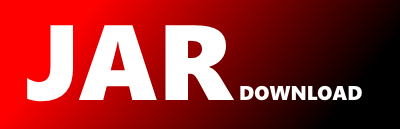
com.pulumi.azure.aadb2c.outputs.GetDirectoryResult Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.aadb2c.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
@CustomType
public final class GetDirectoryResult {
/**
* @return The type of billing for the AAD B2C tenant. Possible values include: `MAU` or `Auths`.
*
*/
private String billingType;
/**
* @return Location in which the B2C tenant is hosted and data resides. See [official docs](https://aka.ms/B2CDataResidenc) for more information.
*
*/
private String dataResidencyLocation;
private String domainName;
/**
* @return The date from which the billing type took effect. May not be populated until after the first billing cycle.
*
*/
private String effectiveStartDate;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
private String resourceGroupName;
/**
* @return Billing SKU for the B2C tenant. See [official docs](https://aka.ms/b2cBilling) for more information.
*
*/
private String skuName;
/**
* @return A mapping of tags assigned to the AAD B2C Directory.
*
*/
private Map tags;
/**
* @return The Tenant ID for the AAD B2C tenant.
*
*/
private String tenantId;
private GetDirectoryResult() {}
/**
* @return The type of billing for the AAD B2C tenant. Possible values include: `MAU` or `Auths`.
*
*/
public String billingType() {
return this.billingType;
}
/**
* @return Location in which the B2C tenant is hosted and data resides. See [official docs](https://aka.ms/B2CDataResidenc) for more information.
*
*/
public String dataResidencyLocation() {
return this.dataResidencyLocation;
}
public String domainName() {
return this.domainName;
}
/**
* @return The date from which the billing type took effect. May not be populated until after the first billing cycle.
*
*/
public String effectiveStartDate() {
return this.effectiveStartDate;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
public String resourceGroupName() {
return this.resourceGroupName;
}
/**
* @return Billing SKU for the B2C tenant. See [official docs](https://aka.ms/b2cBilling) for more information.
*
*/
public String skuName() {
return this.skuName;
}
/**
* @return A mapping of tags assigned to the AAD B2C Directory.
*
*/
public Map tags() {
return this.tags;
}
/**
* @return The Tenant ID for the AAD B2C tenant.
*
*/
public String tenantId() {
return this.tenantId;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetDirectoryResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private String billingType;
private String dataResidencyLocation;
private String domainName;
private String effectiveStartDate;
private String id;
private String resourceGroupName;
private String skuName;
private Map tags;
private String tenantId;
public Builder() {}
public Builder(GetDirectoryResult defaults) {
Objects.requireNonNull(defaults);
this.billingType = defaults.billingType;
this.dataResidencyLocation = defaults.dataResidencyLocation;
this.domainName = defaults.domainName;
this.effectiveStartDate = defaults.effectiveStartDate;
this.id = defaults.id;
this.resourceGroupName = defaults.resourceGroupName;
this.skuName = defaults.skuName;
this.tags = defaults.tags;
this.tenantId = defaults.tenantId;
}
@CustomType.Setter
public Builder billingType(String billingType) {
if (billingType == null) {
throw new MissingRequiredPropertyException("GetDirectoryResult", "billingType");
}
this.billingType = billingType;
return this;
}
@CustomType.Setter
public Builder dataResidencyLocation(String dataResidencyLocation) {
if (dataResidencyLocation == null) {
throw new MissingRequiredPropertyException("GetDirectoryResult", "dataResidencyLocation");
}
this.dataResidencyLocation = dataResidencyLocation;
return this;
}
@CustomType.Setter
public Builder domainName(String domainName) {
if (domainName == null) {
throw new MissingRequiredPropertyException("GetDirectoryResult", "domainName");
}
this.domainName = domainName;
return this;
}
@CustomType.Setter
public Builder effectiveStartDate(String effectiveStartDate) {
if (effectiveStartDate == null) {
throw new MissingRequiredPropertyException("GetDirectoryResult", "effectiveStartDate");
}
this.effectiveStartDate = effectiveStartDate;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetDirectoryResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder resourceGroupName(String resourceGroupName) {
if (resourceGroupName == null) {
throw new MissingRequiredPropertyException("GetDirectoryResult", "resourceGroupName");
}
this.resourceGroupName = resourceGroupName;
return this;
}
@CustomType.Setter
public Builder skuName(String skuName) {
if (skuName == null) {
throw new MissingRequiredPropertyException("GetDirectoryResult", "skuName");
}
this.skuName = skuName;
return this;
}
@CustomType.Setter
public Builder tags(Map tags) {
if (tags == null) {
throw new MissingRequiredPropertyException("GetDirectoryResult", "tags");
}
this.tags = tags;
return this;
}
@CustomType.Setter
public Builder tenantId(String tenantId) {
if (tenantId == null) {
throw new MissingRequiredPropertyException("GetDirectoryResult", "tenantId");
}
this.tenantId = tenantId;
return this;
}
public GetDirectoryResult build() {
final var _resultValue = new GetDirectoryResult();
_resultValue.billingType = billingType;
_resultValue.dataResidencyLocation = dataResidencyLocation;
_resultValue.domainName = domainName;
_resultValue.effectiveStartDate = effectiveStartDate;
_resultValue.id = id;
_resultValue.resourceGroupName = resourceGroupName;
_resultValue.skuName = skuName;
_resultValue.tags = tags;
_resultValue.tenantId = tenantId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy