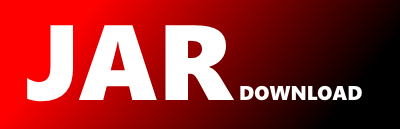
com.pulumi.azure.servicebus.outputs.GetNamespaceDisasterRecoveryConfigResult Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of azure Show documentation
Show all versions of azure Show documentation
A Pulumi package for creating and managing Microsoft Azure cloud resources, based on the Terraform azurerm provider. We recommend using the [Azure Native provider](https://github.com/pulumi/pulumi-azure-native) to provision Azure infrastructure. Azure Native provides complete coverage of Azure resources and same-day access to new resources and resource updates.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.azure.servicebus.outputs;
import com.pulumi.core.annotations.CustomType;
import com.pulumi.exceptions.MissingRequiredPropertyException;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
@CustomType
public final class GetNamespaceDisasterRecoveryConfigResult {
private @Nullable String aliasAuthorizationRuleId;
private String defaultPrimaryKey;
private String defaultSecondaryKey;
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
private String id;
private String name;
private @Nullable String namespaceId;
/**
* @deprecated
* `namespace_name` will be removed in favour of the property `namespace_id` in version 4.0 of the AzureRM Provider.
*
*/
@Deprecated /* `namespace_name` will be removed in favour of the property `namespace_id` in version 4.0 of the AzureRM Provider. */
private @Nullable String namespaceName;
private String partnerNamespaceId;
private String primaryConnectionStringAlias;
/**
* @deprecated
* `resource_group_name` will be removed in favour of the property `namespace_id` in version 4.0 of the AzureRM Provider.
*
*/
@Deprecated /* `resource_group_name` will be removed in favour of the property `namespace_id` in version 4.0 of the AzureRM Provider. */
private @Nullable String resourceGroupName;
private String secondaryConnectionStringAlias;
private GetNamespaceDisasterRecoveryConfigResult() {}
public Optional aliasAuthorizationRuleId() {
return Optional.ofNullable(this.aliasAuthorizationRuleId);
}
public String defaultPrimaryKey() {
return this.defaultPrimaryKey;
}
public String defaultSecondaryKey() {
return this.defaultSecondaryKey;
}
/**
* @return The provider-assigned unique ID for this managed resource.
*
*/
public String id() {
return this.id;
}
public String name() {
return this.name;
}
public Optional namespaceId() {
return Optional.ofNullable(this.namespaceId);
}
/**
* @deprecated
* `namespace_name` will be removed in favour of the property `namespace_id` in version 4.0 of the AzureRM Provider.
*
*/
@Deprecated /* `namespace_name` will be removed in favour of the property `namespace_id` in version 4.0 of the AzureRM Provider. */
public Optional namespaceName() {
return Optional.ofNullable(this.namespaceName);
}
public String partnerNamespaceId() {
return this.partnerNamespaceId;
}
public String primaryConnectionStringAlias() {
return this.primaryConnectionStringAlias;
}
/**
* @deprecated
* `resource_group_name` will be removed in favour of the property `namespace_id` in version 4.0 of the AzureRM Provider.
*
*/
@Deprecated /* `resource_group_name` will be removed in favour of the property `namespace_id` in version 4.0 of the AzureRM Provider. */
public Optional resourceGroupName() {
return Optional.ofNullable(this.resourceGroupName);
}
public String secondaryConnectionStringAlias() {
return this.secondaryConnectionStringAlias;
}
public static Builder builder() {
return new Builder();
}
public static Builder builder(GetNamespaceDisasterRecoveryConfigResult defaults) {
return new Builder(defaults);
}
@CustomType.Builder
public static final class Builder {
private @Nullable String aliasAuthorizationRuleId;
private String defaultPrimaryKey;
private String defaultSecondaryKey;
private String id;
private String name;
private @Nullable String namespaceId;
private @Nullable String namespaceName;
private String partnerNamespaceId;
private String primaryConnectionStringAlias;
private @Nullable String resourceGroupName;
private String secondaryConnectionStringAlias;
public Builder() {}
public Builder(GetNamespaceDisasterRecoveryConfigResult defaults) {
Objects.requireNonNull(defaults);
this.aliasAuthorizationRuleId = defaults.aliasAuthorizationRuleId;
this.defaultPrimaryKey = defaults.defaultPrimaryKey;
this.defaultSecondaryKey = defaults.defaultSecondaryKey;
this.id = defaults.id;
this.name = defaults.name;
this.namespaceId = defaults.namespaceId;
this.namespaceName = defaults.namespaceName;
this.partnerNamespaceId = defaults.partnerNamespaceId;
this.primaryConnectionStringAlias = defaults.primaryConnectionStringAlias;
this.resourceGroupName = defaults.resourceGroupName;
this.secondaryConnectionStringAlias = defaults.secondaryConnectionStringAlias;
}
@CustomType.Setter
public Builder aliasAuthorizationRuleId(@Nullable String aliasAuthorizationRuleId) {
this.aliasAuthorizationRuleId = aliasAuthorizationRuleId;
return this;
}
@CustomType.Setter
public Builder defaultPrimaryKey(String defaultPrimaryKey) {
if (defaultPrimaryKey == null) {
throw new MissingRequiredPropertyException("GetNamespaceDisasterRecoveryConfigResult", "defaultPrimaryKey");
}
this.defaultPrimaryKey = defaultPrimaryKey;
return this;
}
@CustomType.Setter
public Builder defaultSecondaryKey(String defaultSecondaryKey) {
if (defaultSecondaryKey == null) {
throw new MissingRequiredPropertyException("GetNamespaceDisasterRecoveryConfigResult", "defaultSecondaryKey");
}
this.defaultSecondaryKey = defaultSecondaryKey;
return this;
}
@CustomType.Setter
public Builder id(String id) {
if (id == null) {
throw new MissingRequiredPropertyException("GetNamespaceDisasterRecoveryConfigResult", "id");
}
this.id = id;
return this;
}
@CustomType.Setter
public Builder name(String name) {
if (name == null) {
throw new MissingRequiredPropertyException("GetNamespaceDisasterRecoveryConfigResult", "name");
}
this.name = name;
return this;
}
@CustomType.Setter
public Builder namespaceId(@Nullable String namespaceId) {
this.namespaceId = namespaceId;
return this;
}
@CustomType.Setter
public Builder namespaceName(@Nullable String namespaceName) {
this.namespaceName = namespaceName;
return this;
}
@CustomType.Setter
public Builder partnerNamespaceId(String partnerNamespaceId) {
if (partnerNamespaceId == null) {
throw new MissingRequiredPropertyException("GetNamespaceDisasterRecoveryConfigResult", "partnerNamespaceId");
}
this.partnerNamespaceId = partnerNamespaceId;
return this;
}
@CustomType.Setter
public Builder primaryConnectionStringAlias(String primaryConnectionStringAlias) {
if (primaryConnectionStringAlias == null) {
throw new MissingRequiredPropertyException("GetNamespaceDisasterRecoveryConfigResult", "primaryConnectionStringAlias");
}
this.primaryConnectionStringAlias = primaryConnectionStringAlias;
return this;
}
@CustomType.Setter
public Builder resourceGroupName(@Nullable String resourceGroupName) {
this.resourceGroupName = resourceGroupName;
return this;
}
@CustomType.Setter
public Builder secondaryConnectionStringAlias(String secondaryConnectionStringAlias) {
if (secondaryConnectionStringAlias == null) {
throw new MissingRequiredPropertyException("GetNamespaceDisasterRecoveryConfigResult", "secondaryConnectionStringAlias");
}
this.secondaryConnectionStringAlias = secondaryConnectionStringAlias;
return this;
}
public GetNamespaceDisasterRecoveryConfigResult build() {
final var _resultValue = new GetNamespaceDisasterRecoveryConfigResult();
_resultValue.aliasAuthorizationRuleId = aliasAuthorizationRuleId;
_resultValue.defaultPrimaryKey = defaultPrimaryKey;
_resultValue.defaultSecondaryKey = defaultSecondaryKey;
_resultValue.id = id;
_resultValue.name = name;
_resultValue.namespaceId = namespaceId;
_resultValue.namespaceName = namespaceName;
_resultValue.partnerNamespaceId = partnerNamespaceId;
_resultValue.primaryConnectionStringAlias = primaryConnectionStringAlias;
_resultValue.resourceGroupName = resourceGroupName;
_resultValue.secondaryConnectionStringAlias = secondaryConnectionStringAlias;
return _resultValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy