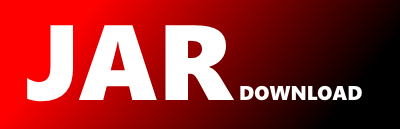
com.pulumi.azure.appservice.outputs.FunctionAppSourceControl Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.appservice.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.Boolean;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class FunctionAppSourceControl {
/**
* @return The branch of the remote repository to use. Defaults to 'master'.
*
*/
private @Nullable String branch;
/**
* @return Limits to manual integration. Defaults to `false` if not specified.
*
*/
private @Nullable Boolean manualIntegration;
/**
* @return The URL of the source code repository.
*
*/
private @Nullable String repoUrl;
/**
* @return Enable roll-back for the repository. Defaults to `false` if not specified.
*
*/
private @Nullable Boolean rollbackEnabled;
/**
* @return Use Mercurial if `true`, otherwise uses Git.
*
*/
private @Nullable Boolean useMercurial;
private FunctionAppSourceControl() {}
/**
* @return The branch of the remote repository to use. Defaults to 'master'.
*
*/
public Optional branch() {
return Optional.ofNullable(this.branch);
}
/**
* @return Limits to manual integration. Defaults to `false` if not specified.
*
*/
public Optional manualIntegration() {
return Optional.ofNullable(this.manualIntegration);
}
/**
* @return The URL of the source code repository.
*
*/
public Optional repoUrl() {
return Optional.ofNullable(this.repoUrl);
}
/**
* @return Enable roll-back for the repository. Defaults to `false` if not specified.
*
*/
public Optional rollbackEnabled() {
return Optional.ofNullable(this.rollbackEnabled);
}
/**
* @return Use Mercurial if `true`, otherwise uses Git.
*
*/
public Optional useMercurial() {
return Optional.ofNullable(this.useMercurial);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(FunctionAppSourceControl defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String branch;
private @Nullable Boolean manualIntegration;
private @Nullable String repoUrl;
private @Nullable Boolean rollbackEnabled;
private @Nullable Boolean useMercurial;
public Builder() {}
public Builder(FunctionAppSourceControl defaults) {
Objects.requireNonNull(defaults);
this.branch = defaults.branch;
this.manualIntegration = defaults.manualIntegration;
this.repoUrl = defaults.repoUrl;
this.rollbackEnabled = defaults.rollbackEnabled;
this.useMercurial = defaults.useMercurial;
}
@CustomType.Setter
public Builder branch(@Nullable String branch) {
this.branch = branch;
return this;
}
@CustomType.Setter
public Builder manualIntegration(@Nullable Boolean manualIntegration) {
this.manualIntegration = manualIntegration;
return this;
}
@CustomType.Setter
public Builder repoUrl(@Nullable String repoUrl) {
this.repoUrl = repoUrl;
return this;
}
@CustomType.Setter
public Builder rollbackEnabled(@Nullable Boolean rollbackEnabled) {
this.rollbackEnabled = rollbackEnabled;
return this;
}
@CustomType.Setter
public Builder useMercurial(@Nullable Boolean useMercurial) {
this.useMercurial = useMercurial;
return this;
}
public FunctionAppSourceControl build() {
final var _resultValue = new FunctionAppSourceControl();
_resultValue.branch = branch;
_resultValue.manualIntegration = manualIntegration;
_resultValue.repoUrl = repoUrl;
_resultValue.rollbackEnabled = rollbackEnabled;
_resultValue.useMercurial = useMercurial;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy