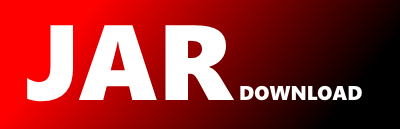
com.pulumi.consul.KeyPrefix Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of consul Show documentation
Show all versions of consul Show documentation
A Pulumi package for creating and managing consul resources.
// *** WARNING: this file was generated by pulumi-java-gen. ***
// *** Do not edit by hand unless you're certain you know what you are doing! ***
package com.pulumi.consul;
import com.pulumi.consul.KeyPrefixArgs;
import com.pulumi.consul.Utilities;
import com.pulumi.consul.inputs.KeyPrefixState;
import com.pulumi.consul.outputs.KeyPrefixSubkeyCollection;
import com.pulumi.core.Output;
import com.pulumi.core.annotations.Export;
import com.pulumi.core.annotations.ResourceType;
import com.pulumi.core.internal.Codegen;
import java.lang.String;
import java.util.List;
import java.util.Map;
import java.util.Optional;
import javax.annotation.Nullable;
/**
* ## Example Usage
* ```java
* package generated_program;
*
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.consul.KeyPrefix;
* import com.pulumi.consul.KeyPrefixArgs;
* import com.pulumi.consul.inputs.KeyPrefixSubkeyCollectionArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
*
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
*
* public static void stack(Context ctx) {
* var myappConfig = new KeyPrefix("myappConfig", KeyPrefixArgs.builder()
* .datacenter("nyc1")
* .pathPrefix("myapp/config/")
* .subkeyCollection(KeyPrefixSubkeyCollectionArgs.builder()
* .flags(2)
* .path("database/password")
* .value(aws_db_instance.app().password())
* .build())
* .subkeys(Map.ofEntries(
* Map.entry("database/hostname", aws_db_instance.app().address()),
* Map.entry("database/name", aws_db_instance.app().name()),
* Map.entry("database/port", aws_db_instance.app().port()),
* Map.entry("database/username", aws_db_instance.app().username()),
* Map.entry("elb_cname", aws_elb.app().dns_name()),
* Map.entry("s3_bucket_name", aws_s3_bucket.app().bucket())
* ))
* .token("abcd")
* .build());
*
* }
* }
* ```
*
* ## Import
*
* `consul_key_prefix` can be imported. This is useful when the path already exists and you know all keys in path should be managed by Terraform.
*
* ```sh
* $ pulumi import consul:index/keyPrefix:KeyPrefix myapp_config myapp/config/
* ```
*
*/
@ResourceType(type="consul:index/keyPrefix:KeyPrefix")
public class KeyPrefix extends com.pulumi.resources.CustomResource {
/**
* The datacenter to use. This overrides the
* agent's default datacenter and the datacenter in the provider setup.
*
*/
@Export(name="datacenter", refs={String.class}, tree="[0]")
private Output datacenter;
/**
* @return The datacenter to use. This overrides the
* agent's default datacenter and the datacenter in the provider setup.
*
*/
public Output datacenter() {
return this.datacenter;
}
/**
* The namespace to create the keys within.
*
*/
@Export(name="namespace", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> namespace;
/**
* @return The namespace to create the keys within.
*
*/
public Output> namespace() {
return Codegen.optional(this.namespace);
}
/**
* The admin partition to create the keys within.
*
*/
@Export(name="partition", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> partition;
/**
* @return The admin partition to create the keys within.
*
*/
public Output> partition() {
return Codegen.optional(this.partition);
}
/**
* Specifies the common prefix shared by all keys
* that will be managed by this resource instance. In most cases this will
* end with a slash, to manage a "folder" of keys.
*
*/
@Export(name="pathPrefix", refs={String.class}, tree="[0]")
private Output pathPrefix;
/**
* @return Specifies the common prefix shared by all keys
* that will be managed by this resource instance. In most cases this will
* end with a slash, to manage a "folder" of keys.
*
*/
public Output pathPrefix() {
return this.pathPrefix;
}
/**
* A subkey to add. Supported values documented below.
* Multiple blocks supported.
*
*/
@Export(name="subkeyCollection", refs={List.class,KeyPrefixSubkeyCollection.class}, tree="[0,1]")
private Output* @Nullable */ List> subkeyCollection;
/**
* @return A subkey to add. Supported values documented below.
* Multiple blocks supported.
*
*/
public Output>> subkeyCollection() {
return Codegen.optional(this.subkeyCollection);
}
/**
* A mapping from subkey name (which will be appended
* to the given `path_prefix`) to the value that should be stored at that key.
* Use slashes, as shown in the above example, to create "sub-folders" under
* the given path prefix.
*
*/
@Export(name="subkeys", refs={Map.class,String.class}, tree="[0,1,1]")
private Output* @Nullable */ Map> subkeys;
/**
* @return A mapping from subkey name (which will be appended
* to the given `path_prefix`) to the value that should be stored at that key.
* Use slashes, as shown in the above example, to create "sub-folders" under
* the given path prefix.
*
*/
public Output>> subkeys() {
return Codegen.optional(this.subkeys);
}
/**
* The ACL token to use. This overrides the
* token that the agent provides by default.
*
* @deprecated
* The token argument has been deprecated and will be removed in a future release.
* Please use the token argument in the provider configuration
*
*/
@Deprecated /* The token argument has been deprecated and will be removed in a future release.
Please use the token argument in the provider configuration */
@Export(name="token", refs={String.class}, tree="[0]")
private Output* @Nullable */ String> token;
/**
* @return The ACL token to use. This overrides the
* token that the agent provides by default.
*
*/
public Output> token() {
return Codegen.optional(this.token);
}
/**
*
* @param name The _unique_ name of the resulting resource.
*/
public KeyPrefix(String name) {
this(name, KeyPrefixArgs.Empty);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
*/
public KeyPrefix(String name, KeyPrefixArgs args) {
this(name, args, null);
}
/**
*
* @param name The _unique_ name of the resulting resource.
* @param args The arguments to use to populate this resource's properties.
* @param options A bag of options that control this resource's behavior.
*/
public KeyPrefix(String name, KeyPrefixArgs args, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("consul:index/keyPrefix:KeyPrefix", name, args == null ? KeyPrefixArgs.Empty : args, makeResourceOptions(options, Codegen.empty()));
}
private KeyPrefix(String name, Output id, @Nullable KeyPrefixState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
super("consul:index/keyPrefix:KeyPrefix", name, state, makeResourceOptions(options, id));
}
private static com.pulumi.resources.CustomResourceOptions makeResourceOptions(@Nullable com.pulumi.resources.CustomResourceOptions options, @Nullable Output id) {
var defaultOptions = com.pulumi.resources.CustomResourceOptions.builder()
.version(Utilities.getVersion())
.additionalSecretOutputs(List.of(
"token"
))
.build();
return com.pulumi.resources.CustomResourceOptions.merge(defaultOptions, options, id);
}
/**
* Get an existing Host resource's state with the given name, ID, and optional extra
* properties used to qualify the lookup.
*
* @param name The _unique_ name of the resulting resource.
* @param id The _unique_ provider ID of the resource to lookup.
* @param state
* @param options Optional settings to control the behavior of the CustomResource.
*/
public static KeyPrefix get(String name, Output id, @Nullable KeyPrefixState state, @Nullable com.pulumi.resources.CustomResourceOptions options) {
return new KeyPrefix(name, id, state, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy