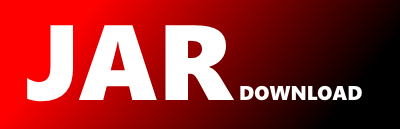
com.pulumi.consul.AutopilotConfigArgs Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of consul Show documentation
Show all versions of consul Show documentation
A Pulumi package for creating and managing consul resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.consul;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Import;
import java.lang.Boolean;
import java.lang.Integer;
import java.lang.String;
import java.util.Objects;
import java.util.Optional;
import javax.annotation.Nullable;
public final class AutopilotConfigArgs extends com.pulumi.resources.ResourceArgs {
public static final AutopilotConfigArgs Empty = new AutopilotConfigArgs();
/**
* Whether to remove failing servers when a
* replacement comes online. Defaults to true.
*
*/
@Import(name="cleanupDeadServers")
private @Nullable Output cleanupDeadServers;
/**
* @return Whether to remove failing servers when a
* replacement comes online. Defaults to true.
*
*/
public Optional
© 2015 - 2025 Weber Informatics LLC | Privacy Policy