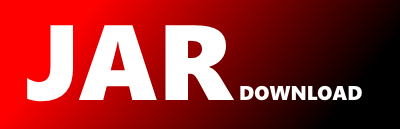
com.pulumi.azure.privatelink.outputs.EndpointPrivateServiceConnection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure Show documentation
Show all versions of azure Show documentation
A Pulumi package for creating and managing Microsoft Azure cloud resources, based on the Terraform azurerm provider. We recommend using the [Azure Native provider](https://github.com/pulumi/pulumi-azure-native) to provision Azure infrastructure. Azure Native provides complete coverage of Azure resources and same-day access to new resources and resource updates.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.privatelink.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class EndpointPrivateServiceConnection {
/**
* @return Does the Private Endpoint require Manual Approval from the remote resource owner? Changing this forces a new resource to be created.
*
* > **NOTE:** If you are trying to connect the Private Endpoint to a remote resource without having the correct RBAC permissions on the remote resource set this value to `true`.
*
*/
private Boolean isManualConnection;
/**
* @return Specifies the Name of the Private Service Connection. Changing this forces a new resource to be created.
*
*/
private String name;
/**
* @return The Service Alias of the Private Link Enabled Remote Resource which this Private Endpoint should be connected to. One of `private_connection_resource_id` or `private_connection_resource_alias` must be specified. Changing this forces a new resource to be created.
*
*/
private @Nullable String privateConnectionResourceAlias;
/**
* @return The ID of the Private Link Enabled Remote Resource which this Private Endpoint should be connected to. One of `private_connection_resource_id` or `private_connection_resource_alias` must be specified. Changing this forces a new resource to be created. For a web app or function app slot, the parent web app should be used in this field instead of a reference to the slot itself.
*
*/
private @Nullable String privateConnectionResourceId;
/**
* @return (Required) The static IP address set by this configuration. It is recommended to use the private IP address exported in the `private_service_connection` block to obtain the address associated with the private endpoint.
*
*/
private @Nullable String privateIpAddress;
/**
* @return A message passed to the owner of the remote resource when the private endpoint attempts to establish the connection to the remote resource. The provider allows a maximum request message length of `140` characters, however the request message maximum length is dependent on the service the private endpoint is connected to. Only valid if `is_manual_connection` is set to `true`.
*
* > **NOTE:** When connected to an SQL resource the `request_message` maximum length is `128`.
*
*/
private @Nullable String requestMessage;
/**
* @return A list of subresource names which the Private Endpoint is able to connect to. `subresource_names` corresponds to `group_id`. Possible values are detailed in the product [documentation](https://docs.microsoft.com/azure/private-link/private-endpoint-overview#private-link-resource) in the `Subresources` column. Changing this forces a new resource to be created.
*
* > **NOTE:** Some resource types (such as Storage Account) only support 1 subresource per private endpoint.
*
* > **NOTE:** For most Private Links one or more `subresource_names` will need to be specified, please see the linked documentation for details.
*
*/
private @Nullable List subresourceNames;
private EndpointPrivateServiceConnection() {}
/**
* @return Does the Private Endpoint require Manual Approval from the remote resource owner? Changing this forces a new resource to be created.
*
* > **NOTE:** If you are trying to connect the Private Endpoint to a remote resource without having the correct RBAC permissions on the remote resource set this value to `true`.
*
*/
public Boolean isManualConnection() {
return this.isManualConnection;
}
/**
* @return Specifies the Name of the Private Service Connection. Changing this forces a new resource to be created.
*
*/
public String name() {
return this.name;
}
/**
* @return The Service Alias of the Private Link Enabled Remote Resource which this Private Endpoint should be connected to. One of `private_connection_resource_id` or `private_connection_resource_alias` must be specified. Changing this forces a new resource to be created.
*
*/
public Optional privateConnectionResourceAlias() {
return Optional.ofNullable(this.privateConnectionResourceAlias);
}
/**
* @return The ID of the Private Link Enabled Remote Resource which this Private Endpoint should be connected to. One of `private_connection_resource_id` or `private_connection_resource_alias` must be specified. Changing this forces a new resource to be created. For a web app or function app slot, the parent web app should be used in this field instead of a reference to the slot itself.
*
*/
public Optional privateConnectionResourceId() {
return Optional.ofNullable(this.privateConnectionResourceId);
}
/**
* @return (Required) The static IP address set by this configuration. It is recommended to use the private IP address exported in the `private_service_connection` block to obtain the address associated with the private endpoint.
*
*/
public Optional privateIpAddress() {
return Optional.ofNullable(this.privateIpAddress);
}
/**
* @return A message passed to the owner of the remote resource when the private endpoint attempts to establish the connection to the remote resource. The provider allows a maximum request message length of `140` characters, however the request message maximum length is dependent on the service the private endpoint is connected to. Only valid if `is_manual_connection` is set to `true`.
*
* > **NOTE:** When connected to an SQL resource the `request_message` maximum length is `128`.
*
*/
public Optional requestMessage() {
return Optional.ofNullable(this.requestMessage);
}
/**
* @return A list of subresource names which the Private Endpoint is able to connect to. `subresource_names` corresponds to `group_id`. Possible values are detailed in the product [documentation](https://docs.microsoft.com/azure/private-link/private-endpoint-overview#private-link-resource) in the `Subresources` column. Changing this forces a new resource to be created.
*
* > **NOTE:** Some resource types (such as Storage Account) only support 1 subresource per private endpoint.
*
* > **NOTE:** For most Private Links one or more `subresource_names` will need to be specified, please see the linked documentation for details.
*
*/
public List subresourceNames() {
return this.subresourceNames == null ? List.of() : this.subresourceNames;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(EndpointPrivateServiceConnection defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private Boolean isManualConnection;
private String name;
private @Nullable String privateConnectionResourceAlias;
private @Nullable String privateConnectionResourceId;
private @Nullable String privateIpAddress;
private @Nullable String requestMessage;
private @Nullable List subresourceNames;
public Builder() {}
public Builder(EndpointPrivateServiceConnection defaults) {
Objects.requireNonNull(defaults);
this.isManualConnection = defaults.isManualConnection;
this.name = defaults.name;
this.privateConnectionResourceAlias = defaults.privateConnectionResourceAlias;
this.privateConnectionResourceId = defaults.privateConnectionResourceId;
this.privateIpAddress = defaults.privateIpAddress;
this.requestMessage = defaults.requestMessage;
this.subresourceNames = defaults.subresourceNames;
}
@CustomType.Setter
public Builder isManualConnection(Boolean isManualConnection) {
if (isManualConnection == null) {
throw new MissingRequiredPropertyException("EndpointPrivateServiceConnection", "isManualConnection");
}
this.isManualConnection = isManualConnection;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("EndpointPrivateServiceConnection", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder privateConnectionResourceAlias(@Nullable String privateConnectionResourceAlias) {
this.privateConnectionResourceAlias = privateConnectionResourceAlias;
return this;
}
@CustomType.Setter
public Builder privateConnectionResourceId(@Nullable String privateConnectionResourceId) {
this.privateConnectionResourceId = privateConnectionResourceId;
return this;
}
@CustomType.Setter
public Builder privateIpAddress(@Nullable String privateIpAddress) {
this.privateIpAddress = privateIpAddress;
return this;
}
@CustomType.Setter
public Builder requestMessage(@Nullable String requestMessage) {
this.requestMessage = requestMessage;
return this;
}
@CustomType.Setter
public Builder subresourceNames(@Nullable List subresourceNames) {
this.subresourceNames = subresourceNames;
return this;
}
public Builder subresourceNames(String... subresourceNames) {
return subresourceNames(List.of(subresourceNames));
}
public EndpointPrivateServiceConnection build() {
final var _resultValue = new EndpointPrivateServiceConnection();
_resultValue.isManualConnection = isManualConnection;
_resultValue.name = name;
_resultValue.privateConnectionResourceAlias = privateConnectionResourceAlias;
_resultValue.privateConnectionResourceId = privateConnectionResourceId;
_resultValue.privateIpAddress = privateIpAddress;
_resultValue.requestMessage = requestMessage;
_resultValue.subresourceNames = subresourceNames;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy