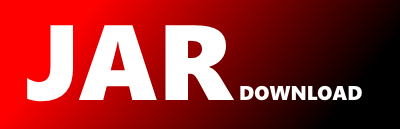
com.pulumi.azure.ProviderArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure Show documentation
Show all versions of azure Show documentation
A Pulumi package for creating and managing Microsoft Azure cloud resources, based on the Terraform azurerm provider. We recommend using the [Azure Native provider](https://github.com/pulumi/pulumi-azure-native) to provision Azure infrastructure. Azure Native provides complete coverage of Azure resources and same-day access to new resources and resource updates.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure;
import com.pulumi.azure.inputs.ProviderFeaturesArgs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ProviderArgs extends com.pulumi.resources.ResourceArgs {
public static final ProviderArgs Empty = new ProviderArgs();
@Import(name="auxiliaryTenantIds", json=true)
private @Nullable Output> auxiliaryTenantIds;
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy