAccessEnabled;
/**
* @return Should public network access be enabled for the Function App. Defaults to `true`.
*
*/
public Output> publicNetworkAccessEnabled() {
return Codegen.optional(this.publicNetworkAccessEnabled);
}
/**
* The ID of the Service Plan in which to run this slot. If not specified the same Service Plan as the Linux Function App will be used.
*
*/
@Export(name="servicePlanId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> servicePlanId;
/**
* @return The ID of the Service Plan in which to run this slot. If not specified the same Service Plan as the Linux Function App will be used.
*
*/
public Output> servicePlanId() {
return Codegen.optional(this.servicePlanId);
}
/**
* a `site_config` block as detailed below.
*
*/
@Export(name="siteConfig", refs={LinuxFunctionAppSlotSiteConfig.class}, tree="[0]")
private Output siteConfig;
/**
* @return a `site_config` block as detailed below.
*
*/
public Output siteConfig() {
return this.siteConfig;
}
/**
* A `site_credential` block as defined below.
*
*/
@Export(name="siteCredentials", refs={List.class,LinuxFunctionAppSlotSiteCredential.class}, tree="[0,1]")
private Output> siteCredentials;
/**
* @return A `site_credential` block as defined below.
*
*/
public Output> siteCredentials() {
return this.siteCredentials;
}
/**
* The access key which will be used to access the storage account for the Function App Slot.
*
*/
@Export(name="storageAccountAccessKey", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> storageAccountAccessKey;
/**
* @return The access key which will be used to access the storage account for the Function App Slot.
*
*/
public Output> storageAccountAccessKey() {
return Codegen.optional(this.storageAccountAccessKey);
}
/**
* The backend storage account name which will be used by this Function App Slot.
*
*/
@Export(name="storageAccountName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> storageAccountName;
/**
* @return The backend storage account name which will be used by this Function App Slot.
*
*/
public Output> storageAccountName() {
return Codegen.optional(this.storageAccountName);
}
/**
* One or more `storage_account` blocks as defined below.
*
*/
@Export(name="storageAccounts", refs={List.class,LinuxFunctionAppSlotStorageAccount.class}, tree="[0,1]")
private Output* @Nullable */ List> storageAccounts;
/**
* @return One or more `storage_account` blocks as defined below.
*
*/
public Output>> storageAccounts() {
return Codegen.optional(this.storageAccounts);
}
/**
* The Key Vault Secret ID, optionally including version, that contains the Connection String to connect to the storage account for this Function App.
*
* > **NOTE:** `storage_key_vault_secret_id` cannot be used with `storage_account_name`.
*
* > **NOTE:** `storage_key_vault_secret_id` used without a version will use the latest version of the secret, however, the service can take up to 24h to pick up a rotation of the latest version. See the [official docs](https://docs.microsoft.com/azure/app-service/app-service-key-vault-references#rotation) for more information.
*
*/
@Export(name="storageKeyVaultSecretId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> storageKeyVaultSecretId;
/**
* @return The Key Vault Secret ID, optionally including version, that contains the Connection String to connect to the storage account for this Function App.
*
* > **NOTE:** `storage_key_vault_secret_id` cannot be used with `storage_account_name`.
*
* > **NOTE:** `storage_key_vault_secret_id` used without a version will use the latest version of the secret, however, the service can take up to 24h to pick up a rotation of the latest version. See the [official docs](https://docs.microsoft.com/azure/app-service/app-service-key-vault-references#rotation) for more information.
*
*/
public Output> storageKeyVaultSecretId() {
return Codegen.optional(this.storageKeyVaultSecretId);
}
/**
* Should the Function App Slot use its Managed Identity to access storage.
*
* > **NOTE:** One of `storage_account_access_key` or `storage_uses_managed_identity` must be specified when using `storage_account_name`.
*
*/
@Export(name="storageUsesManagedIdentity", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> storageUsesManagedIdentity;
/**
* @return Should the Function App Slot use its Managed Identity to access storage.
*
* > **NOTE:** One of `storage_account_access_key` or `storage_uses_managed_identity` must be specified when using `storage_account_name`.
*
*/
public Output> storageUsesManagedIdentity() {
return Codegen.optional(this.storageUsesManagedIdentity);
}
/**
* A mapping of tags which should be assigned to the Linux Function App.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A mapping of tags which should be assigned to the Linux Function App.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
@Export(name="virtualNetworkSubnetId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> virtualNetworkSubnetId;
public Output> virtualNetworkSubnetId() {
return Codegen.optional(this.virtualNetworkSubnetId);
}
/**
* Is container image pull over virtual network enabled? Defaults to `false`.
*
*/
@Export(name="vnetImagePullEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> vnetImagePullEnabled;
/**
* @return Is container image pull over virtual network enabled? Defaults to `false`.
*
*/
public Output> vnetImagePullEnabled() {
return Codegen.optional(this.vnetImagePullEnabled);
}
/**
* Should the default WebDeploy Basic Authentication publishing credentials enabled. Defaults to `true`.
*
*/
@Export(name="webdeployPublishBasicAuthenticationEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> webdeployPublishBasicAuthenticationEnabled;
/**
* @return Should the default WebDeploy Basic Authentication publishing credentials enabled. Defaults to `true`.
*
*/
public Output> webdeployPublishBasicAuthenticationEnabled() {
return Codegen.optional(this.webdeployPublishBasicAuthenticationEnabled);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public LinuxFunctionAppSlot(java.lang.String name) {
this(name, LinuxFunctionAppSlotArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public LinuxFunctionAppSlot(java.lang.String name, LinuxFunctionAppSlotArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public LinuxFunctionAppSlot(java.lang.String name, LinuxFunctionAppSlotArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure:appservice/linuxFunctionAppSlot:LinuxFunctionAppSlot", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private LinuxFunctionAppSlot(java.lang.String name, Output id, @Nullable LinuxFunctionAppSlotState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure:appservice/linuxFunctionAppSlot:LinuxFunctionAppSlot", name, state, makeResourceOptions(options, id), false);
}
private static LinuxFunctionAppSlotArgs makeArgs(LinuxFunctionAppSlotArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? LinuxFunctionAppSlotArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.additionalSecretOutputs(List.of(
"customDomainVerificationId",
"siteCredentials",
"storageAccountAccessKey"
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static LinuxFunctionAppSlot get(java.lang.String name, Output id, @Nullable LinuxFunctionAppSlotState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new LinuxFunctionAppSlot(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy