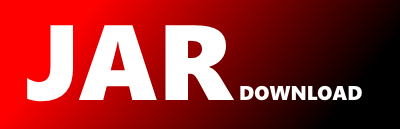
com.pulumi.azure.datafactory.outputs.IntegrationRuntimeSsisExpressCustomSetup Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure Show documentation
Show all versions of azure Show documentation
A Pulumi package for creating and managing Microsoft Azure cloud resources, based on the Terraform azurerm provider. We recommend using the [Azure Native provider](https://github.com/pulumi/pulumi-azure-native) to provision Azure infrastructure. Azure Native provides complete coverage of Azure resources and same-day access to new resources and resource updates.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.datafactory.outputs;
import com.pulumi.azure.datafactory.outputs.IntegrationRuntimeSsisExpressCustomSetupCommandKey;
import com.pulumi.azure.datafactory.outputs.IntegrationRuntimeSsisExpressCustomSetupComponent;
import com.pulumi.core.annotations.CustomType;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class IntegrationRuntimeSsisExpressCustomSetup {
/**
* @return One or more `command_key` blocks as defined below.
*
*/
private @Nullable List commandKeys;
/**
* @return One or more `component` blocks as defined below.
*
*/
private @Nullable List components;
/**
* @return The Environment Variables for the Azure-SSIS Integration Runtime.
*
*/
private @Nullable Map environment;
/**
* @return The version of Azure Powershell installed for the Azure-SSIS Integration Runtime.
*
* > **NOTE** At least one of `env`, `powershell_version`, `component` and `command_key` should be specified.
*
*/
private @Nullable String powershellVersion;
private IntegrationRuntimeSsisExpressCustomSetup() {}
/**
* @return One or more `command_key` blocks as defined below.
*
*/
public List commandKeys() {
return this.commandKeys == null ? List.of() : this.commandKeys;
}
/**
* @return One or more `component` blocks as defined below.
*
*/
public List components() {
return this.components == null ? List.of() : this.components;
}
/**
* @return The Environment Variables for the Azure-SSIS Integration Runtime.
*
*/
public Map environment() {
return this.environment == null ? Map.of() : this.environment;
}
/**
* @return The version of Azure Powershell installed for the Azure-SSIS Integration Runtime.
*
* > **NOTE** At least one of `env`, `powershell_version`, `component` and `command_key` should be specified.
*
*/
public Optional powershellVersion() {
return Optional.ofNullable(this.powershellVersion);
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(IntegrationRuntimeSsisExpressCustomSetup defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable List commandKeys;
private @Nullable List components;
private @Nullable Map environment;
private @Nullable String powershellVersion;
public Builder() {}
public Builder(IntegrationRuntimeSsisExpressCustomSetup defaults) {
Objects.requireNonNull(defaults);
this.commandKeys = defaults.commandKeys;
this.components = defaults.components;
this.environment = defaults.environment;
this.powershellVersion = defaults.powershellVersion;
}
@CustomType.Setter
public Builder commandKeys(@Nullable List commandKeys) {
this.commandKeys = commandKeys;
return this;
}
public Builder commandKeys(IntegrationRuntimeSsisExpressCustomSetupCommandKey... commandKeys) {
return commandKeys(List.of(commandKeys));
}
@CustomType.Setter
public Builder components(@Nullable List components) {
this.components = components;
return this;
}
public Builder components(IntegrationRuntimeSsisExpressCustomSetupComponent... components) {
return components(List.of(components));
}
@CustomType.Setter
public Builder environment(@Nullable Map environment) {
this.environment = environment;
return this;
}
@CustomType.Setter
public Builder powershellVersion(@Nullable String powershellVersion) {
this.powershellVersion = powershellVersion;
return this;
}
public IntegrationRuntimeSsisExpressCustomSetup build() {
final var _resultValue = new IntegrationRuntimeSsisExpressCustomSetup();
_resultValue.commandKeys = commandKeys;
_resultValue.components = components;
_resultValue.environment = environment;
_resultValue.powershellVersion = powershellVersion;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy