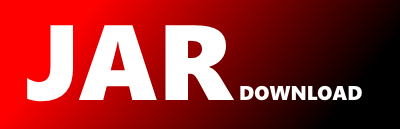
com.pulumi.azure.containerservice.outputs.GroupContainerVolume Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure Show documentation
Show all versions of azure Show documentation
A Pulumi package for creating and managing Microsoft Azure cloud resources, based on the Terraform azurerm provider. We recommend using the [Azure Native provider](https://github.com/pulumi/pulumi-azure-native) to provision Azure infrastructure. Azure Native provides complete coverage of Azure resources and same-day access to new resources and resource updates.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.containerservice.outputs;
import com.pulumi.azure.containerservice.outputs.GroupContainerVolumeGitRepo;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GroupContainerVolume {
/**
* @return Boolean as to whether the mounted volume should be an empty directory. Defaults to `false`. Changing this forces a new resource to be created.
*
*/
private @Nullable Boolean emptyDir;
/**
* @return A `git_repo` block as defined below. Changing this forces a new resource to be created.
*
*/
private @Nullable GroupContainerVolumeGitRepo gitRepo;
/**
* @return The path on which this volume is to be mounted. Changing this forces a new resource to be created.
*
*/
private String mountPath;
/**
* @return The name of the volume mount. Changing this forces a new resource to be created.
*
*/
private String name;
/**
* @return Specify if the volume is to be mounted as read only or not. The default value is `false`. Changing this forces a new resource to be created.
*
*/
private @Nullable Boolean readOnly;
/**
* @return A map of secrets that will be mounted as files in the volume. Changing this forces a new resource to be created.
*
* > **Note:** Exactly one of `empty_dir` volume, `git_repo` volume, `secret` volume or storage account volume (`share_name`, `storage_account_name`, and `storage_account_key`) must be specified.
*
* > **Note** when using a storage account volume, all of `share_name`, `storage_account_name`, and `storage_account_key` must be specified.
*
* > **Note:** The secret values must be supplied as Base64 encoded strings. The secret values are decoded to their original values when mounted in the volume on the container.
*
*/
private @Nullable Map secret;
/**
* @return The Azure storage share that is to be mounted as a volume. This must be created on the storage account specified as above. Changing this forces a new resource to be created.
*
*/
private @Nullable String shareName;
/**
* @return The access key for the Azure Storage account specified as above. Changing this forces a new resource to be created.
*
*/
private @Nullable String storageAccountKey;
/**
* @return The Azure storage account from which the volume is to be mounted. Changing this forces a new resource to be created.
*
*/
private @Nullable String storageAccountName;
private GroupContainerVolume() {}
/**
* @return Boolean as to whether the mounted volume should be an empty directory. Defaults to `false`. Changing this forces a new resource to be created.
*
*/
public Optional emptyDir() {
return Optional.ofNullable(this.emptyDir);
}
/**
* @return A `git_repo` block as defined below. Changing this forces a new resource to be created.
*
*/
public Optional gitRepo() {
return Optional.ofNullable(this.gitRepo);
}
/**
* @return The path on which this volume is to be mounted. Changing this forces a new resource to be created.
*
*/
public String mountPath() {
return this.mountPath;
}
/**
* @return The name of the volume mount. Changing this forces a new resource to be created.
*
*/
public String name() {
return this.name;
}
/**
* @return Specify if the volume is to be mounted as read only or not. The default value is `false`. Changing this forces a new resource to be created.
*
*/
public Optional readOnly() {
return Optional.ofNullable(this.readOnly);
}
/**
* @return A map of secrets that will be mounted as files in the volume. Changing this forces a new resource to be created.
*
* > **Note:** Exactly one of `empty_dir` volume, `git_repo` volume, `secret` volume or storage account volume (`share_name`, `storage_account_name`, and `storage_account_key`) must be specified.
*
* > **Note** when using a storage account volume, all of `share_name`, `storage_account_name`, and `storage_account_key` must be specified.
*
* > **Note:** The secret values must be supplied as Base64 encoded strings. The secret values are decoded to their original values when mounted in the volume on the container.
*
*/
public Map secret() {
return this.secret == null ? Map.of() : this.secret;
}
/**
* @return The Azure storage share that is to be mounted as a volume. This must be created on the storage account specified as above. Changing this forces a new resource to be created.
*
*/
public Optional shareName() {
return Optional.ofNullable(this.shareName);
}
/**
* @return The access key for the Azure Storage account specified as above. Changing this forces a new resource to be created.
*
*/
public Optional storageAccountKey() {
return Optional.ofNullable(this.storageAccountKey);
}
/**
* @return The Azure storage account from which the volume is to be mounted. Changing this forces a new resource to be created.
*
*/
public Optional storageAccountName() {
return Optional.ofNullable(this.storageAccountName);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GroupContainerVolume defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable Boolean emptyDir;
private @Nullable GroupContainerVolumeGitRepo gitRepo;
private String mountPath;
private String name;
private @Nullable Boolean readOnly;
private @Nullable Map secret;
private @Nullable String shareName;
private @Nullable String storageAccountKey;
private @Nullable String storageAccountName;
public Builder() {}
public Builder(GroupContainerVolume defaults) {
Objects.requireNonNull(defaults);
this.emptyDir = defaults.emptyDir;
this.gitRepo = defaults.gitRepo;
this.mountPath = defaults.mountPath;
this.name = defaults.name;
this.readOnly = defaults.readOnly;
this.secret = defaults.secret;
this.shareName = defaults.shareName;
this.storageAccountKey = defaults.storageAccountKey;
this.storageAccountName = defaults.storageAccountName;
}
@CustomType.Setter
public Builder emptyDir(@Nullable Boolean emptyDir) {
this.emptyDir = emptyDir;
return this;
}
@CustomType.Setter
public Builder gitRepo(@Nullable GroupContainerVolumeGitRepo gitRepo) {
this.gitRepo = gitRepo;
return this;
}
@CustomType.Setter
public Builder mountPath(String mountPath) {
if (mountPath == null) {
throw new MissingRequiredPropertyException("GroupContainerVolume", "mountPath");
}
this.mountPath = mountPath;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GroupContainerVolume", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder readOnly(@Nullable Boolean readOnly) {
this.readOnly = readOnly;
return this;
}
@CustomType.Setter
public Builder secret(@Nullable Map secret) {
this.secret = secret;
return this;
}
@CustomType.Setter
public Builder shareName(@Nullable String shareName) {
this.shareName = shareName;
return this;
}
@CustomType.Setter
public Builder storageAccountKey(@Nullable String storageAccountKey) {
this.storageAccountKey = storageAccountKey;
return this;
}
@CustomType.Setter
public Builder storageAccountName(@Nullable String storageAccountName) {
this.storageAccountName = storageAccountName;
return this;
}
public GroupContainerVolume build() {
final var _resultValue = new GroupContainerVolume();
_resultValue.emptyDir = emptyDir;
_resultValue.gitRepo = gitRepo;
_resultValue.mountPath = mountPath;
_resultValue.name = name;
_resultValue.readOnly = readOnly;
_resultValue.secret = secret;
_resultValue.shareName = shareName;
_resultValue.storageAccountKey = storageAccountKey;
_resultValue.storageAccountName = storageAccountName;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy