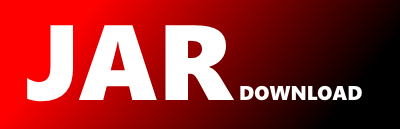
com.pulumi.azure.redhatopenshift.inputs.ClusterWorkerProfileArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure Show documentation
Show all versions of azure Show documentation
A Pulumi package for creating and managing Microsoft Azure cloud resources, based on the Terraform azurerm provider. We recommend using the [Azure Native provider](https://github.com/pulumi/pulumi-azure-native) to provision Azure infrastructure. Azure Native provides complete coverage of Azure resources and same-day access to new resources and resource updates.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.redhatopenshift.inputs;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class ClusterWorkerProfileArgs extends com.pulumi.resources.ResourceArgs {
public static final ClusterWorkerProfileArgs Empty = new ClusterWorkerProfileArgs();
/**
* The resource ID of an associated disk encryption set. Changing this forces a new resource to be created.
*
*/
@Import(name="diskEncryptionSetId")
private @Nullable Output diskEncryptionSetId;
/**
* @return The resource ID of an associated disk encryption set. Changing this forces a new resource to be created.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy