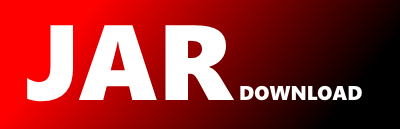
com.pulumi.azure.appservice.outputs.FunctionAppSlotAuthSettingsGoogle Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.appservice.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import javax.annotation.Nullable;
@CustomType
public final class FunctionAppSlotAuthSettingsGoogle {
/**
* @return The OpenID Connect Client ID for the Google web application.
*
*/
private String clientId;
/**
* @return The client secret associated with the Google web application.
*
*/
private String clientSecret;
/**
* @return The OAuth 2.0 scopes that will be requested as part of Google Sign-In authentication. <https://developers.google.com/identity/sign-in/web/>
*
*/
private @Nullable List oauthScopes;
private FunctionAppSlotAuthSettingsGoogle() {}
/**
* @return The OpenID Connect Client ID for the Google web application.
*
*/
public String clientId() {
return this.clientId;
}
/**
* @return The client secret associated with the Google web application.
*
*/
public String clientSecret() {
return this.clientSecret;
}
/**
* @return The OAuth 2.0 scopes that will be requested as part of Google Sign-In authentication. <https://developers.google.com/identity/sign-in/web/>
*
*/
public List oauthScopes() {
return this.oauthScopes == null ? List.of() : this.oauthScopes;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(FunctionAppSlotAuthSettingsGoogle defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String clientId;
private String clientSecret;
private @Nullable List oauthScopes;
public Builder() {}
public Builder(FunctionAppSlotAuthSettingsGoogle defaults) {
Objects.requireNonNull(defaults);
this.clientId = defaults.clientId;
this.clientSecret = defaults.clientSecret;
this.oauthScopes = defaults.oauthScopes;
}
@CustomType.Setter
public Builder clientId(String clientId) {
if (clientId == null) {
throw new MissingRequiredPropertyException("FunctionAppSlotAuthSettingsGoogle", "clientId");
}
this.clientId = clientId;
return this;
}
@CustomType.Setter
public Builder clientSecret(String clientSecret) {
if (clientSecret == null) {
throw new MissingRequiredPropertyException("FunctionAppSlotAuthSettingsGoogle", "clientSecret");
}
this.clientSecret = clientSecret;
return this;
}
@CustomType.Setter
public Builder oauthScopes(@Nullable List oauthScopes) {
this.oauthScopes = oauthScopes;
return this;
}
public Builder oauthScopes(String... oauthScopes) {
return oauthScopes(List.of(oauthScopes));
}
public FunctionAppSlotAuthSettingsGoogle build() {
final var _resultValue = new FunctionAppSlotAuthSettingsGoogle();
_resultValue.clientId = clientId;
_resultValue.clientSecret = clientSecret;
_resultValue.oauthScopes = oauthScopes;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy