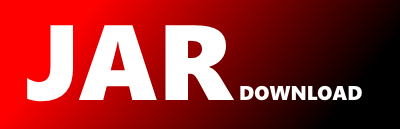
com.pulumi.azure.streamanalytics.ReferenceInputMssql Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure Show documentation
Show all versions of azure Show documentation
A Pulumi package for creating and managing Microsoft Azure cloud resources, based on the Terraform azurerm provider. We recommend using the [Azure Native provider](https://github.com/pulumi/pulumi-azure-native) to provision Azure infrastructure. Azure Native provides complete coverage of Azure resources and same-day access to new resources and resource updates.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.streamanalytics;
import com.pulumi.azure.Utilities;
import com.pulumi.azure.streamanalytics.ReferenceInputMssqlArgs;
import com.pulumi.azure.streamanalytics.inputs.ReferenceInputMssqlState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages a Stream Analytics Reference Input from MS SQL. Reference data (also known as a lookup table) is a finite data set that is static or slowly changing in nature, used to perform a lookup or to correlate with your data stream. Learn more [here](https://docs.microsoft.com/azure/stream-analytics/stream-analytics-use-reference-data#azure-sql-database).
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.streamanalytics.StreamanalyticsFunctions;
* import com.pulumi.azure.streamanalytics.inputs.GetJobArgs;
* import com.pulumi.azure.mssql.Server;
* import com.pulumi.azure.mssql.ServerArgs;
* import com.pulumi.azure.mssql.Database;
* import com.pulumi.azure.mssql.DatabaseArgs;
* import com.pulumi.azure.streamanalytics.ReferenceInputMssql;
* import com.pulumi.azure.streamanalytics.ReferenceInputMssqlArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var exampleResourceGroup = new ResourceGroup("exampleResourceGroup", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
*
* final var example = StreamanalyticsFunctions.getJob(GetJobArgs.builder()
* .name("example-job")
* .resourceGroupName(exampleResourceGroup.name())
* .build());
*
* var exampleServer = new Server("exampleServer", ServerArgs.builder()
* .name("example-sqlserver")
* .resourceGroupName(exampleResourceGroup.name())
* .location(exampleResourceGroup.location())
* .version("12.0")
* .administratorLogin("admin")
* .administratorLoginPassword("password")
* .build());
*
* var exampleDatabase = new Database("exampleDatabase", DatabaseArgs.builder()
* .name("example-db")
* .serverId(exampleServer.id())
* .build());
*
* var exampleReferenceInputMssql = new ReferenceInputMssql("exampleReferenceInputMssql", ReferenceInputMssqlArgs.builder()
* .name("example-reference-input")
* .resourceGroupName(example.applyValue(getJobResult -> getJobResult).applyValue(example -> example.applyValue(getJobResult -> getJobResult.resourceGroupName())))
* .streamAnalyticsJobName(example.applyValue(getJobResult -> getJobResult).applyValue(example -> example.applyValue(getJobResult -> getJobResult.name())))
* .server(exampleServer.fullyQualifiedDomainName())
* .database(exampleDatabase.name())
* .username("exampleuser")
* .password("examplepassword")
* .refreshType("RefreshPeriodicallyWithFull")
* .refreshIntervalDuration("00:20:00")
* .fullSnapshotQuery("""
* SELECT *
* INTO [YourOutputAlias]
* FROM [YourInputAlias]
* """)
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Stream Analytics can be imported using the `resource id`, e.g.
*
* ```sh
* $ pulumi import azure:streamanalytics/referenceInputMssql:ReferenceInputMssql example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.StreamAnalytics/streamingJobs/job1/inputs/input1
* ```
*
*/
@ResourceType(type="azure:streamanalytics/referenceInputMssql:ReferenceInputMssql")
public class ReferenceInputMssql extends com.pulumi.resources.CustomResource {
/**
* The MS SQL database name where the reference data exists.
*
*/
@Export(name="database", refs={String.class}, tree="[0]")
private Output database;
/**
* @return The MS SQL database name where the reference data exists.
*
*/
public Output database() {
return this.database;
}
/**
* The query used to retrieve incremental changes in the reference data from the MS SQL database. Cannot be set when `refresh_type` is `Static`.
*
*/
@Export(name="deltaSnapshotQuery", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> deltaSnapshotQuery;
/**
* @return The query used to retrieve incremental changes in the reference data from the MS SQL database. Cannot be set when `refresh_type` is `Static`.
*
*/
public Output> deltaSnapshotQuery() {
return Codegen.optional(this.deltaSnapshotQuery);
}
/**
* The query used to retrieve the reference data from the MS SQL database.
*
*/
@Export(name="fullSnapshotQuery", refs={String.class}, tree="[0]")
private Output fullSnapshotQuery;
/**
* @return The query used to retrieve the reference data from the MS SQL database.
*
*/
public Output fullSnapshotQuery() {
return this.fullSnapshotQuery;
}
/**
* The name of the Reference Input MS SQL data. Changing this forces a new resource to be created.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The name of the Reference Input MS SQL data. Changing this forces a new resource to be created.
*
*/
public Output name() {
return this.name;
}
/**
* The password to connect to the MS SQL database.
*
*/
@Export(name="password", refs={String.class}, tree="[0]")
private Output password;
/**
* @return The password to connect to the MS SQL database.
*
*/
public Output password() {
return this.password;
}
/**
* The frequency in `hh:mm:ss` with which the reference data should be retrieved from the MS SQL database e.g. `00:20:00` for every 20 minutes. Must be set when `refresh_type` is `RefreshPeriodicallyWithFull` or `RefreshPeriodicallyWithDelta`.
*
*/
@Export(name="refreshIntervalDuration", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> refreshIntervalDuration;
/**
* @return The frequency in `hh:mm:ss` with which the reference data should be retrieved from the MS SQL database e.g. `00:20:00` for every 20 minutes. Must be set when `refresh_type` is `RefreshPeriodicallyWithFull` or `RefreshPeriodicallyWithDelta`.
*
*/
public Output> refreshIntervalDuration() {
return Codegen.optional(this.refreshIntervalDuration);
}
/**
* Defines whether and how the reference data should be refreshed. Accepted values are `Static`, `RefreshPeriodicallyWithFull` and `RefreshPeriodicallyWithDelta`.
*
*/
@Export(name="refreshType", refs={String.class}, tree="[0]")
private Output refreshType;
/**
* @return Defines whether and how the reference data should be refreshed. Accepted values are `Static`, `RefreshPeriodicallyWithFull` and `RefreshPeriodicallyWithDelta`.
*
*/
public Output refreshType() {
return this.refreshType;
}
/**
* The name of the Resource Group where the Stream Analytics Job should exist. Changing this forces a new resource to be created.
*
*/
@Export(name="resourceGroupName", refs={String.class}, tree="[0]")
private Output resourceGroupName;
/**
* @return The name of the Resource Group where the Stream Analytics Job should exist. Changing this forces a new resource to be created.
*
*/
public Output resourceGroupName() {
return this.resourceGroupName;
}
/**
* The fully qualified domain name of the MS SQL server.
*
*/
@Export(name="server", refs={String.class}, tree="[0]")
private Output server;
/**
* @return The fully qualified domain name of the MS SQL server.
*
*/
public Output server() {
return this.server;
}
/**
* The name of the Stream Analytics Job. Changing this forces a new resource to be created.
*
*/
@Export(name="streamAnalyticsJobName", refs={String.class}, tree="[0]")
private Output streamAnalyticsJobName;
/**
* @return The name of the Stream Analytics Job. Changing this forces a new resource to be created.
*
*/
public Output streamAnalyticsJobName() {
return this.streamAnalyticsJobName;
}
/**
* The name of the table in the Azure SQL database.
*
*/
@Export(name="table", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> table;
/**
* @return The name of the table in the Azure SQL database.
*
*/
public Output> table() {
return Codegen.optional(this.table);
}
/**
* The username to connect to the MS SQL database.
*
*/
@Export(name="username", refs={String.class}, tree="[0]")
private Output username;
/**
* @return The username to connect to the MS SQL database.
*
*/
public Output username() {
return this.username;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public ReferenceInputMssql(java.lang.String name) {
this(name, ReferenceInputMssqlArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public ReferenceInputMssql(java.lang.String name, ReferenceInputMssqlArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public ReferenceInputMssql(java.lang.String name, ReferenceInputMssqlArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure:streamanalytics/referenceInputMssql:ReferenceInputMssql", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private ReferenceInputMssql(java.lang.String name, Output id, @Nullable ReferenceInputMssqlState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure:streamanalytics/referenceInputMssql:ReferenceInputMssql", name, state, makeResourceOptions(options, id), false);
}
private static ReferenceInputMssqlArgs makeArgs(ReferenceInputMssqlArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? ReferenceInputMssqlArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.additionalSecretOutputs(List.of(
"password"
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static ReferenceInputMssql get(java.lang.String name, Output id, @Nullable ReferenceInputMssqlState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new ReferenceInputMssql(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy