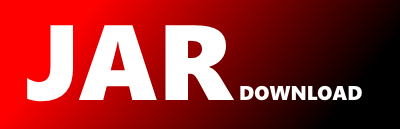
com.pulumi.azure.batch.Account Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure Show documentation
Show all versions of azure Show documentation
A Pulumi package for creating and managing Microsoft Azure cloud resources, based on the Terraform azurerm provider. We recommend using the [Azure Native provider](https://github.com/pulumi/pulumi-azure-native) to provision Azure infrastructure. Azure Native provides complete coverage of Azure resources and same-day access to new resources and resource updates.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.batch;
import com.pulumi.azure.Utilities;
import com.pulumi.azure.batch.AccountArgs;
import com.pulumi.azure.batch.inputs.AccountState;
import com.pulumi.azure.batch.outputs.AccountEncryption;
import com.pulumi.azure.batch.outputs.AccountIdentity;
import com.pulumi.azure.batch.outputs.AccountKeyVaultReference;
import com.pulumi.azure.batch.outputs.AccountNetworkProfile;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages an Azure Batch account.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.storage.Account;
* import com.pulumi.azure.storage.AccountArgs;
* import com.pulumi.azure.batch.Account;
* import com.pulumi.azure.batch.AccountArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("testbatch")
* .location("West Europe")
* .build());
*
* var exampleAccount = new Account("exampleAccount", AccountArgs.builder()
* .name("teststorage")
* .resourceGroupName(example.name())
* .location(example.location())
* .accountTier("Standard")
* .accountReplicationType("LRS")
* .build());
*
* var exampleAccount2 = new Account("exampleAccount2", AccountArgs.builder()
* .name("testbatchaccount")
* .resourceGroupName(example.name())
* .location(example.location())
* .poolAllocationMode("BatchService")
* .storageAccountId(exampleAccount.id())
* .storageAccountAuthenticationMode("StorageKeys")
* .tags(Map.of("env", "test"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Batch Account can be imported using the `resource id`, e.g.
*
* ```sh
* $ pulumi import azure:batch/account:Account example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/mygroup1/providers/Microsoft.Batch/batchAccounts/account1
* ```
*
*/
@ResourceType(type="azure:batch/account:Account")
public class Account extends com.pulumi.resources.CustomResource {
/**
* The account endpoint used to interact with the Batch service.
*
*/
@Export(name="accountEndpoint", refs={String.class}, tree="[0]")
private Output accountEndpoint;
/**
* @return The account endpoint used to interact with the Batch service.
*
*/
public Output accountEndpoint() {
return this.accountEndpoint;
}
/**
* Specifies the allowed authentication mode for the Batch account. Possible values include `AAD`, `SharedKey` or `TaskAuthenticationToken`.
*
*/
@Export(name="allowedAuthenticationModes", refs={List.class,String.class}, tree="[0,1]")
private Output> allowedAuthenticationModes;
/**
* @return Specifies the allowed authentication mode for the Batch account. Possible values include `AAD`, `SharedKey` or `TaskAuthenticationToken`.
*
*/
public Output> allowedAuthenticationModes() {
return this.allowedAuthenticationModes;
}
/**
* Specifies if customer managed key encryption should be used to encrypt batch account data. One `encryption` block as defined below.
*
*/
@Export(name="encryption", refs={AccountEncryption.class}, tree="[0]")
private Output* @Nullable */ AccountEncryption> encryption;
/**
* @return Specifies if customer managed key encryption should be used to encrypt batch account data. One `encryption` block as defined below.
*
*/
public Output> encryption() {
return Codegen.optional(this.encryption);
}
/**
* An `identity` block as defined below.
*
*/
@Export(name="identity", refs={AccountIdentity.class}, tree="[0]")
private Output* @Nullable */ AccountIdentity> identity;
/**
* @return An `identity` block as defined below.
*
*/
public Output> identity() {
return Codegen.optional(this.identity);
}
/**
* A `key_vault_reference` block, as defined below, that describes the Azure KeyVault reference to use when deploying the Azure Batch account using the `UserSubscription` pool allocation mode.
*
*/
@Export(name="keyVaultReference", refs={AccountKeyVaultReference.class}, tree="[0]")
private Output* @Nullable */ AccountKeyVaultReference> keyVaultReference;
/**
* @return A `key_vault_reference` block, as defined below, that describes the Azure KeyVault reference to use when deploying the Azure Batch account using the `UserSubscription` pool allocation mode.
*
*/
public Output> keyVaultReference() {
return Codegen.optional(this.keyVaultReference);
}
/**
* Specifies the supported Azure location where the resource exists. Changing this forces a new resource to be created.
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return Specifies the supported Azure location where the resource exists. Changing this forces a new resource to be created.
*
*/
public Output location() {
return this.location;
}
/**
* Specifies the name of the Batch account. Only lowercase Alphanumeric characters allowed. Changing this forces a new resource to be created.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Specifies the name of the Batch account. Only lowercase Alphanumeric characters allowed. Changing this forces a new resource to be created.
*
*/
public Output name() {
return this.name;
}
/**
* A `network_profile` block as defined below.
*
*/
@Export(name="networkProfile", refs={AccountNetworkProfile.class}, tree="[0]")
private Output* @Nullable */ AccountNetworkProfile> networkProfile;
/**
* @return A `network_profile` block as defined below.
*
*/
public Output> networkProfile() {
return Codegen.optional(this.networkProfile);
}
/**
* Specifies the mode to use for pool allocation. Possible values are `BatchService` or `UserSubscription`. Defaults to `BatchService`.
*
*/
@Export(name="poolAllocationMode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> poolAllocationMode;
/**
* @return Specifies the mode to use for pool allocation. Possible values are `BatchService` or `UserSubscription`. Defaults to `BatchService`.
*
*/
public Output> poolAllocationMode() {
return Codegen.optional(this.poolAllocationMode);
}
/**
* The Batch account primary access key.
*
*/
@Export(name="primaryAccessKey", refs={String.class}, tree="[0]")
private Output primaryAccessKey;
/**
* @return The Batch account primary access key.
*
*/
public Output primaryAccessKey() {
return this.primaryAccessKey;
}
/**
* Whether public network access is allowed for this server. Defaults to `true`.
*
* > **NOTE:** When using `UserSubscription` mode, an Azure KeyVault reference has to be specified. See `key_vault_reference` below.
*
* > **NOTE:** When using `UserSubscription` mode, the `Microsoft Azure Batch` service principal has to have `Contributor` role on your subscription scope, as documented [here](https://docs.microsoft.com/azure/batch/batch-account-create-portal#additional-configuration-for-user-subscription-mode).
*
*/
@Export(name="publicNetworkAccessEnabled", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> publicNetworkAccessEnabled;
/**
* @return Whether public network access is allowed for this server. Defaults to `true`.
*
* > **NOTE:** When using `UserSubscription` mode, an Azure KeyVault reference has to be specified. See `key_vault_reference` below.
*
* > **NOTE:** When using `UserSubscription` mode, the `Microsoft Azure Batch` service principal has to have `Contributor` role on your subscription scope, as documented [here](https://docs.microsoft.com/azure/batch/batch-account-create-portal#additional-configuration-for-user-subscription-mode).
*
*/
public Output> publicNetworkAccessEnabled() {
return Codegen.optional(this.publicNetworkAccessEnabled);
}
/**
* The name of the resource group in which to create the Batch account. Changing this forces a new resource to be created.
*
* > **NOTE:** To work around [a bug in the Azure API](https://github.com/Azure/azure-rest-api-specs/issues/5574) this property is currently treated as case-insensitive. A future version of this provider will require that the casing is correct.
*
*/
@Export(name="resourceGroupName", refs={String.class}, tree="[0]")
private Output resourceGroupName;
/**
* @return The name of the resource group in which to create the Batch account. Changing this forces a new resource to be created.
*
* > **NOTE:** To work around [a bug in the Azure API](https://github.com/Azure/azure-rest-api-specs/issues/5574) this property is currently treated as case-insensitive. A future version of this provider will require that the casing is correct.
*
*/
public Output resourceGroupName() {
return this.resourceGroupName;
}
/**
* The Batch account secondary access key.
*
*/
@Export(name="secondaryAccessKey", refs={String.class}, tree="[0]")
private Output secondaryAccessKey;
/**
* @return The Batch account secondary access key.
*
*/
public Output secondaryAccessKey() {
return this.secondaryAccessKey;
}
/**
* Specifies the storage account authentication mode. Possible values include `StorageKeys`, `BatchAccountManagedIdentity`.
*
* > **NOTE:** When using `BatchAccountManagedIdentity` mod, the `identity.type` must set to `UserAssigned` or `SystemAssigned`.
*
*/
@Export(name="storageAccountAuthenticationMode", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> storageAccountAuthenticationMode;
/**
* @return Specifies the storage account authentication mode. Possible values include `StorageKeys`, `BatchAccountManagedIdentity`.
*
* > **NOTE:** When using `BatchAccountManagedIdentity` mod, the `identity.type` must set to `UserAssigned` or `SystemAssigned`.
*
*/
public Output> storageAccountAuthenticationMode() {
return Codegen.optional(this.storageAccountAuthenticationMode);
}
/**
* Specifies the storage account to use for the Batch account. If not specified, Azure Batch will manage the storage.
*
* > **NOTE:** When using `storage_account_id`, the `storage_account_authentication_mode` must be specified as well.
*
*/
@Export(name="storageAccountId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> storageAccountId;
/**
* @return Specifies the storage account to use for the Batch account. If not specified, Azure Batch will manage the storage.
*
* > **NOTE:** When using `storage_account_id`, the `storage_account_authentication_mode` must be specified as well.
*
*/
public Output> storageAccountId() {
return Codegen.optional(this.storageAccountId);
}
/**
* Specifies the user assigned identity for the storage account.
*
*/
@Export(name="storageAccountNodeIdentity", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> storageAccountNodeIdentity;
/**
* @return Specifies the user assigned identity for the storage account.
*
*/
public Output> storageAccountNodeIdentity() {
return Codegen.optional(this.storageAccountNodeIdentity);
}
/**
* A mapping of tags to assign to the resource.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return A mapping of tags to assign to the resource.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Account(java.lang.String name) {
this(name, AccountArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Account(java.lang.String name, AccountArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Account(java.lang.String name, AccountArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure:batch/account:Account", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Account(java.lang.String name, Output id, @Nullable AccountState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure:batch/account:Account", name, state, makeResourceOptions(options, id), false);
}
private static AccountArgs makeArgs(AccountArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? AccountArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.additionalSecretOutputs(List.of(
"primaryAccessKey",
"secondaryAccessKey"
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Account get(java.lang.String name, Output id, @Nullable AccountState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Account(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy