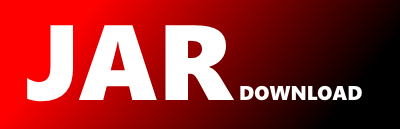
com.pulumi.azure.appservice.outputs.LinuxWebAppSiteConfigApplicationStack Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.appservice.outputs;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class LinuxWebAppSiteConfigApplicationStack {
/**
* @return The docker image, including tag, to be used. e.g. `appsvc/staticsite:latest`.
*
*/
private @Nullable String dockerImageName;
/**
* @return The User Name to use for authentication against the registry to pull the image.
*
* > **NOTE:** `docker_registry_url`, `docker_registry_username`, and `docker_registry_password` replace the use of the `app_settings` values of `DOCKER_REGISTRY_SERVER_URL`, `DOCKER_REGISTRY_SERVER_USERNAME` and `DOCKER_REGISTRY_SERVER_PASSWORD` respectively, these values will be managed by the provider and should not be specified in the `app_settings` map.
*
*/
private @Nullable String dockerRegistryPassword;
/**
* @return The URL of the container registry where the `docker_image_name` is located. e.g. `https://index.docker.io` or `https://mcr.microsoft.com`. This value is required with `docker_image_name`.
*
*/
private @Nullable String dockerRegistryUrl;
/**
* @return The User Name to use for authentication against the registry to pull the image.
*
*/
private @Nullable String dockerRegistryUsername;
/**
* @return The version of .NET to use. Possible values include `3.1`, `5.0`, `6.0`, `7.0` and `8.0`.
*
*/
private @Nullable String dotnetVersion;
/**
* @return The version of Go to use. Possible values include `1.18`, and `1.19`.
*
*/
private @Nullable String goVersion;
/**
* @return The Java server type. Possible values include `JAVA`, `TOMCAT`, and `JBOSSEAP`.
*
* > **NOTE:** `JBOSSEAP` requires a Premium Service Plan SKU to be a valid option.
*
*/
private @Nullable String javaServer;
/**
* @return The Version of the `java_server` to use.
*
*/
private @Nullable String javaServerVersion;
/**
* @return The Version of Java to use. Possible values include `8`, `11`, and `17`.
*
* > **NOTE:** The valid version combinations for `java_version`, `java_server` and `java_server_version` can be checked from the command line via `az webapp list-runtimes --linux`.
*
* > **NOTE:** `java_server`, `java_server_version`, and `java_version` must all be specified if building a java app
*
*/
private @Nullable String javaVersion;
/**
* @return The version of Node to run. Possible values include `12-lts`, `14-lts`, `16-lts`, `18-lts` and `20-lts`. This property conflicts with `java_version`.
*
* > **NOTE:** 10.x versions have been/are being deprecated so may cease to work for new resources in the future and may be removed from the provider.
*
*/
private @Nullable String nodeVersion;
/**
* @return The version of PHP to run. Possible values are `7.4`, `8.0`, `8.1`, `8.2` and `8.3`.
*
* > **NOTE:** version `7.4` is deprecated and will be removed from the provider in a future version.
*
*/
private @Nullable String phpVersion;
/**
* @return The version of Python to run. Possible values include `3.7`, `3.8`, `3.9`, `3.10`, `3.11` and `3.12`.
*
*/
private @Nullable String pythonVersion;
/**
* @return The version of Ruby to run. Possible values include `2.6` and `2.7`.
*
*/
private @Nullable String rubyVersion;
private LinuxWebAppSiteConfigApplicationStack() {}
/**
* @return The docker image, including tag, to be used. e.g. `appsvc/staticsite:latest`.
*
*/
public Optional dockerImageName() {
return Optional.ofNullable(this.dockerImageName);
}
/**
* @return The User Name to use for authentication against the registry to pull the image.
*
* > **NOTE:** `docker_registry_url`, `docker_registry_username`, and `docker_registry_password` replace the use of the `app_settings` values of `DOCKER_REGISTRY_SERVER_URL`, `DOCKER_REGISTRY_SERVER_USERNAME` and `DOCKER_REGISTRY_SERVER_PASSWORD` respectively, these values will be managed by the provider and should not be specified in the `app_settings` map.
*
*/
public Optional dockerRegistryPassword() {
return Optional.ofNullable(this.dockerRegistryPassword);
}
/**
* @return The URL of the container registry where the `docker_image_name` is located. e.g. `https://index.docker.io` or `https://mcr.microsoft.com`. This value is required with `docker_image_name`.
*
*/
public Optional dockerRegistryUrl() {
return Optional.ofNullable(this.dockerRegistryUrl);
}
/**
* @return The User Name to use for authentication against the registry to pull the image.
*
*/
public Optional dockerRegistryUsername() {
return Optional.ofNullable(this.dockerRegistryUsername);
}
/**
* @return The version of .NET to use. Possible values include `3.1`, `5.0`, `6.0`, `7.0` and `8.0`.
*
*/
public Optional dotnetVersion() {
return Optional.ofNullable(this.dotnetVersion);
}
/**
* @return The version of Go to use. Possible values include `1.18`, and `1.19`.
*
*/
public Optional goVersion() {
return Optional.ofNullable(this.goVersion);
}
/**
* @return The Java server type. Possible values include `JAVA`, `TOMCAT`, and `JBOSSEAP`.
*
* > **NOTE:** `JBOSSEAP` requires a Premium Service Plan SKU to be a valid option.
*
*/
public Optional javaServer() {
return Optional.ofNullable(this.javaServer);
}
/**
* @return The Version of the `java_server` to use.
*
*/
public Optional javaServerVersion() {
return Optional.ofNullable(this.javaServerVersion);
}
/**
* @return The Version of Java to use. Possible values include `8`, `11`, and `17`.
*
* > **NOTE:** The valid version combinations for `java_version`, `java_server` and `java_server_version` can be checked from the command line via `az webapp list-runtimes --linux`.
*
* > **NOTE:** `java_server`, `java_server_version`, and `java_version` must all be specified if building a java app
*
*/
public Optional javaVersion() {
return Optional.ofNullable(this.javaVersion);
}
/**
* @return The version of Node to run. Possible values include `12-lts`, `14-lts`, `16-lts`, `18-lts` and `20-lts`. This property conflicts with `java_version`.
*
* > **NOTE:** 10.x versions have been/are being deprecated so may cease to work for new resources in the future and may be removed from the provider.
*
*/
public Optional nodeVersion() {
return Optional.ofNullable(this.nodeVersion);
}
/**
* @return The version of PHP to run. Possible values are `7.4`, `8.0`, `8.1`, `8.2` and `8.3`.
*
* > **NOTE:** version `7.4` is deprecated and will be removed from the provider in a future version.
*
*/
public Optional phpVersion() {
return Optional.ofNullable(this.phpVersion);
}
/**
* @return The version of Python to run. Possible values include `3.7`, `3.8`, `3.9`, `3.10`, `3.11` and `3.12`.
*
*/
public Optional pythonVersion() {
return Optional.ofNullable(this.pythonVersion);
}
/**
* @return The version of Ruby to run. Possible values include `2.6` and `2.7`.
*
*/
public Optional rubyVersion() {
return Optional.ofNullable(this.rubyVersion);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(LinuxWebAppSiteConfigApplicationStack defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String dockerImageName;
private @Nullable String dockerRegistryPassword;
private @Nullable String dockerRegistryUrl;
private @Nullable String dockerRegistryUsername;
private @Nullable String dotnetVersion;
private @Nullable String goVersion;
private @Nullable String javaServer;
private @Nullable String javaServerVersion;
private @Nullable String javaVersion;
private @Nullable String nodeVersion;
private @Nullable String phpVersion;
private @Nullable String pythonVersion;
private @Nullable String rubyVersion;
public Builder() {}
public Builder(LinuxWebAppSiteConfigApplicationStack defaults) {
Objects.requireNonNull(defaults);
this.dockerImageName = defaults.dockerImageName;
this.dockerRegistryPassword = defaults.dockerRegistryPassword;
this.dockerRegistryUrl = defaults.dockerRegistryUrl;
this.dockerRegistryUsername = defaults.dockerRegistryUsername;
this.dotnetVersion = defaults.dotnetVersion;
this.goVersion = defaults.goVersion;
this.javaServer = defaults.javaServer;
this.javaServerVersion = defaults.javaServerVersion;
this.javaVersion = defaults.javaVersion;
this.nodeVersion = defaults.nodeVersion;
this.phpVersion = defaults.phpVersion;
this.pythonVersion = defaults.pythonVersion;
this.rubyVersion = defaults.rubyVersion;
}
@CustomType.Setter
public Builder dockerImageName(@Nullable String dockerImageName) {
this.dockerImageName = dockerImageName;
return this;
}
@CustomType.Setter
public Builder dockerRegistryPassword(@Nullable String dockerRegistryPassword) {
this.dockerRegistryPassword = dockerRegistryPassword;
return this;
}
@CustomType.Setter
public Builder dockerRegistryUrl(@Nullable String dockerRegistryUrl) {
this.dockerRegistryUrl = dockerRegistryUrl;
return this;
}
@CustomType.Setter
public Builder dockerRegistryUsername(@Nullable String dockerRegistryUsername) {
this.dockerRegistryUsername = dockerRegistryUsername;
return this;
}
@CustomType.Setter
public Builder dotnetVersion(@Nullable String dotnetVersion) {
this.dotnetVersion = dotnetVersion;
return this;
}
@CustomType.Setter
public Builder goVersion(@Nullable String goVersion) {
this.goVersion = goVersion;
return this;
}
@CustomType.Setter
public Builder javaServer(@Nullable String javaServer) {
this.javaServer = javaServer;
return this;
}
@CustomType.Setter
public Builder javaServerVersion(@Nullable String javaServerVersion) {
this.javaServerVersion = javaServerVersion;
return this;
}
@CustomType.Setter
public Builder javaVersion(@Nullable String javaVersion) {
this.javaVersion = javaVersion;
return this;
}
@CustomType.Setter
public Builder nodeVersion(@Nullable String nodeVersion) {
this.nodeVersion = nodeVersion;
return this;
}
@CustomType.Setter
public Builder phpVersion(@Nullable String phpVersion) {
this.phpVersion = phpVersion;
return this;
}
@CustomType.Setter
public Builder pythonVersion(@Nullable String pythonVersion) {
this.pythonVersion = pythonVersion;
return this;
}
@CustomType.Setter
public Builder rubyVersion(@Nullable String rubyVersion) {
this.rubyVersion = rubyVersion;
return this;
}
public LinuxWebAppSiteConfigApplicationStack build() {
final var _resultValue = new LinuxWebAppSiteConfigApplicationStack();
_resultValue.dockerImageName = dockerImageName;
_resultValue.dockerRegistryPassword = dockerRegistryPassword;
_resultValue.dockerRegistryUrl = dockerRegistryUrl;
_resultValue.dockerRegistryUsername = dockerRegistryUsername;
_resultValue.dotnetVersion = dotnetVersion;
_resultValue.goVersion = goVersion;
_resultValue.javaServer = javaServer;
_resultValue.javaServerVersion = javaServerVersion;
_resultValue.javaVersion = javaVersion;
_resultValue.nodeVersion = nodeVersion;
_resultValue.phpVersion = phpVersion;
_resultValue.pythonVersion = pythonVersion;
_resultValue.rubyVersion = rubyVersion;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy