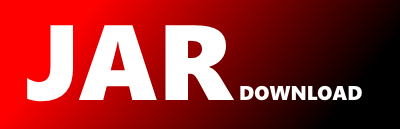
com.pulumi.azure.cognitive.AccountCustomerManagedKey Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure Show documentation
Show all versions of azure Show documentation
A Pulumi package for creating and managing Microsoft Azure cloud resources, based on the Terraform azurerm provider. We recommend using the [Azure Native provider](https://github.com/pulumi/pulumi-azure-native) to provision Azure infrastructure. Azure Native provides complete coverage of Azure resources and same-day access to new resources and resource updates.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.cognitive;
import com.pulumi.azure.Utilities;
import com.pulumi.azure.cognitive.AccountCustomerManagedKeyArgs;
import com.pulumi.azure.cognitive.inputs.AccountCustomerManagedKeyState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages a Customer Managed Key for a Cognitive Services Account.
*
* > **NOTE:** It's possible to define a Customer Managed Key both within the `azure.cognitive.Account` resource via the `customer_managed_key` block and by using the `azure.cognitive.AccountCustomerManagedKey` resource. However it's not possible to use both methods to manage a Customer Managed Key for a Cognitive Account, since there'll be conflicts.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.CoreFunctions;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.authorization.UserAssignedIdentity;
* import com.pulumi.azure.authorization.UserAssignedIdentityArgs;
* import com.pulumi.azure.cognitive.Account;
* import com.pulumi.azure.cognitive.AccountArgs;
* import com.pulumi.azure.cognitive.inputs.AccountIdentityArgs;
* import com.pulumi.azure.keyvault.KeyVault;
* import com.pulumi.azure.keyvault.KeyVaultArgs;
* import com.pulumi.azure.keyvault.inputs.KeyVaultAccessPolicyArgs;
* import com.pulumi.azure.keyvault.Key;
* import com.pulumi.azure.keyvault.KeyArgs;
* import com.pulumi.azure.cognitive.AccountCustomerManagedKey;
* import com.pulumi.azure.cognitive.AccountCustomerManagedKeyArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var current = CoreFunctions.getClientConfig();
*
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West US")
* .build());
*
* var exampleUserAssignedIdentity = new UserAssignedIdentity("exampleUserAssignedIdentity", UserAssignedIdentityArgs.builder()
* .resourceGroupName(example.name())
* .location(example.location())
* .name("example-identity")
* .build());
*
* var exampleAccount = new Account("exampleAccount", AccountArgs.builder()
* .name("example-account")
* .location(example.location())
* .resourceGroupName(example.name())
* .kind("Face")
* .skuName("E0")
* .customSubdomainName("example-account")
* .identity(AccountIdentityArgs.builder()
* .type("SystemAssigned, UserAssigned")
* .identityIds(exampleUserAssignedIdentity.id())
* .build())
* .build());
*
* var exampleKeyVault = new KeyVault("exampleKeyVault", KeyVaultArgs.builder()
* .name("example-vault")
* .location(example.location())
* .resourceGroupName(example.name())
* .tenantId(current.applyValue(getClientConfigResult -> getClientConfigResult.tenantId()))
* .skuName("standard")
* .purgeProtectionEnabled(true)
* .accessPolicies(
* KeyVaultAccessPolicyArgs.builder()
* .tenantId(exampleAccount.identity().applyValue(identity -> identity.tenantId()))
* .objectId(exampleAccount.identity().applyValue(identity -> identity.principalId()))
* .keyPermissions(
* "Get",
* "Create",
* "List",
* "Restore",
* "Recover",
* "UnwrapKey",
* "WrapKey",
* "Purge",
* "Encrypt",
* "Decrypt",
* "Sign",
* "Verify")
* .secretPermissions("Get")
* .build(),
* KeyVaultAccessPolicyArgs.builder()
* .tenantId(current.applyValue(getClientConfigResult -> getClientConfigResult.tenantId()))
* .objectId(current.applyValue(getClientConfigResult -> getClientConfigResult.objectId()))
* .keyPermissions(
* "Get",
* "Create",
* "Delete",
* "List",
* "Restore",
* "Recover",
* "UnwrapKey",
* "WrapKey",
* "Purge",
* "Encrypt",
* "Decrypt",
* "Sign",
* "Verify",
* "GetRotationPolicy")
* .secretPermissions("Get")
* .build(),
* KeyVaultAccessPolicyArgs.builder()
* .tenantId(exampleUserAssignedIdentity.tenantId())
* .objectId(exampleUserAssignedIdentity.principalId())
* .keyPermissions(
* "Get",
* "Create",
* "Delete",
* "List",
* "Restore",
* "Recover",
* "UnwrapKey",
* "WrapKey",
* "Purge",
* "Encrypt",
* "Decrypt",
* "Sign",
* "Verify")
* .secretPermissions("Get")
* .build())
* .build());
*
* var exampleKey = new Key("exampleKey", KeyArgs.builder()
* .name("example-key")
* .keyVaultId(exampleKeyVault.id())
* .keyType("RSA")
* .keySize(2048)
* .keyOpts(
* "decrypt",
* "encrypt",
* "sign",
* "unwrapKey",
* "verify",
* "wrapKey")
* .build());
*
* var exampleAccountCustomerManagedKey = new AccountCustomerManagedKey("exampleAccountCustomerManagedKey", AccountCustomerManagedKeyArgs.builder()
* .cognitiveAccountId(exampleAccount.id())
* .keyVaultKeyId(exampleKey.id())
* .identityClientId(exampleUserAssignedIdentity.clientId())
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Customer Managed Keys for a Cognitive Account can be imported using the `resource id`, e.g.
*
* ```sh
* $ pulumi import azure:cognitive/accountCustomerManagedKey:AccountCustomerManagedKey example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.CognitiveServices/accounts/account1
* ```
*
*/
@ResourceType(type="azure:cognitive/accountCustomerManagedKey:AccountCustomerManagedKey")
public class AccountCustomerManagedKey extends com.pulumi.resources.CustomResource {
/**
* The ID of the Cognitive Account. Changing this forces a new resource to be created.
*
*/
@Export(name="cognitiveAccountId", refs={String.class}, tree="[0]")
private Output cognitiveAccountId;
/**
* @return The ID of the Cognitive Account. Changing this forces a new resource to be created.
*
*/
public Output cognitiveAccountId() {
return this.cognitiveAccountId;
}
/**
* The Client ID of the User Assigned Identity that has access to the key. This property only needs to be specified when there're multiple identities attached to the Cognitive Account.
*
*/
@Export(name="identityClientId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> identityClientId;
/**
* @return The Client ID of the User Assigned Identity that has access to the key. This property only needs to be specified when there're multiple identities attached to the Cognitive Account.
*
*/
public Output> identityClientId() {
return Codegen.optional(this.identityClientId);
}
/**
* The ID of the Key Vault Key which should be used to Encrypt the data in this Cognitive Account.
*
*/
@Export(name="keyVaultKeyId", refs={String.class}, tree="[0]")
private Output keyVaultKeyId;
/**
* @return The ID of the Key Vault Key which should be used to Encrypt the data in this Cognitive Account.
*
*/
public Output keyVaultKeyId() {
return this.keyVaultKeyId;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public AccountCustomerManagedKey(java.lang.String name) {
this(name, AccountCustomerManagedKeyArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public AccountCustomerManagedKey(java.lang.String name, AccountCustomerManagedKeyArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public AccountCustomerManagedKey(java.lang.String name, AccountCustomerManagedKeyArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure:cognitive/accountCustomerManagedKey:AccountCustomerManagedKey", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private AccountCustomerManagedKey(java.lang.String name, Output id, @Nullable AccountCustomerManagedKeyState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure:cognitive/accountCustomerManagedKey:AccountCustomerManagedKey", name, state, makeResourceOptions(options, id), false);
}
private static AccountCustomerManagedKeyArgs makeArgs(AccountCustomerManagedKeyArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? AccountCustomerManagedKeyArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static AccountCustomerManagedKey get(java.lang.String name, Output id, @Nullable AccountCustomerManagedKeyState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new AccountCustomerManagedKey(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy