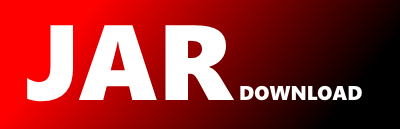
com.pulumi.azure.containerservice.inputs.KubernetesFleetManagerState Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.containerservice.inputs;
import com.pulumi.azure.containerservice.inputs.KubernetesFleetManagerHubProfileArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class KubernetesFleetManagerState extends com.pulumi.resources.ResourceArgs {
public static final KubernetesFleetManagerState Empty = new KubernetesFleetManagerState();
/**
* @deprecated
* The service team has indicated this field is now deprecated and not to be used, as such we are marking it as such and no longer sending it to the API, please see url: https://learn.microsoft.com/en-us/azure/kubernetes-fleet/architectural-overview
*
*/
@Deprecated /* The service team has indicated this field is now deprecated and not to be used, as such we are marking it as such and no longer sending it to the API, please see url: https://learn.microsoft.com/en-us/azure/kubernetes-fleet/architectural-overview */
@Import(name="hubProfile")
private @Nullable Output hubProfile;
/**
* @deprecated
* The service team has indicated this field is now deprecated and not to be used, as such we are marking it as such and no longer sending it to the API, please see url: https://learn.microsoft.com/en-us/azure/kubernetes-fleet/architectural-overview
*
*/
@Deprecated /* The service team has indicated this field is now deprecated and not to be used, as such we are marking it as such and no longer sending it to the API, please see url: https://learn.microsoft.com/en-us/azure/kubernetes-fleet/architectural-overview */
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy