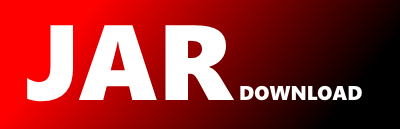
com.pulumi.azure.managedapplication.Application Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure Show documentation
Show all versions of azure Show documentation
A Pulumi package for creating and managing Microsoft Azure cloud resources, based on the Terraform azurerm provider. We recommend using the [Azure Native provider](https://github.com/pulumi/pulumi-azure-native) to provision Azure infrastructure. Azure Native provides complete coverage of Azure resources and same-day access to new resources and resource updates.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.managedapplication;
import com.pulumi.azure.Utilities;
import com.pulumi.azure.managedapplication.ApplicationArgs;
import com.pulumi.azure.managedapplication.inputs.ApplicationState;
import com.pulumi.azure.managedapplication.outputs.ApplicationPlan;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* Manages a Managed Application.
*
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.CoreFunctions;
* import com.pulumi.azure.authorization.AuthorizationFunctions;
* import com.pulumi.azure.authorization.inputs.GetRoleDefinitionArgs;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.managedapplication.Definition;
* import com.pulumi.azure.managedapplication.DefinitionArgs;
* import com.pulumi.azure.managedapplication.inputs.DefinitionAuthorizationArgs;
* import com.pulumi.azure.managedapplication.Application;
* import com.pulumi.azure.managedapplication.ApplicationArgs;
* import static com.pulumi.codegen.internal.Serialization.*;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* final var current = CoreFunctions.getClientConfig();
*
* final var builtin = AuthorizationFunctions.getRoleDefinition(GetRoleDefinitionArgs.builder()
* .name("Contributor")
* .build());
*
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
*
* var exampleDefinition = new Definition("exampleDefinition", DefinitionArgs.builder()
* .name("examplemanagedapplicationdefinition")
* .location(example.location())
* .resourceGroupName(example.name())
* .lockLevel("ReadOnly")
* .packageFileUri("https://github.com/Azure/azure-managedapp-samples/raw/master/Managed Application Sample Packages/201-managed-storage-account/managedstorage.zip")
* .displayName("TestManagedAppDefinition")
* .description("Test Managed App Definition")
* .authorizations(DefinitionAuthorizationArgs.builder()
* .servicePrincipalId(current.applyValue(getClientConfigResult -> getClientConfigResult.objectId()))
* .roleDefinitionId(StdFunctions.split(SplitArgs.builder()
* .separator("/")
* .text(builtin.applyValue(getRoleDefinitionResult -> getRoleDefinitionResult.id()))
* .build()).result()[StdFunctions.split(SplitArgs.builder()
* .separator("/")
* .text(builtin.applyValue(getRoleDefinitionResult -> getRoleDefinitionResult.id()))
* .build()).result().length() - 1])
* .build())
* .build());
*
* var exampleApplication = new Application("exampleApplication", ApplicationArgs.builder()
* .name("example-managedapplication")
* .location(example.location())
* .resourceGroupName(example.name())
* .kind("ServiceCatalog")
* .managedResourceGroupName("infrastructureGroup")
* .applicationDefinitionId(exampleDefinition.id())
* .parameterValues(example.location().applyValue(location -> serializeJson(
* jsonObject(
* jsonProperty("location", jsonObject(
* jsonProperty("value", location)
* )),
* jsonProperty("storageAccountNamePrefix", jsonObject(
* jsonProperty("value", "storeNamePrefix")
* )),
* jsonProperty("storageAccountType", jsonObject(
* jsonProperty("value", "Standard_LRS")
* ))
* ))))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* Managed Application can be imported using the `resource id`, e.g.
*
* ```sh
* $ pulumi import azure:managedapplication/application:Application example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.Solutions/applications/app1
* ```
*
*/
@ResourceType(type="azure:managedapplication/application:Application")
public class Application extends com.pulumi.resources.CustomResource {
/**
* The application definition ID to deploy.
*
*/
@Export(name="applicationDefinitionId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> applicationDefinitionId;
/**
* @return The application definition ID to deploy.
*
*/
public Output> applicationDefinitionId() {
return Codegen.optional(this.applicationDefinitionId);
}
/**
* The kind of the managed application to deploy. Possible values are `MarketPlace` and `ServiceCatalog`. Changing this forces a new resource to be created.
*
*/
@Export(name="kind", refs={String.class}, tree="[0]")
private Output kind;
/**
* @return The kind of the managed application to deploy. Possible values are `MarketPlace` and `ServiceCatalog`. Changing this forces a new resource to be created.
*
*/
public Output kind() {
return this.kind;
}
/**
* Specifies the supported Azure location where the resource exists. Changing this forces a new resource to be created.
*
*/
@Export(name="location", refs={String.class}, tree="[0]")
private Output location;
/**
* @return Specifies the supported Azure location where the resource exists. Changing this forces a new resource to be created.
*
*/
public Output location() {
return this.location;
}
/**
* The name of the target resource group where all the resources deployed by the managed application will reside. Changing this forces a new resource to be created.
*
*/
@Export(name="managedResourceGroupName", refs={String.class}, tree="[0]")
private Output managedResourceGroupName;
/**
* @return The name of the target resource group where all the resources deployed by the managed application will reside. Changing this forces a new resource to be created.
*
*/
public Output managedResourceGroupName() {
return this.managedResourceGroupName;
}
/**
* Specifies the name of the Managed Application. Changing this forces a new resource to be created.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return Specifies the name of the Managed Application. Changing this forces a new resource to be created.
*
*/
public Output name() {
return this.name;
}
/**
* The name and value pairs that define the managed application outputs.
*
*/
@Export(name="outputs", refs={Map.class,String.class}, tree="[0,1,1]")
private Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy