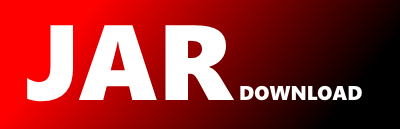
com.pulumi.azure.compute.LinuxVirtualMachineScaleSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure Show documentation
Show all versions of azure Show documentation
A Pulumi package for creating and managing Microsoft Azure cloud resources, based on the Terraform azurerm provider. We recommend using the [Azure Native provider](https://github.com/pulumi/pulumi-azure-native) to provision Azure infrastructure. Azure Native provides complete coverage of Azure resources and same-day access to new resources and resource updates.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.compute;
import com.pulumi.azure.Utilities;
import com.pulumi.azure.compute.LinuxVirtualMachineScaleSetArgs;
import com.pulumi.azure.compute.inputs.LinuxVirtualMachineScaleSetState;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetAdditionalCapabilities;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetAdminSshKey;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetAutomaticInstanceRepair;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetAutomaticOsUpgradePolicy;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetBootDiagnostics;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetDataDisk;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetExtension;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetGalleryApplication;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetIdentity;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetNetworkInterface;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetOsDisk;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetPlan;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetRollingUpgradePolicy;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetScaleIn;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetSecret;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetSourceImageReference;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetSpotRestore;
import com.pulumi.azure.compute.outputs.LinuxVirtualMachineScaleSetTerminationNotification;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.Double;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages a Linux Virtual Machine Scale Set.
*
* ## Disclaimers
*
* > **Note:** As of the **v2.86.0** (November 19, 2021) release of the provider this resource will only create Virtual Machine Scale Sets with the **Uniform** Orchestration Mode. For Virtual Machine Scale Sets with **Flexible** orchestration mode, use `azure.compute.OrchestratedVirtualMachineScaleSet`. Flexible orchestration mode is recommended for workloads on Azure.
* rraform will automatically update & reimage the nodes in the Scale Set (if Required) during an Update - this behaviour can be configured using the `features` setting within the Provider block.
*
* ## Example Usage
*
* This example provisions a basic Linux Virtual Machine Scale Set on an internal network.
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.network.VirtualNetwork;
* import com.pulumi.azure.network.VirtualNetworkArgs;
* import com.pulumi.azure.network.Subnet;
* import com.pulumi.azure.network.SubnetArgs;
* import com.pulumi.azure.compute.LinuxVirtualMachineScaleSet;
* import com.pulumi.azure.compute.LinuxVirtualMachineScaleSetArgs;
* import com.pulumi.azure.compute.inputs.LinuxVirtualMachineScaleSetAdminSshKeyArgs;
* import com.pulumi.azure.compute.inputs.LinuxVirtualMachineScaleSetSourceImageReferenceArgs;
* import com.pulumi.azure.compute.inputs.LinuxVirtualMachineScaleSetOsDiskArgs;
* import com.pulumi.azure.compute.inputs.LinuxVirtualMachineScaleSetNetworkInterfaceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App }{{@code
* public static void main(String[] args) }{{@code
* Pulumi.run(App::stack);
* }}{@code
*
* public static void stack(Context ctx) }{{@code
* final var firstPublicKey = "ssh-rsa AAAAB3NzaC1yc2EAAAADAQABAAABAQC+wWK73dCr+jgQOAxNsHAnNNNMEMWOHYEccp6wJm2gotpr9katuF/ZAdou5AaW1C61slRkHRkpRRX9FA9CYBiitZgvCCz+3nWNN7l/Up54Zps/pHWGZLHNJZRYyAB6j5yVLMVHIHriY49d/GZTZVNB8GoJv9Gakwc/fuEZYYl4YDFiGMBP///TzlI4jhiJzjKnEvqPFki5p2ZRJqcbCiF4pJrxUQR/RXqVFQdbRLZgYfJ8xGB878RENq3yQ39d8dVOkq4edbkzwcUmwwwkYVPIoDGsYLaRHnG+To7FvMeyO7xDVQkMKzopTQV8AuKpyvpqu0a9pWOMaiCyDytO7GGN you}{@literal @}{@code me.com";
*
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
*
* var exampleVirtualNetwork = new VirtualNetwork("exampleVirtualNetwork", VirtualNetworkArgs.builder()
* .name("example-network")
* .resourceGroupName(example.name())
* .location(example.location())
* .addressSpaces("10.0.0.0/16")
* .build());
*
* var internal = new Subnet("internal", SubnetArgs.builder()
* .name("internal")
* .resourceGroupName(example.name())
* .virtualNetworkName(exampleVirtualNetwork.name())
* .addressPrefixes("10.0.2.0/24")
* .build());
*
* var exampleLinuxVirtualMachineScaleSet = new LinuxVirtualMachineScaleSet("exampleLinuxVirtualMachineScaleSet", LinuxVirtualMachineScaleSetArgs.builder()
* .name("example-vmss")
* .resourceGroupName(example.name())
* .location(example.location())
* .sku("Standard_F2")
* .instances(1)
* .adminUsername("adminuser")
* .adminSshKeys(LinuxVirtualMachineScaleSetAdminSshKeyArgs.builder()
* .username("adminuser")
* .publicKey(firstPublicKey)
* .build())
* .sourceImageReference(LinuxVirtualMachineScaleSetSourceImageReferenceArgs.builder()
* .publisher("Canonical")
* .offer("0001-com-ubuntu-server-jammy")
* .sku("22_04-lts")
* .version("latest")
* .build())
* .osDisk(LinuxVirtualMachineScaleSetOsDiskArgs.builder()
* .storageAccountType("Standard_LRS")
* .caching("ReadWrite")
* .build())
* .networkInterfaces(LinuxVirtualMachineScaleSetNetworkInterfaceArgs.builder()
* .name("example")
* .primary(true)
* .ipConfigurations(LinuxVirtualMachineScaleSetNetworkInterfaceIpConfigurationArgs.builder()
* .name("internal")
* .primary(true)
* .subnetId(internal.id())
* .build())
* .build())
* .build());
*
* }}{@code
* }}{@code
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Linux Virtual Machine Scale Sets can be imported using the `resource id`, e.g.
*
* ```sh
* $ pulumi import azure:compute/linuxVirtualMachineScaleSet:LinuxVirtualMachineScaleSet example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/mygroup1/providers/Microsoft.Compute/virtualMachineScaleSets/scaleset1
* ```
*
*/
@ResourceType(type="azure:compute/linuxVirtualMachineScaleSet:LinuxVirtualMachineScaleSet")
public class LinuxVirtualMachineScaleSet extends com.pulumi.resources.CustomResource {
/**
* An `additional_capabilities` block as defined below.
*
*/
@Export(name="additionalCapabilities", refs={LinuxVirtualMachineScaleSetAdditionalCapabilities.class}, tree="[0]")
private Output* @Nullable */ LinuxVirtualMachineScaleSetAdditionalCapabilities> additionalCapabilities;
/**
* @return An `additional_capabilities` block as defined below.
*
*/
public Output> additionalCapabilities() {
return Codegen.optional(this.additionalCapabilities);
}
/**
* The Password which should be used for the local-administrator on this Virtual Machine. Changing this forces a new resource to be created.
*
* > **Note:** When an `admin_password` is specified `disable_password_authentication` must be set to `false`.
*
* > **Note:** One of either `admin_password` or `admin_ssh_key` must be specified.
*
*/
@Export(name="adminPassword", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> adminPassword;
/**
* @return The Password which should be used for the local-administrator on this Virtual Machine. Changing this forces a new resource to be created.
*
* > **Note:** When an `admin_password` is specified `disable_password_authentication` must be set to `false`.
*
* > **Note:** One of either `admin_password` or `admin_ssh_key` must be specified.
*
*/
public Output> adminPassword() {
return Codegen.optional(this.adminPassword);
}
/**
* One or more `admin_ssh_key` blocks as defined below.
*
* > **Note:** One of either `admin_password` or `admin_ssh_key` must be specified.
*
*/
@Export(name="adminSshKeys", refs={List.class,LinuxVirtualMachineScaleSetAdminSshKey.class}, tree="[0,1]")
private Output* @Nullable */ List> adminSshKeys;
/**
* @return One or more `admin_ssh_key` blocks as defined below.
*
* > **Note:** One of either `admin_password` or `admin_ssh_key` must be specified.
*
*/
public Output>> adminSshKeys() {
return Codegen.optional(this.adminSshKeys);
}
/**
* The username of the local administrator on each Virtual Machine Scale Set instance. Changing this forces a new resource to be created.
*
*/
@Export(name="adminUsername", refs={String.class}, tree="[0]")
private Output adminUsername;
/**
* @return The username of the local administrator on each Virtual Machine Scale Set instance. Changing this forces a new resource to be created.
*
*/
public Output adminUsername() {
return this.adminUsername;
}
/**
* An `automatic_instance_repair` block as defined below. To enable the automatic instance repair, this Virtual Machine Scale Set must have a valid `health_probe_id` or an [Application Health Extension](https://docs.microsoft.com/azure/virtual-machine-scale-sets/virtual-machine-scale-sets-health-extension).
*
* > **Note:** For more information about Automatic Instance Repair, please refer to the [product documentation](https://docs.microsoft.com/azure/virtual-machine-scale-sets/virtual-machine-scale-sets-automatic-instance-repairs).
*
*/
@Export(name="automaticInstanceRepair", refs={LinuxVirtualMachineScaleSetAutomaticInstanceRepair.class}, tree="[0]")
private Output automaticInstanceRepair;
/**
* @return An `automatic_instance_repair` block as defined below. To enable the automatic instance repair, this Virtual Machine Scale Set must have a valid `health_probe_id` or an [Application Health Extension](https://docs.microsoft.com/azure/virtual-machine-scale-sets/virtual-machine-scale-sets-health-extension).
*
* > **Note:** For more information about Automatic Instance Repair, please refer to the [product documentation](https://docs.microsoft.com/azure/virtual-machine-scale-sets/virtual-machine-scale-sets-automatic-instance-repairs).
*
*/
public Output automaticInstanceRepair() {
return this.automaticInstanceRepair;
}
/**
* An `automatic_os_upgrade_policy` block as defined below. This can only be specified when `upgrade_mode` is set to either `Automatic` or `Rolling`.
*
*/
@Export(name="automaticOsUpgradePolicy", refs={LinuxVirtualMachineScaleSetAutomaticOsUpgradePolicy.class}, tree="[0]")
private Output* @Nullable */ LinuxVirtualMachineScaleSetAutomaticOsUpgradePolicy> automaticOsUpgradePolicy;
/**
* @return An `automatic_os_upgrade_policy` block as defined below. This can only be specified when `upgrade_mode` is set to either `Automatic` or `Rolling`.
*
*/
public Output> automaticOsUpgradePolicy() {
return Codegen.optional(this.automaticOsUpgradePolicy);
}
/**
* A `boot_diagnostics` block as defined below.
*
*/
@Export(name="bootDiagnostics", refs={LinuxVirtualMachineScaleSetBootDiagnostics.class}, tree="[0]")
private Output* @Nullable */ LinuxVirtualMachineScaleSetBootDiagnostics> bootDiagnostics;
/**
* @return A `boot_diagnostics` block as defined below.
*
*/
public Output> bootDiagnostics() {
return Codegen.optional(this.bootDiagnostics);
}
/**
* Specifies the ID of the Capacity Reservation Group which the Virtual Machine Scale Set should be allocated to. Changing this forces a new resource to be created.
*
* > **Note:** `capacity_reservation_group_id` cannot be used with `proximity_placement_group_id`
*
* > **Note:** `single_placement_group` must be set to `false` when `capacity_reservation_group_id` is specified.
*
*/
@Export(name="capacityReservationGroupId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> capacityReservationGroupId;
/**
* @return Specifies the ID of the Capacity Reservation Group which the Virtual Machine Scale Set should be allocated to. Changing this forces a new resource to be created.
*
* > **Note:** `capacity_reservation_group_id` cannot be used with `proximity_placement_group_id`
*
* > **Note:** `single_placement_group` must be set to `false` when `capacity_reservation_group_id` is specified.
*
*/
public Output> capacityReservationGroupId() {
return Codegen.optional(this.capacityReservationGroupId);
}
/**
* The prefix which should be used for the name of the Virtual Machines in this Scale Set. If unspecified this defaults to the value for the `name` field. If the value of the `name` field is not a valid `computer_name_prefix`, then you must specify `computer_name_prefix`. Changing this forces a new resource to be created.
*
*/
@Export(name="computerNamePrefix", refs={String.class}, tree="[0]")
private Output computerNamePrefix;
/**
* @return The prefix which should be used for the name of the Virtual Machines in this Scale Set. If unspecified this defaults to the value for the `name` field. If the value of the `name` field is not a valid `computer_name_prefix`, then you must specify `computer_name_prefix`. Changing this forces a new resource to be created.
*
*/
public Output computerNamePrefix() {
return this.computerNamePrefix;
}
/**
* The Base64-Encoded Custom Data which should be used for this Virtual Machine Scale Set.
*
* > **Note:** When Custom Data has been configured, it's not possible to remove it without tainting the Virtual Machine Scale Set, due to a limitation of the Azure API.
*
*/
@Export(name="customData", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> customData;
/**
* @return The Base64-Encoded Custom Data which should be used for this Virtual Machine Scale Set.
*
* > **Note:** When Custom Data has been configured, it's not possible to remove it without tainting the Virtual Machine Scale Set, due to a limitation of the Azure API.
*
*/
public Output> customData() {
return Codegen.optional(this.customData);
}
/**
* One or more `data_disk` blocks as defined below.
*
*/
@Export(name="dataDisks", refs={List.class,LinuxVirtualMachineScaleSetDataDisk.class}, tree="[0,1]")
private Output* @Nullable */ List> dataDisks;
/**
* @return One or more `data_disk` blocks as defined below.
*
*/
public Output>> dataDisks() {
return Codegen.optional(this.dataDisks);
}
/**
* Should Password Authentication be disabled on this Virtual Machine Scale Set? Defaults to `true`.
*
* > In general we'd recommend using SSH Keys for authentication rather than Passwords - but there's tradeoff's to each - please [see this thread for more information](https://security.stackexchange.com/questions/69407/why-is-using-an-ssh-key-more-secure-than-using-passwords).
*
* > **Note:** When a `admin_password` is specified `disable_password_authentication` must be set to `false`.
*
*/
@Export(name="disablePasswordAuthentication", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> disablePasswordAuthentication;
/**
* @return Should Password Authentication be disabled on this Virtual Machine Scale Set? Defaults to `true`.
*
* > In general we'd recommend using SSH Keys for authentication rather than Passwords - but there's tradeoff's to each - please [see this thread for more information](https://security.stackexchange.com/questions/69407/why-is-using-an-ssh-key-more-secure-than-using-passwords).
*
* > **Note:** When a `admin_password` is specified `disable_password_authentication` must be set to `false`.
*
*/
public Output> disablePasswordAuthentication() {
return Codegen.optional(this.disablePasswordAuthentication);
}
/**
* Should Virtual Machine Extensions be run on Overprovisioned Virtual Machines in the Scale Set? Defaults to `false`.
*
*/
@Export(name="doNotRunExtensionsOnOverprovisionedMachines", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> doNotRunExtensionsOnOverprovisionedMachines;
/**
* @return Should Virtual Machine Extensions be run on Overprovisioned Virtual Machines in the Scale Set? Defaults to `false`.
*
*/
public Output> doNotRunExtensionsOnOverprovisionedMachines() {
return Codegen.optional(this.doNotRunExtensionsOnOverprovisionedMachines);
}
/**
* Specifies the Edge Zone within the Azure Region where this Linux Virtual Machine Scale Set should exist. Changing this forces a new Linux Virtual Machine Scale Set to be created.
*
*/
@Export(name="edgeZone", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> edgeZone;
/**
* @return Specifies the Edge Zone within the Azure Region where this Linux Virtual Machine Scale Set should exist. Changing this forces a new Linux Virtual Machine Scale Set to be created.
*
*/
public Output> edgeZone() {
return Codegen.optional(this.edgeZone);
}
/**
* Should all of the disks (including the temp disk) attached to this Virtual Machine be encrypted by enabling Encryption at Host?
*
*/
@Export(name="encryptionAtHostEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> encryptionAtHostEnabled;
/**
* @return Should all of the disks (including the temp disk) attached to this Virtual Machine be encrypted by enabling Encryption at Host?
*
*/
public Output> encryptionAtHostEnabled() {
return Codegen.optional(this.encryptionAtHostEnabled);
}
/**
* Specifies the eviction policy for Virtual Machines in this Scale Set. Possible values are `Deallocate` and `Delete`. Changing this forces a new resource to be created.
*
* > **Note:** This can only be configured when `priority` is set to `Spot`.
*
*/
@Export(name="evictionPolicy", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> evictionPolicy;
/**
* @return Specifies the eviction policy for Virtual Machines in this Scale Set. Possible values are `Deallocate` and `Delete`. Changing this forces a new resource to be created.
*
* > **Note:** This can only be configured when `priority` is set to `Spot`.
*
*/
public Output> evictionPolicy() {
return Codegen.optional(this.evictionPolicy);
}
/**
* Should extension operations be allowed on the Virtual Machine Scale Set? Possible values are `true` or `false`. Defaults to `true`. Changing this forces a new Linux Virtual Machine Scale Set to be created.
*
* > **Note:** `extension_operations_enabled` may only be set to `false` if there are no extensions defined in the `extension` field.
*
*/
@Export(name="extensionOperationsEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> extensionOperationsEnabled;
/**
* @return Should extension operations be allowed on the Virtual Machine Scale Set? Possible values are `true` or `false`. Defaults to `true`. Changing this forces a new Linux Virtual Machine Scale Set to be created.
*
* > **Note:** `extension_operations_enabled` may only be set to `false` if there are no extensions defined in the `extension` field.
*
*/
public Output> extensionOperationsEnabled() {
return Codegen.optional(this.extensionOperationsEnabled);
}
/**
* One or more `extension` blocks as defined below
*
*/
@Export(name="extensions", refs={List.class,LinuxVirtualMachineScaleSetExtension.class}, tree="[0,1]")
private Output> extensions;
/**
* @return One or more `extension` blocks as defined below
*
*/
public Output> extensions() {
return this.extensions;
}
/**
* Specifies the duration allocated for all extensions to start. The time duration should be between `15` minutes and `120` minutes (inclusive) and should be specified in ISO 8601 format. Defaults to `PT1H30M`.
*
*/
@Export(name="extensionsTimeBudget", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> extensionsTimeBudget;
/**
* @return Specifies the duration allocated for all extensions to start. The time duration should be between `15` minutes and `120` minutes (inclusive) and should be specified in ISO 8601 format. Defaults to `PT1H30M`.
*
*/
public Output> extensionsTimeBudget() {
return Codegen.optional(this.extensionsTimeBudget);
}
/**
* One or more `gallery_application` blocks as defined below.
*
*/
@Export(name="galleryApplications", refs={List.class,LinuxVirtualMachineScaleSetGalleryApplication.class}, tree="[0,1]")
private Output* @Nullable */ List> galleryApplications;
/**
* @return One or more `gallery_application` blocks as defined below.
*
*/
public Output>> galleryApplications() {
return Codegen.optional(this.galleryApplications);
}
/**
* The ID of a Load Balancer Probe which should be used to determine the health of an instance. This is Required and can only be specified when `upgrade_mode` is set to `Automatic` or `Rolling`.
*
*/
@Export(name="healthProbeId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> healthProbeId;
/**
* @return The ID of a Load Balancer Probe which should be used to determine the health of an instance. This is Required and can only be specified when `upgrade_mode` is set to `Automatic` or `Rolling`.
*
*/
public Output> healthProbeId() {
return Codegen.optional(this.healthProbeId);
}
/**
* Specifies the ID of the dedicated host group that the virtual machine scale set resides in. Changing this forces a new resource to be created.
*
*/
@Export(name="hostGroupId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> hostGroupId;
/**
* @return Specifies the ID of the dedicated host group that the virtual machine scale set resides in. Changing this forces a new resource to be created.
*
*/
public Output> hostGroupId() {
return Codegen.optional(this.hostGroupId);
}
/**
* An `identity` block as defined below.
*
*/
@Export(name="identity", refs={LinuxVirtualMachineScaleSetIdentity.class}, tree="[0]")
private Output* @Nullable */ LinuxVirtualMachineScaleSetIdentity> identity;
/**
* @return An `identity` block as defined below.
*
*/
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* The number of Virtual Machines in the Scale Set. Defaults to `0`.
*
* > **NOTE:** If you're using AutoScaling, you may wish to use [`Ignore Changes` functionality](https://www.pulumi.com/docs/intro/concepts/programming-model/#ignorechanges) to ignore changes to this field.
*
*/
@Export(name="instances", refs={Integer.class}, tree="[0]")
private Output* @Nullable */ Integer> instances;
/**
* @return The number of Virtual Machines in the Scale Set. Defaults to `0`.
*
* > **NOTE:** If you're using AutoScaling, you may wish to use [`Ignore Changes` functionality](https://www.pulumi.com/docs/intro/concepts/programming-model/#ignorechanges) to ignore changes to this field.
*
*/
public Output> instances() {
return Codegen.optional(this.instances);
}
/**
* The Azure location where the Linux Virtual Machine Scale Set should exist. Changing this forces a new resource to be created.
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return The Azure location where the Linux Virtual Machine Scale Set should exist. Changing this forces a new resource to be created.
*
*/
public Output location() {
return this.location;
}
/**
* The maximum price you're willing to pay for each Virtual Machine in this Scale Set, in US Dollars; which must be greater than the current spot price. If this bid price falls below the current spot price the Virtual Machines in the Scale Set will be evicted using the `eviction_policy`. Defaults to `-1`, which means that each Virtual Machine in this Scale Set should not be evicted for price reasons.
*
* > **Note:** This can only be configured when `priority` is set to `Spot`.
*
*/
@Export(name="maxBidPrice", refs={Double.class}, tree="[0]")
private Output* @Nullable */ Double> maxBidPrice;
/**
* @return The maximum price you're willing to pay for each Virtual Machine in this Scale Set, in US Dollars; which must be greater than the current spot price. If this bid price falls below the current spot price the Virtual Machines in the Scale Set will be evicted using the `eviction_policy`. Defaults to `-1`, which means that each Virtual Machine in this Scale Set should not be evicted for price reasons.
*
* > **Note:** This can only be configured when `priority` is set to `Spot`.
*
*/
public Output> maxBidPrice() {
return Codegen.optional(this.maxBidPrice);
}
/**
* The name of the Linux Virtual Machine Scale Set. Changing this forces a new resource to be created.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the Linux Virtual Machine Scale Set. Changing this forces a new resource to be created.
*
*/
public Output name() {
return this.name;
}
/**
* One or more `network_interface` blocks as defined below.
*
*/
@Export(name="networkInterfaces", refs={List.class,LinuxVirtualMachineScaleSetNetworkInterface.class}, tree="[0,1]")
private Output> networkInterfaces;
/**
* @return One or more `network_interface` blocks as defined below.
*
*/
public Output> networkInterfaces() {
return this.networkInterfaces;
}
/**
* An `os_disk` block as defined below.
*
*/
@Export(name="osDisk", refs={LinuxVirtualMachineScaleSetOsDisk.class}, tree="[0]")
private Output osDisk;
/**
* @return An `os_disk` block as defined below.
*
*/
public Output osDisk() {
return this.osDisk;
}
/**
* Should Azure over-provision Virtual Machines in this Scale Set? This means that multiple Virtual Machines will be provisioned and Azure will keep the instances which become available first - which improves provisioning success rates and improves deployment time. You're not billed for these over-provisioned VM's and they don't count towards the Subscription Quota. Defaults to `true`.
*
*/
@Export(name="overprovision", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> overprovision;
/**
* @return Should Azure over-provision Virtual Machines in this Scale Set? This means that multiple Virtual Machines will be provisioned and Azure will keep the instances which become available first - which improves provisioning success rates and improves deployment time. You're not billed for these over-provisioned VM's and they don't count towards the Subscription Quota. Defaults to `true`.
*
*/
public Output> overprovision() {
return Codegen.optional(this.overprovision);
}
/**
* A `plan` block as defined below. Changing this forces a new resource to be created.
*
* > **Note:** When using an image from Azure Marketplace a `plan` must be specified.
*
*/
@Export(name="plan", refs={LinuxVirtualMachineScaleSetPlan.class}, tree="[0]")
private Output* @Nullable */ LinuxVirtualMachineScaleSetPlan> plan;
/**
* @return A `plan` block as defined below. Changing this forces a new resource to be created.
*
* > **Note:** When using an image from Azure Marketplace a `plan` must be specified.
*
*/
public Output> plan() {
return Codegen.optional(this.plan);
}
/**
* Specifies the number of fault domains that are used by this Linux Virtual Machine Scale Set. Changing this forces a new resource to be created.
*
*/
@Export(name="platformFaultDomainCount", refs={Integer.class}, tree="[0]")
private Output platformFaultDomainCount;
/**
* @return Specifies the number of fault domains that are used by this Linux Virtual Machine Scale Set. Changing this forces a new resource to be created.
*
*/
public Output platformFaultDomainCount() {
return this.platformFaultDomainCount;
}
/**
* The Priority of this Virtual Machine Scale Set. Possible values are `Regular` and `Spot`. Defaults to `Regular`. Changing this value forces a new resource.
*
* > **Note:** When `priority` is set to `Spot` an `eviction_policy` must be specified.
*
*/
@Export(name="priority", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> priority;
/**
* @return The Priority of this Virtual Machine Scale Set. Possible values are `Regular` and `Spot`. Defaults to `Regular`. Changing this value forces a new resource.
*
* > **Note:** When `priority` is set to `Spot` an `eviction_policy` must be specified.
*
*/
public Output> priority() {
return Codegen.optional(this.priority);
}
/**
* Should the Azure VM Agent be provisioned on each Virtual Machine in the Scale Set? Defaults to `true`. Changing this value forces a new resource to be created.
*
*/
@Export(name="provisionVmAgent", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> provisionVmAgent;
/**
* @return Should the Azure VM Agent be provisioned on each Virtual Machine in the Scale Set? Defaults to `true`. Changing this value forces a new resource to be created.
*
*/
public Output> provisionVmAgent() {
return Codegen.optional(this.provisionVmAgent);
}
/**
* The ID of the Proximity Placement Group in which the Virtual Machine Scale Set should be assigned to. Changing this forces a new resource to be created.
*
*/
@Export(name="proximityPlacementGroupId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> proximityPlacementGroupId;
/**
* @return The ID of the Proximity Placement Group in which the Virtual Machine Scale Set should be assigned to. Changing this forces a new resource to be created.
*
*/
public Output> proximityPlacementGroupId() {
return Codegen.optional(this.proximityPlacementGroupId);
}
/**
* The name of the Resource Group in which the Linux Virtual Machine Scale Set should be exist. Changing this forces a new resource to be created.
*
*/
@Export(name="resourceGroupName", refs={String.class}, tree="[0]")
private Output resourceGroupName;
/**
* @return The name of the Resource Group in which the Linux Virtual Machine Scale Set should be exist. Changing this forces a new resource to be created.
*
*/
public Output resourceGroupName() {
return this.resourceGroupName;
}
/**
* A `rolling_upgrade_policy` block as defined below. This is Required and can only be specified when `upgrade_mode` is set to `Automatic` or `Rolling`. Changing this forces a new resource to be created.
*
*/
@Export(name="rollingUpgradePolicy", refs={LinuxVirtualMachineScaleSetRollingUpgradePolicy.class}, tree="[0]")
private Output* @Nullable */ LinuxVirtualMachineScaleSetRollingUpgradePolicy> rollingUpgradePolicy;
/**
* @return A `rolling_upgrade_policy` block as defined below. This is Required and can only be specified when `upgrade_mode` is set to `Automatic` or `Rolling`. Changing this forces a new resource to be created.
*
*/
public Output> rollingUpgradePolicy() {
return Codegen.optional(this.rollingUpgradePolicy);
}
/**
* A `scale_in` block as defined below.
*
*/
@Export(name="scaleIn", refs={LinuxVirtualMachineScaleSetScaleIn.class}, tree="[0]")
private Output* @Nullable */ LinuxVirtualMachineScaleSetScaleIn> scaleIn;
/**
* @return A `scale_in` block as defined below.
*
*/
public Output> scaleIn() {
return Codegen.optional(this.scaleIn);
}
/**
* One or more `secret` blocks as defined below.
*
*/
@Export(name="secrets", refs={List.class,LinuxVirtualMachineScaleSetSecret.class}, tree="[0,1]")
private Output* @Nullable */ List> secrets;
/**
* @return One or more `secret` blocks as defined below.
*
*/
public Output>> secrets() {
return Codegen.optional(this.secrets);
}
/**
* Specifies whether secure boot should be enabled on the virtual machine. Changing this forces a new resource to be created.
*
*/
@Export(name="secureBootEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> secureBootEnabled;
/**
* @return Specifies whether secure boot should be enabled on the virtual machine. Changing this forces a new resource to be created.
*
*/
public Output> secureBootEnabled() {
return Codegen.optional(this.secureBootEnabled);
}
/**
* Should this Virtual Machine Scale Set be limited to a Single Placement Group, which means the number of instances will be capped at 100 Virtual Machines. Defaults to `true`.
*
*/
@Export(name="singlePlacementGroup", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> singlePlacementGroup;
/**
* @return Should this Virtual Machine Scale Set be limited to a Single Placement Group, which means the number of instances will be capped at 100 Virtual Machines. Defaults to `true`.
*
*/
public Output> singlePlacementGroup() {
return Codegen.optional(this.singlePlacementGroup);
}
/**
* The Virtual Machine SKU for the Scale Set, such as `Standard_F2`.
*
*/
@Export(name="sku", refs={String.class}, tree="[0]")
private Output sku;
/**
* @return The Virtual Machine SKU for the Scale Set, such as `Standard_F2`.
*
*/
public Output sku() {
return this.sku;
}
/**
* The ID of an Image which each Virtual Machine in this Scale Set should be based on. Possible Image ID types include `Image ID`, `Shared Image ID`, `Shared Image Version ID`, `Community Gallery Image ID`, `Community Gallery Image Version ID`, `Shared Gallery Image ID` and `Shared Gallery Image Version ID`.
*
* > **Note:** One of either `source_image_id` or `source_image_reference` must be set.
*
*/
@Export(name="sourceImageId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> sourceImageId;
/**
* @return The ID of an Image which each Virtual Machine in this Scale Set should be based on. Possible Image ID types include `Image ID`, `Shared Image ID`, `Shared Image Version ID`, `Community Gallery Image ID`, `Community Gallery Image Version ID`, `Shared Gallery Image ID` and `Shared Gallery Image Version ID`.
*
* > **Note:** One of either `source_image_id` or `source_image_reference` must be set.
*
*/
public Output> sourceImageId() {
return Codegen.optional(this.sourceImageId);
}
/**
* A `source_image_reference` block as defined below.
*
* > **Note:** One of either `source_image_id` or `source_image_reference` must be set.
*
*/
@Export(name="sourceImageReference", refs={LinuxVirtualMachineScaleSetSourceImageReference.class}, tree="[0]")
private Output* @Nullable */ LinuxVirtualMachineScaleSetSourceImageReference> sourceImageReference;
/**
* @return A `source_image_reference` block as defined below.
*
* > **Note:** One of either `source_image_id` or `source_image_reference` must be set.
*
*/
public Output> sourceImageReference() {
return Codegen.optional(this.sourceImageReference);
}
/**
* A `spot_restore` block as defined below.
*
*/
@Export(name="spotRestore", refs={LinuxVirtualMachineScaleSetSpotRestore.class}, tree="[0]")
private Output spotRestore;
/**
* @return A `spot_restore` block as defined below.
*
*/
public Output spotRestore() {
return this.spotRestore;
}
/**
* A mapping of tags which should be assigned to this Virtual Machine Scale Set.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A mapping of tags which should be assigned to this Virtual Machine Scale Set.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* A `termination_notification` block as defined below.
*
*/
@Export(name="terminationNotification", refs={LinuxVirtualMachineScaleSetTerminationNotification.class}, tree="[0]")
private Output terminationNotification;
/**
* @return A `termination_notification` block as defined below.
*
*/
public Output terminationNotification() {
return this.terminationNotification;
}
/**
* The Unique ID for this Linux Virtual Machine Scale Set.
*
*/
@Export(name="uniqueId", refs={String.class}, tree="[0]")
private Output uniqueId;
/**
* @return The Unique ID for this Linux Virtual Machine Scale Set.
*
*/
public Output uniqueId() {
return this.uniqueId;
}
@Export(name="upgradeMode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> upgradeMode;
public Output> upgradeMode() {
return Codegen.optional(this.upgradeMode);
}
/**
* The Base64-Encoded User Data which should be used for this Virtual Machine Scale Set.
*
*/
@Export(name="userData", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> userData;
/**
* @return The Base64-Encoded User Data which should be used for this Virtual Machine Scale Set.
*
*/
public Output> userData() {
return Codegen.optional(this.userData);
}
/**
* Specifies whether vTPM should be enabled on the virtual machine. Changing this forces a new resource to be created.
*
*/
@Export(name="vtpmEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> vtpmEnabled;
/**
* @return Specifies whether vTPM should be enabled on the virtual machine. Changing this forces a new resource to be created.
*
*/
public Output> vtpmEnabled() {
return Codegen.optional(this.vtpmEnabled);
}
/**
* Should the Virtual Machines in this Scale Set be strictly evenly distributed across Availability Zones? Defaults to `false`. Changing this forces a new resource to be created.
*
* > **Note:** This can only be set to `true` when one or more `zones` are configured.
*
*/
@Export(name="zoneBalance", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> zoneBalance;
/**
* @return Should the Virtual Machines in this Scale Set be strictly evenly distributed across Availability Zones? Defaults to `false`. Changing this forces a new resource to be created.
*
* > **Note:** This can only be set to `true` when one or more `zones` are configured.
*
*/
public Output> zoneBalance() {
return Codegen.optional(this.zoneBalance);
}
/**
* Specifies a list of Availability Zones in which this Linux Virtual Machine Scale Set should be located.
*
* > **Note:** Updating `zones` to remove an existing zone forces a new Virtual Machine Scale Set to be created.
*
*/
@Export(name="zones", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> zones;
/**
* @return Specifies a list of Availability Zones in which this Linux Virtual Machine Scale Set should be located.
*
* > **Note:** Updating `zones` to remove an existing zone forces a new Virtual Machine Scale Set to be created.
*
*/
public Output>> zones() {
return Codegen.optional(this.zones);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public LinuxVirtualMachineScaleSet(java.lang.String name) {
this(name, LinuxVirtualMachineScaleSetArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public LinuxVirtualMachineScaleSet(java.lang.String name, LinuxVirtualMachineScaleSetArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public LinuxVirtualMachineScaleSet(java.lang.String name, LinuxVirtualMachineScaleSetArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure:compute/linuxVirtualMachineScaleSet:LinuxVirtualMachineScaleSet", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private LinuxVirtualMachineScaleSet(java.lang.String name, Output id, @Nullable LinuxVirtualMachineScaleSetState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure:compute/linuxVirtualMachineScaleSet:LinuxVirtualMachineScaleSet", name, state, makeResourceOptions(options, id), false);
}
private static LinuxVirtualMachineScaleSetArgs makeArgs(LinuxVirtualMachineScaleSetArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? LinuxVirtualMachineScaleSetArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.additionalSecretOutputs(List.of(
"adminPassword",
"customData"
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static LinuxVirtualMachineScaleSet get(java.lang.String name, Output id, @Nullable LinuxVirtualMachineScaleSetState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new LinuxVirtualMachineScaleSet(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy