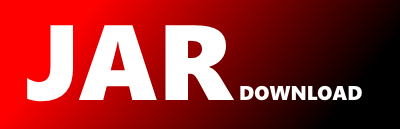
com.pulumi.azure.containerservice.outputs.KubernetesClusterIngressApplicationGateway Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure Show documentation
Show all versions of azure Show documentation
A Pulumi package for creating and managing Microsoft Azure cloud resources, based on the Terraform azurerm provider. We recommend using the [Azure Native provider](https://github.com/pulumi/pulumi-azure-native) to provision Azure infrastructure. Azure Native provides complete coverage of Azure resources and same-day access to new resources and resource updates.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.containerservice.outputs;
import com.pulumi.azure.containerservice.outputs.KubernetesClusterIngressApplicationGatewayIngressApplicationGatewayIdentity;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class KubernetesClusterIngressApplicationGateway {
/**
* @return The ID of the Application Gateway associated with the ingress controller deployed to this Kubernetes Cluster.
*
*/
private @Nullable String effectiveGatewayId;
/**
* @return The ID of the Application Gateway to integrate with the ingress controller of this Kubernetes Cluster. See [this](https://docs.microsoft.com/azure/application-gateway/tutorial-ingress-controller-add-on-existing) page for further details.
*
*/
private @Nullable String gatewayId;
/**
* @return The name of the Application Gateway to be used or created in the Nodepool Resource Group, which in turn will be integrated with the ingress controller of this Kubernetes Cluster. See [this](https://docs.microsoft.com/azure/application-gateway/tutorial-ingress-controller-add-on-new) page for further details.
*
*/
private @Nullable String gatewayName;
/**
* @return An `ingress_application_gateway_identity` block is exported. The exported attributes are defined below.
*
*/
private @Nullable List ingressApplicationGatewayIdentities;
/**
* @return The subnet CIDR to be used to create an Application Gateway, which in turn will be integrated with the ingress controller of this Kubernetes Cluster. See [this](https://docs.microsoft.com/azure/application-gateway/tutorial-ingress-controller-add-on-new) page for further details.
*
*/
private @Nullable String subnetCidr;
/**
* @return The ID of the subnet on which to create an Application Gateway, which in turn will be integrated with the ingress controller of this Kubernetes Cluster. See [this](https://docs.microsoft.com/azure/application-gateway/tutorial-ingress-controller-add-on-new) page for further details.
*
* > **Note:** Exactly one of `gateway_id`, `subnet_id` or `subnet_cidr` must be specified.
*
* > **Note:** If specifying `ingress_application_gateway` in conjunction with `only_critical_addons_enabled`, the AGIC pod will fail to start. A separate `azure.containerservice.KubernetesClusterNodePool` is required to run the AGIC pod successfully. This is because AGIC is classed as a "non-critical addon".
*
*/
private @Nullable String subnetId;
private KubernetesClusterIngressApplicationGateway() {}
/**
* @return The ID of the Application Gateway associated with the ingress controller deployed to this Kubernetes Cluster.
*
*/
public Optional effectiveGatewayId() {
return Optional.ofNullable(this.effectiveGatewayId);
}
/**
* @return The ID of the Application Gateway to integrate with the ingress controller of this Kubernetes Cluster. See [this](https://docs.microsoft.com/azure/application-gateway/tutorial-ingress-controller-add-on-existing) page for further details.
*
*/
public Optional gatewayId() {
return Optional.ofNullable(this.gatewayId);
}
/**
* @return The name of the Application Gateway to be used or created in the Nodepool Resource Group, which in turn will be integrated with the ingress controller of this Kubernetes Cluster. See [this](https://docs.microsoft.com/azure/application-gateway/tutorial-ingress-controller-add-on-new) page for further details.
*
*/
public Optional gatewayName() {
return Optional.ofNullable(this.gatewayName);
}
/**
* @return An `ingress_application_gateway_identity` block is exported. The exported attributes are defined below.
*
*/
public List ingressApplicationGatewayIdentities() {
return this.ingressApplicationGatewayIdentities == null ? List.of() : this.ingressApplicationGatewayIdentities;
}
/**
* @return The subnet CIDR to be used to create an Application Gateway, which in turn will be integrated with the ingress controller of this Kubernetes Cluster. See [this](https://docs.microsoft.com/azure/application-gateway/tutorial-ingress-controller-add-on-new) page for further details.
*
*/
public Optional subnetCidr() {
return Optional.ofNullable(this.subnetCidr);
}
/**
* @return The ID of the subnet on which to create an Application Gateway, which in turn will be integrated with the ingress controller of this Kubernetes Cluster. See [this](https://docs.microsoft.com/azure/application-gateway/tutorial-ingress-controller-add-on-new) page for further details.
*
* > **Note:** Exactly one of `gateway_id`, `subnet_id` or `subnet_cidr` must be specified.
*
* > **Note:** If specifying `ingress_application_gateway` in conjunction with `only_critical_addons_enabled`, the AGIC pod will fail to start. A separate `azure.containerservice.KubernetesClusterNodePool` is required to run the AGIC pod successfully. This is because AGIC is classed as a "non-critical addon".
*
*/
public Optional subnetId() {
return Optional.ofNullable(this.subnetId);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(KubernetesClusterIngressApplicationGateway defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String effectiveGatewayId;
private @Nullable String gatewayId;
private @Nullable String gatewayName;
private @Nullable List ingressApplicationGatewayIdentities;
private @Nullable String subnetCidr;
private @Nullable String subnetId;
public Builder() {}
public Builder(KubernetesClusterIngressApplicationGateway defaults) {
Objects.requireNonNull(defaults);
this.effectiveGatewayId = defaults.effectiveGatewayId;
this.gatewayId = defaults.gatewayId;
this.gatewayName = defaults.gatewayName;
this.ingressApplicationGatewayIdentities = defaults.ingressApplicationGatewayIdentities;
this.subnetCidr = defaults.subnetCidr;
this.subnetId = defaults.subnetId;
}
@CustomType.Setter
public Builder effectiveGatewayId(@Nullable String effectiveGatewayId) {
this.effectiveGatewayId = effectiveGatewayId;
return this;
}
@CustomType.Setter
public Builder gatewayId(@Nullable String gatewayId) {
this.gatewayId = gatewayId;
return this;
}
@CustomType.Setter
public Builder gatewayName(@Nullable String gatewayName) {
this.gatewayName = gatewayName;
return this;
}
@CustomType.Setter
public Builder ingressApplicationGatewayIdentities(@Nullable List ingressApplicationGatewayIdentities) {
this.ingressApplicationGatewayIdentities = ingressApplicationGatewayIdentities;
return this;
}
public Builder ingressApplicationGatewayIdentities(KubernetesClusterIngressApplicationGatewayIngressApplicationGatewayIdentity... ingressApplicationGatewayIdentities) {
return ingressApplicationGatewayIdentities(List.of(ingressApplicationGatewayIdentities));
}
@CustomType.Setter
public Builder subnetCidr(@Nullable String subnetCidr) {
this.subnetCidr = subnetCidr;
return this;
}
@CustomType.Setter
public Builder subnetId(@Nullable String subnetId) {
this.subnetId = subnetId;
return this;
}
public KubernetesClusterIngressApplicationGateway build() {
final var _resultValue = new KubernetesClusterIngressApplicationGateway();
_resultValue.effectiveGatewayId = effectiveGatewayId;
_resultValue.gatewayId = gatewayId;
_resultValue.gatewayName = gatewayName;
_resultValue.ingressApplicationGatewayIdentities = ingressApplicationGatewayIdentities;
_resultValue.subnetCidr = subnetCidr;
_resultValue.subnetId = subnetId;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy