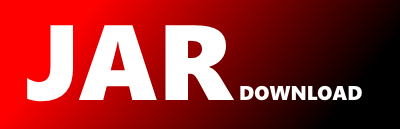
com.pulumi.cloudngfwaws.Ngfw Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cloudngfwaws Show documentation
Show all versions of cloudngfwaws Show documentation
A Pulumi package for creating and managing Cloud NGFW for AWS resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.cloudngfwaws;
import com.pulumi.cloudngfwaws.NgfwArgs;
import com.pulumi.cloudngfwaws.Utilities;
import com.pulumi.cloudngfwaws.inputs.NgfwState;
import com.pulumi.cloudngfwaws.outputs.NgfwStatus;
import com.pulumi.cloudngfwaws.outputs.NgfwSubnetMapping;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.Boolean;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ## Example Usage
*
* <!--Start PulumiCodeChooser -->
*
* {@code
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.cloudngfwaws.CommitRulestack;
* import com.pulumi.cloudngfwaws.CommitRulestackArgs;
* import com.pulumi.aws.vpc;
* import com.pulumi.aws.VpcArgs;
* import com.pulumi.aws.subnet;
* import com.pulumi.aws.SubnetArgs;
* import com.pulumi.cloudngfwaws.Ngfw;
* import com.pulumi.cloudngfwaws.NgfwArgs;
* import com.pulumi.cloudngfwaws.inputs.NgfwSubnetMappingArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var rs = new CommitRulestack("rs", CommitRulestackArgs.builder()
* .rulestack("my-rulestack")
* .build());
*
* var exampleVpc = new Vpc("exampleVpc", VpcArgs.builder()
* .cidrBlock("172.16.0.0/16")
* .tags(%!v(PANIC=Format method: runtime error: invalid memory address or nil pointer dereference))
* .build());
*
* var subnet1 = new Subnet("subnet1", SubnetArgs.builder()
* .vpcId(myVpc.id())
* .cidrBlock("172.16.10.0/24")
* .availabilityZone("us-west-2a")
* .tags(%!v(PANIC=Format method: runtime error: invalid memory address or nil pointer dereference))
* .build());
*
* var subnet2 = new Subnet("subnet2", SubnetArgs.builder()
* .vpcId(myVpc.id())
* .cidrBlock("172.16.20.0/24")
* .availabilityZone("us-west-2b")
* .tags(%!v(PANIC=Format method: runtime error: invalid memory address or nil pointer dereference))
* .build());
*
* var example = new Ngfw("example", NgfwArgs.builder()
* .name("example-instance")
* .vpcId(exampleVpc.id())
* .accountId("12345678")
* .description("Example description")
* .linkId("Link-81e80ccc-357a-4e4e-8325-1ed1d830cba5")
* .endpointMode("ServiceManaged")
* .subnetMappings(
* NgfwSubnetMappingArgs.builder()
* .subnetId(subnet1.id())
* .build(),
* NgfwSubnetMappingArgs.builder()
* .subnetId(subnet2.id())
* .build())
* .rulestack(rs.rulestack())
* .tags(Map.of("Foo", "bar"))
* .build());
*
* }
* }
* }
*
* <!--End PulumiCodeChooser -->
*
* ## Import
*
* import name is <account_id>:<name>
*
* ```sh
* $ pulumi import cloudngfwaws:index/ngfw:Ngfw example 12345678:example-instance
* ```
*
*/
@ResourceType(type="cloudngfwaws:index/ngfw:Ngfw")
public class Ngfw extends com.pulumi.resources.CustomResource {
/**
* The account ID. This field is mandatory if using multiple accounts.
*
*/
@Export(name="accountId", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> accountId;
/**
* @return The account ID. This field is mandatory if using multiple accounts.
*
*/
public Output> accountId() {
return Codegen.optional(this.accountId);
}
/**
* App-ID version number.
*
*/
@Export(name="appIdVersion", refs={String.class}, tree="[0]")
private Output appIdVersion;
/**
* @return App-ID version number.
*
*/
public Output appIdVersion() {
return this.appIdVersion;
}
/**
* Automatic App-ID upgrade version number. Defaults to `true`.
*
*/
@Export(name="automaticUpgradeAppIdVersion", refs={Boolean.class}, tree="[0]")
private Output* @Nullable */ Boolean> automaticUpgradeAppIdVersion;
/**
* @return Automatic App-ID upgrade version number. Defaults to `true`.
*
*/
public Output> automaticUpgradeAppIdVersion() {
return Codegen.optional(this.automaticUpgradeAppIdVersion);
}
/**
* The description.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return The description.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* Set endpoint mode from the following options. Valid values are `ServiceManaged` or `CustomerManaged`.
*
*/
@Export(name="endpointMode", refs={String.class}, tree="[0]")
private Output endpointMode;
/**
* @return Set endpoint mode from the following options. Valid values are `ServiceManaged` or `CustomerManaged`.
*
*/
public Output endpointMode() {
return this.endpointMode;
}
/**
* The endpoint service name.
*
*/
@Export(name="endpointServiceName", refs={String.class}, tree="[0]")
private Output endpointServiceName;
/**
* @return The endpoint service name.
*
*/
public Output endpointServiceName() {
return this.endpointServiceName;
}
/**
* The Id of the NGFW.
*
*/
@Export(name="firewallId", refs={String.class}, tree="[0]")
private Output firewallId;
/**
* @return The Id of the NGFW.
*
*/
public Output firewallId() {
return this.firewallId;
}
/**
* The global rulestack for this NGFW.
*
*/
@Export(name="globalRulestack", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> globalRulestack;
/**
* @return The global rulestack for this NGFW.
*
*/
public Output> globalRulestack() {
return Codegen.optional(this.globalRulestack);
}
/**
* A unique identifier for establishing and managing the link between the Cloud NGFW and other AWS resources.
*
*/
@Export(name="linkId", refs={String.class}, tree="[0]")
private Output linkId;
/**
* @return A unique identifier for establishing and managing the link between the Cloud NGFW and other AWS resources.
*
*/
public Output linkId() {
return this.linkId;
}
/**
* The link status.
*
*/
@Export(name="linkStatus", refs={String.class}, tree="[0]")
private Output linkStatus;
/**
* @return The link status.
*
*/
public Output linkStatus() {
return this.linkStatus;
}
/**
* Share NGFW with Multiple VPCs. This feature can be enabled only if the endpoint_mode is CustomerManaged.
*
*/
@Export(name="multiVpc", refs={Boolean.class}, tree="[0]")
private Output multiVpc;
/**
* @return Share NGFW with Multiple VPCs. This feature can be enabled only if the endpoint_mode is CustomerManaged.
*
*/
public Output multiVpc() {
return this.multiVpc;
}
/**
* The NGFW name.
*
*/
@Export(name="name", refs={String.class}, tree="[0]")
private Output name;
/**
* @return The NGFW name.
*
*/
public Output name() {
return this.name;
}
/**
* The rulestack for this NGFW.
*
*/
@Export(name="rulestack", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> rulestack;
/**
* @return The rulestack for this NGFW.
*
*/
public Output> rulestack() {
return Codegen.optional(this.rulestack);
}
@Export(name="statuses", refs={List.class,NgfwStatus.class}, tree="[0,1]")
private Output> statuses;
public Output> statuses() {
return this.statuses;
}
/**
* Subnet mappings.
*
*/
@Export(name="subnetMappings", refs={List.class,NgfwSubnetMapping.class}, tree="[0,1]")
private Output> subnetMappings;
/**
* @return Subnet mappings.
*
*/
public Output> subnetMappings() {
return this.subnetMappings;
}
/**
* The tags.
*
*/
@Export(name="tags", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> tags;
/**
* @return The tags.
*
*/
public Output>> tags() {
return Codegen.optional(this.tags);
}
/**
* The update token.
*
*/
@Export(name="updateToken", refs={String.class}, tree="[0]")
private Output updateToken;
/**
* @return The update token.
*
*/
public Output updateToken() {
return this.updateToken;
}
/**
* The vpc id.
*
*/
@Export(name="vpcId", refs={String.class}, tree="[0]")
private Output vpcId;
/**
* @return The vpc id.
*
*/
public Output vpcId() {
return this.vpcId;
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Ngfw(java.lang.String name) {
this(name, NgfwArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Ngfw(java.lang.String name, NgfwArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Ngfw(java.lang.String name, NgfwArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("cloudngfwaws:index/ngfw:Ngfw", name, makeArgs(args, options), makeResourceOptions(options, Codegen.empty()), false);
}
private Ngfw(java.lang.String name, Output id, @Nullable NgfwState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("cloudngfwaws:index/ngfw:Ngfw", name, state, makeResourceOptions(options, id), false);
}
private static NgfwArgs makeArgs(NgfwArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
if (options != null && options.getUrn().isPresent()) {
return null;
}
return args == null ? NgfwArgs.Empty : args;
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Ngfw get(java.lang.String name, Output id, @Nullable NgfwState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Ngfw(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy