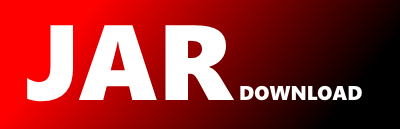
com.pulumi.consul.Intention Maven / Gradle / Ivy
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.consul;
import com.pulumi.consul.IntentionArgs;
import com.pulumi.consul.Utilities;
import com.pulumi.consul.inputs.IntentionState;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* [Intentions](https://www.consul.io/docs/connect/intentions.html) are used to define
* rules for which services may connect to one another when using [Consul Connect](https://www.consul.io/docs/connect/index.html).
*
* > **NOTE:** This resource is appropriate for managing legacy intentions in
* Consul version 1.8 and earlier. As of Consul 1.9, intentions should be managed
* using the [`service-intentions`](https://www.consul.io/docs/connect/intentions)
* configuration entry. It is recommended to migrate from the `consul.Intention`
* resource to `consul.ConfigEntry` when running Consul 1.9 and later.
*
* It is appropriate to either reference existing services, or specify non-existent services
* that will be created in the future when creating intentions. This resource can be used
* in conjunction with the `consul.Service` datasource when referencing services
* registered on nodes that have a running Consul agent.
*
* ## Example Usage
*
* Create a simplest intention with static service names:
* ```java
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.consul.Intention;
* import com.pulumi.consul.IntentionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var database = new Intention("database", IntentionArgs.builder()
* .action("allow")
* .destinationName("db")
* .sourceName("api")
* .build());
*
* }
* }
* ```
*
* Referencing a known service via a datasource:
* ```java
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.consul.Intention;
* import com.pulumi.consul.IntentionArgs;
* import com.pulumi.consul.ConsulFunctions;
* import com.pulumi.consul.inputs.GetServiceArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var database = new Intention("database", IntentionArgs.builder()
* .action("allow")
* .destinationName(consul_service.pg().name())
* .sourceName("api")
* .build());
*
* final var pg = ConsulFunctions.getService(GetServiceArgs.builder()
* .name("postgresql")
* .build());
*
* }
* }
* ```
*
* ## Import
*
* `consul_intention` can be imported:
*
* ```sh
* $ pulumi import consul:index/intention:Intention database 657a57d6-0d56-57e2-31cb-e9f1ed3c18dd
* ```
*
*/
@ResourceType(type="consul:index/intention:Intention")
public class Intention extends com.pulumi.resources.CustomResource {
/**
* The intention action. Must be one of `allow` or `deny`.
*
*/
@Export(name="action", refs={String.class}, tree="[0]")
private Output action;
/**
* @return The intention action. Must be one of `allow` or `deny`.
*
*/
public Output action() {
return this.action;
}
/**
* The datacenter to use. This overrides the
* agent's default datacenter and the datacenter in the provider setup.
*
*/
@Export(name="datacenter", refs={String.class}, tree="[0]")
private Output datacenter;
/**
* @return The datacenter to use. This overrides the
* agent's default datacenter and the datacenter in the provider setup.
*
*/
public Output datacenter() {
return this.datacenter;
}
/**
* Optional description that can be used by Consul
* tooling, but is not used internally.
*
*/
@Export(name="description", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> description;
/**
* @return Optional description that can be used by Consul
* tooling, but is not used internally.
*
*/
public Output> description() {
return Codegen.optional(this.description);
}
/**
* The name of the destination service for the intention. This
* service does not have to exist.
*
*/
@Export(name="destinationName", refs={String.class}, tree="[0]")
private Output destinationName;
/**
* @return The name of the destination service for the intention. This
* service does not have to exist.
*
*/
public Output destinationName() {
return this.destinationName;
}
/**
* The destination
* namespace of the intention.
*
*/
@Export(name="destinationNamespace", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> destinationNamespace;
/**
* @return The destination
* namespace of the intention.
*
*/
public Output> destinationNamespace() {
return Codegen.optional(this.destinationNamespace);
}
/**
* Key/value pairs that are opaque to Consul and are associated
* with the intention.
*
*/
@Export(name="meta", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> meta;
/**
* @return Key/value pairs that are opaque to Consul and are associated
* with the intention.
*
*/
public Output>> meta() {
return Codegen.optional(this.meta);
}
/**
* The name of the source service for the intention. This
* service does not have to exist.
*
*/
@Export(name="sourceName", refs={String.class}, tree="[0]")
private Output sourceName;
/**
* @return The name of the source service for the intention. This
* service does not have to exist.
*
*/
public Output sourceName() {
return this.sourceName;
}
/**
* The source namespace of the
* intention.
*
*/
@Export(name="sourceNamespace", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> sourceNamespace;
/**
* @return The source namespace of the
* intention.
*
*/
public Output> sourceNamespace() {
return Codegen.optional(this.sourceNamespace);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public Intention(String name) {
this(name, IntentionArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public Intention(String name, IntentionArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public Intention(String name, IntentionArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("consul:index/intention:Intention", name, args == null ? IntentionArgs.Empty : args, makeResourceOptions(options, Codegen.empty()));
}
private Intention(String name, Output id, @Nullable IntentionState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("consul:index/intention:Intention", name, state, makeResourceOptions(options, id));
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static Intention get(String name, Output id, @Nullable IntentionState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new Intention(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy