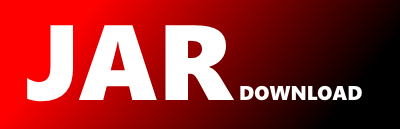
com.pulumi.azure.datafactory.LinkedServiceAzureFunction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure Show documentation
Show all versions of azure Show documentation
A Pulumi package for creating and managing Microsoft Azure cloud resources, based on the Terraform azurerm provider. We recommend using the [Azure Native provider](https://github.com/pulumi/pulumi-azure-native) to provision Azure infrastructure. Azure Native provides complete coverage of Azure resources and same-day access to new resources and resource updates.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.datafactory;
import com.pulumi.azure.Utilities;
import com.pulumi.azure.datafactory.LinkedServiceAzureFunctionArgs;
import com.pulumi.azure.datafactory.inputs.LinkedServiceAzureFunctionState;
import com.pulumi.azure.datafactory.outputs.LinkedServiceAzureFunctionKeyVaultKey;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages a Linked Service (connection) between an Azure Function and Azure Data Factory.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.appservice.AppserviceFunctions;
* import com.pulumi.azure.appservice.inputs.GetFunctionAppArgs;
* import com.pulumi.azure.datafactory.Factory;
* import com.pulumi.azure.datafactory.FactoryArgs;
* import com.pulumi.azure.datafactory.LinkedServiceAzureFunction;
* import com.pulumi.azure.datafactory.LinkedServiceAzureFunctionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var exampleResourceGroup = new ResourceGroup("exampleResourceGroup", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
*
* final var example = AppserviceFunctions.getFunctionApp(GetFunctionAppArgs.builder()
* .name("test-azure-functions")
* .resourceGroupName(exampleResourceGroup.name())
* .build());
*
* var exampleFactory = new Factory("exampleFactory", FactoryArgs.builder()
* .name("example")
* .location(exampleResourceGroup.location())
* .resourceGroupName(exampleResourceGroup.name())
* .build());
*
* var exampleLinkedServiceAzureFunction = new LinkedServiceAzureFunction("exampleLinkedServiceAzureFunction", LinkedServiceAzureFunctionArgs.builder()
* .name("example")
* .dataFactoryId(exampleFactory.id())
* .url(example.applyValue(getFunctionAppResult -> getFunctionAppResult).applyValue(example -> String.format("https://%s", example.applyValue(getFunctionAppResult -> getFunctionAppResult.defaultHostname()))))
* .key("foo")
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Data Factory Linked Service's can be imported using the `resource id`, e.g.
*
* ```sh
* $ pulumi import azure:datafactory/linkedServiceAzureFunction:LinkedServiceAzureFunction example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/example/providers/Microsoft.DataFactory/factories/example/linkedservices/example
* ```
*
*/
@ResourceType(type="azure:datafactory/linkedServiceAzureFunction:LinkedServiceAzureFunction")
public class LinkedServiceAzureFunction extends com.pulumi.resources.CustomResource {
/**
* A map of additional properties to associate with the Data Factory Linked Service.
*
* The following supported arguments are specific to Azure Function Linked Service:
*
*/
@Export(name="additionalProperties", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> additionalProperties;
/**
* @return A map of additional properties to associate with the Data Factory Linked Service.
*
* The following supported arguments are specific to Azure Function Linked Service:
*
*/
public Output>> additionalProperties() {
return Codegen.optional(this.additionalProperties);
}
/**
* List of tags that can be used for describing the Data Factory Linked Service.
*
*/
@Export(name="annotations", refs={List.class,String.class}, tree="[0,1]")
private Output* @Nullable */ List> annotations;
/**
* @return List of tags that can be used for describing the Data Factory Linked Service.
*
*/
public Output>> annotations() {
return Codegen.optional(this.annotations);
}
/**
* The Data Factory ID in which to associate the Linked Service with. Changing this forces a new resource.
*
*/
@Export(name="dataFactoryId", refs={String.class}, tree="[0]")
private Output dataFactoryId;
/**
* @return The Data Factory ID in which to associate the Linked Service with. Changing this forces a new resource.
*
*/
public Output dataFactoryId() {
return this.dataFactoryId;
}
/**
* The description for the Data Factory Linked Service.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return The description for the Data Factory Linked Service.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* The integration runtime reference to associate with the Data Factory Linked Service.
*
*/
@Export(name="integrationRuntimeName", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> integrationRuntimeName;
/**
* @return The integration runtime reference to associate with the Data Factory Linked Service.
*
*/
public Output> integrationRuntimeName() {
return Codegen.optional(this.integrationRuntimeName);
}
/**
* The system key of the Azure Function. Exactly one of either `key` or `key_vault_key` is required
*
*/
@Export(name="key", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> key;
/**
* @return The system key of the Azure Function. Exactly one of either `key` or `key_vault_key` is required
*
*/
public Output> key() {
return Codegen.optional(this.key);
}
/**
* A `key_vault_key` block as defined below. Use this Argument to store the system key of the Azure Function in an existing Key Vault. It needs an existing Key Vault Data Factory Linked Service. Exactly one of either `key` or `key_vault_key` is required.
*
*/
@Export(name="keyVaultKey", refs={LinkedServiceAzureFunctionKeyVaultKey.class}, tree="[0]")
private Output* @Nullable */ LinkedServiceAzureFunctionKeyVaultKey> keyVaultKey;
/**
* @return A `key_vault_key` block as defined below. Use this Argument to store the system key of the Azure Function in an existing Key Vault. It needs an existing Key Vault Data Factory Linked Service. Exactly one of either `key` or `key_vault_key` is required.
*
*/
public Output> keyVaultKey() {
return Codegen.optional(this.keyVaultKey);
}
/**
* Specifies the name of the Data Factory Linked Service. Changing this forces a new resource to be created. Must be unique within a data factory. See the [Microsoft documentation](https://docs.microsoft.com/azure/data-factory/naming-rules) for all restrictions.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Specifies the name of the Data Factory Linked Service. Changing this forces a new resource to be created. Must be unique within a data factory. See the [Microsoft documentation](https://docs.microsoft.com/azure/data-factory/naming-rules) for all restrictions.
*
*/
public Output name() {
return this.name;
}
/**
* A map of parameters to associate with the Data Factory Linked Service.
*
*/
@Export(name="parameters", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> parameters;
/**
* @return A map of parameters to associate with the Data Factory Linked Service.
*
*/
public Output>> parameters() {
return Codegen.optional(this.parameters);
}
/**
* The url of the Azure Function.
*
*/
@Export(name="url", refs={String.class}, tree="[0]")
private Output url;
/**
* @return The url of the Azure Function.
*
*/
public Output url() {
return this.url;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public LinkedServiceAzureFunction(java.lang.String name) {
this(name, LinkedServiceAzureFunctionArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public LinkedServiceAzureFunction(java.lang.String name, LinkedServiceAzureFunctionArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public LinkedServiceAzureFunction(java.lang.String name, LinkedServiceAzureFunctionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure:datafactory/linkedServiceAzureFunction:LinkedServiceAzureFunction", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private LinkedServiceAzureFunction(java.lang.String name, Output id, @Nullable LinkedServiceAzureFunctionState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("azure:datafactory/linkedServiceAzureFunction:LinkedServiceAzureFunction", name, state, makeResourceOptions(options, id), false);
}
private static LinkedServiceAzureFunctionArgs makeArgs(LinkedServiceAzureFunctionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? LinkedServiceAzureFunctionArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.additionalSecretOutputs(List.of(
"key"
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static LinkedServiceAzureFunction get(java.lang.String name, Output id, @Nullable LinkedServiceAzureFunctionState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new LinkedServiceAzureFunction(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy