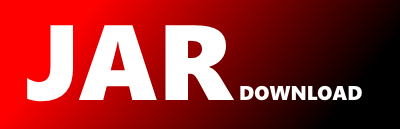
com.pulumi.azure.network.outputs.ApplicationGatewayBackendHttpSetting Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure Show documentation
Show all versions of azure Show documentation
A Pulumi package for creating and managing Microsoft Azure cloud resources, based on the Terraform azurerm provider. We recommend using the [Azure Native provider](https://github.com/pulumi/pulumi-azure-native) to provision Azure infrastructure. Azure Native provides complete coverage of Azure resources and same-day access to new resources and resource updates.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.network.outputs;
import com.pulumi.azure.network.outputs.ApplicationGatewayBackendHttpSettingAuthenticationCertificate;
import com.pulumi.azure.network.outputs.ApplicationGatewayBackendHttpSettingConnectionDraining;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class ApplicationGatewayBackendHttpSetting {
/**
* @return The name of the affinity cookie.
*
*/
private @Nullable String affinityCookieName;
/**
* @return One or more `authentication_certificate_backend` blocks as defined below.
*
*/
private @Nullable List authenticationCertificates;
/**
* @return A `connection_draining` block as defined below.
*
*/
private @Nullable ApplicationGatewayBackendHttpSettingConnectionDraining connectionDraining;
/**
* @return Is Cookie-Based Affinity enabled? Possible values are `Enabled` and `Disabled`.
*
*/
private String cookieBasedAffinity;
/**
* @return Host header to be sent to the backend servers. Cannot be set if `pick_host_name_from_backend_address` is set to `true`.
*
*/
private @Nullable String hostName;
/**
* @return The ID of the Rewrite Rule Set
*
*/
private @Nullable String id;
/**
* @return The name of the Authentication Certificate.
*
*/
private String name;
/**
* @return The Path which should be used as a prefix for all HTTP requests.
*
*/
private @Nullable String path;
/**
* @return Whether host header should be picked from the host name of the backend server. Defaults to `false`.
*
*/
private @Nullable Boolean pickHostNameFromBackendAddress;
/**
* @return The port which should be used for this Backend HTTP Settings Collection.
*
*/
private Integer port;
/**
* @return The ID of the associated Probe.
*
*/
private @Nullable String probeId;
/**
* @return The name of an associated HTTP Probe.
*
*/
private @Nullable String probeName;
/**
* @return The Protocol which should be used. Possible values are `Http` and `Https`.
*
*/
private String protocol;
/**
* @return The request timeout in seconds, which must be between 1 and 86400 seconds. Defaults to `30`.
*
*/
private @Nullable Integer requestTimeout;
/**
* @return A list of `trusted_root_certificate` names.
*
*/
private @Nullable List trustedRootCertificateNames;
private ApplicationGatewayBackendHttpSetting() {}
/**
* @return The name of the affinity cookie.
*
*/
public Optional affinityCookieName() {
return Optional.ofNullable(this.affinityCookieName);
}
/**
* @return One or more `authentication_certificate_backend` blocks as defined below.
*
*/
public List authenticationCertificates() {
return this.authenticationCertificates == null ? List.of() : this.authenticationCertificates;
}
/**
* @return A `connection_draining` block as defined below.
*
*/
public Optional connectionDraining() {
return Optional.ofNullable(this.connectionDraining);
}
/**
* @return Is Cookie-Based Affinity enabled? Possible values are `Enabled` and `Disabled`.
*
*/
public String cookieBasedAffinity() {
return this.cookieBasedAffinity;
}
/**
* @return Host header to be sent to the backend servers. Cannot be set if `pick_host_name_from_backend_address` is set to `true`.
*
*/
public Optional hostName() {
return Optional.ofNullable(this.hostName);
}
/**
* @return The ID of the Rewrite Rule Set
*
*/
public Optional id() {
return Optional.ofNullable(this.id);
}
/**
* @return The name of the Authentication Certificate.
*
*/
public String name() {
return this.name;
}
/**
* @return The Path which should be used as a prefix for all HTTP requests.
*
*/
public Optional path() {
return Optional.ofNullable(this.path);
}
/**
* @return Whether host header should be picked from the host name of the backend server. Defaults to `false`.
*
*/
public Optional pickHostNameFromBackendAddress() {
return Optional.ofNullable(this.pickHostNameFromBackendAddress);
}
/**
* @return The port which should be used for this Backend HTTP Settings Collection.
*
*/
public Integer port() {
return this.port;
}
/**
* @return The ID of the associated Probe.
*
*/
public Optional probeId() {
return Optional.ofNullable(this.probeId);
}
/**
* @return The name of an associated HTTP Probe.
*
*/
public Optional probeName() {
return Optional.ofNullable(this.probeName);
}
/**
* @return The Protocol which should be used. Possible values are `Http` and `Https`.
*
*/
public String protocol() {
return this.protocol;
}
/**
* @return The request timeout in seconds, which must be between 1 and 86400 seconds. Defaults to `30`.
*
*/
public Optional requestTimeout() {
return Optional.ofNullable(this.requestTimeout);
}
/**
* @return A list of `trusted_root_certificate` names.
*
*/
public List trustedRootCertificateNames() {
return this.trustedRootCertificateNames == null ? List.of() : this.trustedRootCertificateNames;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(ApplicationGatewayBackendHttpSetting defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String affinityCookieName;
private @Nullable List authenticationCertificates;
private @Nullable ApplicationGatewayBackendHttpSettingConnectionDraining connectionDraining;
private String cookieBasedAffinity;
private @Nullable String hostName;
private @Nullable String id;
private String name;
private @Nullable String path;
private @Nullable Boolean pickHostNameFromBackendAddress;
private Integer port;
private @Nullable String probeId;
private @Nullable String probeName;
private String protocol;
private @Nullable Integer requestTimeout;
private @Nullable List trustedRootCertificateNames;
public Builder() {}
public Builder(ApplicationGatewayBackendHttpSetting defaults) {
Objects.requireNonNull(defaults);
this.affinityCookieName = defaults.affinityCookieName;
this.authenticationCertificates = defaults.authenticationCertificates;
this.connectionDraining = defaults.connectionDraining;
this.cookieBasedAffinity = defaults.cookieBasedAffinity;
this.hostName = defaults.hostName;
this.id = defaults.id;
this.name = defaults.name;
this.path = defaults.path;
this.pickHostNameFromBackendAddress = defaults.pickHostNameFromBackendAddress;
this.port = defaults.port;
this.probeId = defaults.probeId;
this.probeName = defaults.probeName;
this.protocol = defaults.protocol;
this.requestTimeout = defaults.requestTimeout;
this.trustedRootCertificateNames = defaults.trustedRootCertificateNames;
}
@CustomType.Setter
public Builder affinityCookieName(@Nullable String affinityCookieName) {
this.affinityCookieName = affinityCookieName;
return this;
}
@CustomType.Setter
public Builder authenticationCertificates(@Nullable List authenticationCertificates) {
this.authenticationCertificates = authenticationCertificates;
return this;
}
public Builder authenticationCertificates(ApplicationGatewayBackendHttpSettingAuthenticationCertificate... authenticationCertificates) {
return authenticationCertificates(List.of(authenticationCertificates));
}
@CustomType.Setter
public Builder connectionDraining(@Nullable ApplicationGatewayBackendHttpSettingConnectionDraining connectionDraining) {
this.connectionDraining = connectionDraining;
return this;
}
@CustomType.Setter
public Builder cookieBasedAffinity(String cookieBasedAffinity) {
if (cookieBasedAffinity == null) {
throw new MissingRequiredPropertyException("ApplicationGatewayBackendHttpSetting", "cookieBasedAffinity");
}
this.cookieBasedAffinity = cookieBasedAffinity;
return this;
}
@CustomType.Setter
public Builder hostName(@Nullable String hostName) {
this.hostName = hostName;
return this;
}
@CustomType.Setter
public Builder id(@Nullable String id) {
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("ApplicationGatewayBackendHttpSetting", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder path(@Nullable String path) {
this.path = path;
return this;
}
@CustomType.Setter
public Builder pickHostNameFromBackendAddress(@Nullable Boolean pickHostNameFromBackendAddress) {
this.pickHostNameFromBackendAddress = pickHostNameFromBackendAddress;
return this;
}
@CustomType.Setter
public Builder port(Integer port) {
if (port == null) {
throw new MissingRequiredPropertyException("ApplicationGatewayBackendHttpSetting", "port");
}
this.port = port;
return this;
}
@CustomType.Setter
public Builder probeId(@Nullable String probeId) {
this.probeId = probeId;
return this;
}
@CustomType.Setter
public Builder probeName(@Nullable String probeName) {
this.probeName = probeName;
return this;
}
@CustomType.Setter
public Builder protocol(String protocol) {
if (protocol == null) {
throw new MissingRequiredPropertyException("ApplicationGatewayBackendHttpSetting", "protocol");
}
this.protocol = protocol;
return this;
}
@CustomType.Setter
public Builder requestTimeout(@Nullable Integer requestTimeout) {
this.requestTimeout = requestTimeout;
return this;
}
@CustomType.Setter
public Builder trustedRootCertificateNames(@Nullable List trustedRootCertificateNames) {
this.trustedRootCertificateNames = trustedRootCertificateNames;
return this;
}
public Builder trustedRootCertificateNames(String... trustedRootCertificateNames) {
return trustedRootCertificateNames(List.of(trustedRootCertificateNames));
}
public ApplicationGatewayBackendHttpSetting build() {
final var _resultValue = new ApplicationGatewayBackendHttpSetting();
_resultValue.affinityCookieName = affinityCookieName;
_resultValue.authenticationCertificates = authenticationCertificates;
_resultValue.connectionDraining = connectionDraining;
_resultValue.cookieBasedAffinity = cookieBasedAffinity;
_resultValue.hostName = hostName;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.path = path;
_resultValue.pickHostNameFromBackendAddress = pickHostNameFromBackendAddress;
_resultValue.port = port;
_resultValue.probeId = probeId;
_resultValue.probeName = probeName;
_resultValue.protocol = protocol;
_resultValue.requestTimeout = requestTimeout;
_resultValue.trustedRootCertificateNames = trustedRootCertificateNames;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy